Can you show me the screen shot of the following assembly code please INCLUDE Irvine32.inc .data lowerLimit dword 20 upperLimit dword 40 array_1 sdword 10,30,25,15,17,19,40,41,43 array_2 sdword 10,-30,25,15,-17,55,40,41,43 array_1_msg BYTE "Sum of array_1 elements within range: ", 0 array_2_msg BYTE "Sum of array_2 elements within range: ", 0 .code main PROC mov eax, 0 ; initialize sum to zero mov ebx, offset array_1 mov ecx, lowerLimit mov edx, upperLimit sum_loop: cmp ecx, edx ; check if lower limit <= upper limit jnle sum_done ; if not, we're done adding mov esi, [ebx+ecx*4] ; get array element at index ecx cmp esi, edx ; check if array element is within range jng not_in_range ; if not, skip adding to sum cmp esi, ecx ; check if array element is within range jnl in_range ; if so, add to sum not_in_range: inc ecx ; increment loop counter jmp sum_loop in_range: add eax, esi ; add array element to sum inc ecx ; increment loop counter jmp sum_loop sum_done: ; display sum on console for array_1 mov eax, OFFSET array_1_msg call WriteString mov eax, eax call WriteInt call Crlf ; display sum on console for array_2 mov eax, 0 mov ebx, offset array_2 mov ecx, lowerLimit mov edx, upperLimit mov eax, OFFSET array_2_msg call WriteString sum_loop_2: cmp ecx, edx jnle sum_done_2 mov esi, [ebx+ecx*4] cmp esi, edx jng not_in_range_2 cmp esi, ecx jnl in_range_2 not_in_range_2: inc ecx jmp sum_loop_2 in_range_2: add eax, esi inc ecx jmp sum_loop_2 sum_done_2: mov eax, eax call WriteInt call Crlf ; exit program mov eax, 0 ret main ENDP END
Can you show me the screen shot of the following assembly code please
INCLUDE Irvine32.inc
.data
lowerLimit dword 20
upperLimit dword 40
array_1 sdword 10,30,25,15,17,19,40,41,43
array_2 sdword 10,-30,25,15,-17,55,40,41,43
array_1_msg BYTE "Sum of array_1 elements within range: ", 0
array_2_msg BYTE "Sum of array_2 elements within range: ", 0
.code
main PROC
mov eax, 0 ; initialize sum to zero
mov ebx, offset array_1
mov ecx, lowerLimit
mov edx, upperLimit
sum_loop:
cmp ecx, edx ; check if lower limit <= upper limit
jnle sum_done ; if not, we're done adding
mov esi, [ebx+ecx*4] ; get array element at index ecx
cmp esi, edx ; check if array element is within range
jng not_in_range ; if not, skip adding to sum
cmp esi, ecx ; check if array element is within range
jnl in_range ; if so, add to sum
not_in_range:
inc ecx ; increment loop counter
jmp sum_loop
in_range:
add eax, esi ; add array element to sum
inc ecx ; increment loop counter
jmp sum_loop
sum_done:
; display sum on console for array_1
mov eax, OFFSET array_1_msg
call WriteString
mov eax, eax
call WriteInt
call Crlf
; display sum on console for array_2
mov eax, 0
mov ebx, offset array_2
mov ecx, lowerLimit
mov edx, upperLimit
mov eax, OFFSET array_2_msg
call WriteString
sum_loop_2:
cmp ecx, edx
jnle sum_done_2
mov esi, [ebx+ecx*4]
cmp esi, edx
jng not_in_range_2
cmp esi, ecx
jnl in_range_2
not_in_range_2:
inc ecx
jmp sum_loop_2
in_range_2:
add eax, esi
inc ecx
jmp sum_loop_2
sum_done_2:
mov eax, eax
call WriteInt
call Crlf
; exit
mov eax, 0
ret
main ENDP
END

Step by step
Solved in 2 steps with 1 images

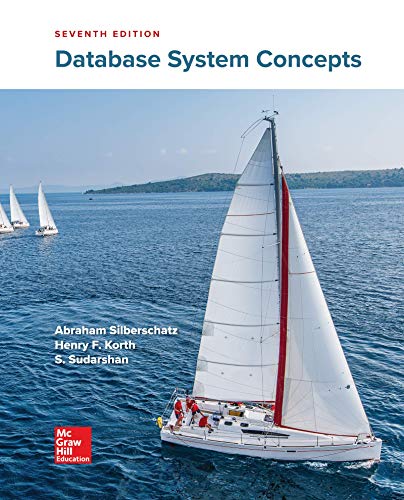
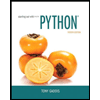
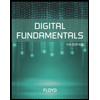
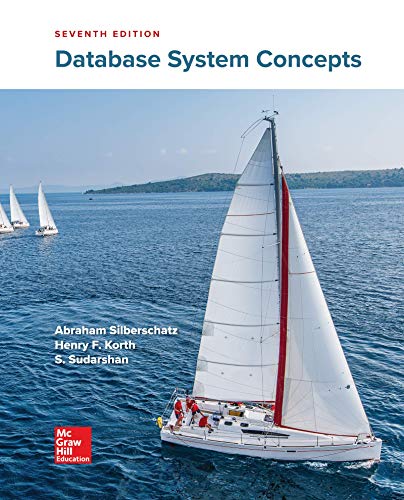
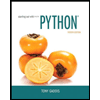
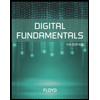
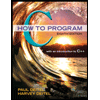
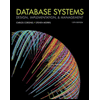
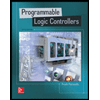