CHALLENGE ACTIVITY 3.15.1: String with digit Set hasDigit to true if the 3-character passCode contains a digit Learn how our autograder works 1 #include 2 #include 3 #include 4 #include 5 6 int main(void) { 7 8 9 10 11 12 13 14 15 16 17 bool hasDigit; char passCode [50]; hasDigit false; scanf("%s", passCode); y Your solution goes here / if (hasDigit) { printf("Has a digit.\n");
CHALLENGE ACTIVITY 3.15.1: String with digit Set hasDigit to true if the 3-character passCode contains a digit Learn how our autograder works 1 #include 2 #include 3 #include 4 #include 5 6 int main(void) { 7 8 9 10 11 12 13 14 15 16 17 bool hasDigit; char passCode [50]; hasDigit false; scanf("%s", passCode); y Your solution goes here / if (hasDigit) { printf("Has a digit.\n");
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
100%
Need help, C
![### Section 3.15: Character Operations
#### Challenge Activity: 3.15.1 String with Digit
**Objective:**
Set `hasDigit` to true if the 3-character `passCode` contains a digit.
**Instructions:**
Complete the given C program to meet the specified objective. You need to write the logic that will determine if the `passCode` contains any digit character.
Here is the initial code provided for you:
```c
#include <stdio.h>
#include <string.h>
#include <stdbool.h>
#include <ctype.h>
int main(void) {
bool hasDigit;
char passCode[50];
hasDigit = false;
scanf("%s", passCode);
/* Your solution goes here */
if (hasDigit) {
printf("Has a digit.\n");
}
return 0;
}
```
**Steps to Follow:**
1. **Input Reading:**
The input `passCode` is read using `scanf`.
2. **Initialize `hasDigit`:**
Set the `hasDigit` variable to `false`.
3. **Logic Insertion:**
Insert logic to traverse the characters of `passCode` and set `hasDigit` to `true` if any character is a digit.
4. **Output:**
Print "Has a digit." if `hasDigit` is `true`.
- **Running the Program:**
Press the "Run" button to execute the program and verify the output.
#### Example: Checking for Digit
Consider the following `passCode` input: `abc123`
- The logic should properly identify that it contains digits.
#### Challenge Activity 3.15.2: Alphabetic Replace
**Objective:**
Replace any alphabetic character with `_` in a 2-character string `passCode`.
**Instructions:**
1. **Initialize:**
Read the `passCode` consisting of exactly 2 characters.
2. **Implementation:**
Use conditional statements to check each character of `passCode` using `isalpha()`. Replace alphabetic characters accordingly.
Example:
- Input: `9a`
- Output: `9_`
Please note that these challenge activities are crucial for understanding character operations in C, which is an essential skill in programming.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F3e035166-c990-4dd0-a7c5-b2c139b07c7d%2F7ae17dbd-fd43-4d44-ab58-92c1e17b838b%2Fdib3pzv_processed.png&w=3840&q=75)
Transcribed Image Text:### Section 3.15: Character Operations
#### Challenge Activity: 3.15.1 String with Digit
**Objective:**
Set `hasDigit` to true if the 3-character `passCode` contains a digit.
**Instructions:**
Complete the given C program to meet the specified objective. You need to write the logic that will determine if the `passCode` contains any digit character.
Here is the initial code provided for you:
```c
#include <stdio.h>
#include <string.h>
#include <stdbool.h>
#include <ctype.h>
int main(void) {
bool hasDigit;
char passCode[50];
hasDigit = false;
scanf("%s", passCode);
/* Your solution goes here */
if (hasDigit) {
printf("Has a digit.\n");
}
return 0;
}
```
**Steps to Follow:**
1. **Input Reading:**
The input `passCode` is read using `scanf`.
2. **Initialize `hasDigit`:**
Set the `hasDigit` variable to `false`.
3. **Logic Insertion:**
Insert logic to traverse the characters of `passCode` and set `hasDigit` to `true` if any character is a digit.
4. **Output:**
Print "Has a digit." if `hasDigit` is `true`.
- **Running the Program:**
Press the "Run" button to execute the program and verify the output.
#### Example: Checking for Digit
Consider the following `passCode` input: `abc123`
- The logic should properly identify that it contains digits.
#### Challenge Activity 3.15.2: Alphabetic Replace
**Objective:**
Replace any alphabetic character with `_` in a 2-character string `passCode`.
**Instructions:**
1. **Initialize:**
Read the `passCode` consisting of exactly 2 characters.
2. **Implementation:**
Use conditional statements to check each character of `passCode` using `isalpha()`. Replace alphabetic characters accordingly.
Example:
- Input: `9a`
- Output: `9_`
Please note that these challenge activities are crucial for understanding character operations in C, which is an essential skill in programming.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
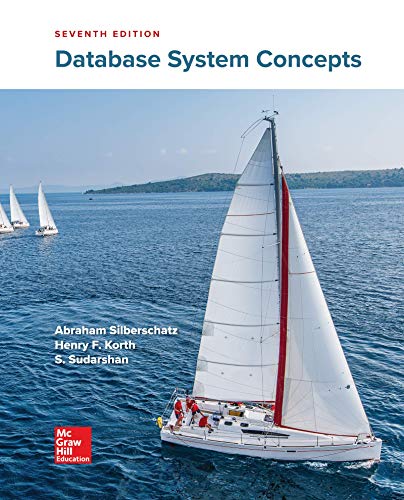
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
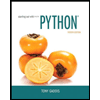
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
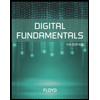
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
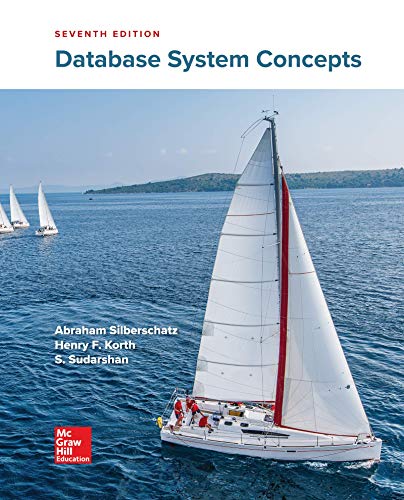
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
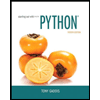
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
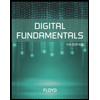
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
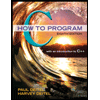
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
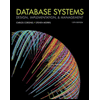
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
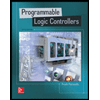
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education