A 2-dimensional 3x3 array of ints, has been created and assigned to tictactoe. Write an expression whose value is TRUE if the elements of the first row are all equal.
A 2-dimensional 3x3 array of ints, has been created and assigned to tictactoe. Write an expression whose value is TRUE if the elements of the first row are all equal.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Java code please
![### Problem Description
A 2-dimensional 3x3 array of integers, named `tictactoe`, has been created and assigned. Write an expression whose value is `TRUE` if the elements of the first row are all equal.
### Solution
```plaintext
1 Enter your code
```
### Explanation
You are given a 3x3 array of integers and you need to determine if the elements in the first row of this array are all the same. This is commonly seen in problems related to game programming, such as checking for a winning condition in tic-tac-toe.
In the provided code input area, you need to write an expression that will return `TRUE` if all three elements in the first row of the `tictactoe` array are equal.
___Example Input:___
```python
tictactoe = [
[1, 1, 1],
[0, 2, 2],
[2, 0, 0]
]
```
___Example Output:___
The expression should return `TRUE` for the above `tictactoe` array because all the elements in the first row are equal.
### Pseudocode
Here’s a simple algorithm to solve this problem:
1. Access the first row of the `tictactoe` array.
2. Compare the first element with the second and the second element with the third.
3. If both comparisons are `TRUE`, then return `TRUE`, otherwise return `FALSE`.
### Implementation in Python
```python
tictactoe[0][0] == tictactoe[0][1] == tictactoe[0][2]
```
In this implementation:
- `tictactoe[0][0]` accesses the first element of the first row.
- `tictactoe[0][1]` and `tictactoe[0][2]` access the second and third elements of the first row respectively.
- The equality operator `==` checks if all the elements are equal.
This simple expression effectively checks if all three elements in the first row are equal and returns `TRUE` if they are, and `FALSE` otherwise.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fd6a7d46e-c507-421a-9895-6bbf67ae6667%2Fee6e0b2f-8873-4af8-a01f-38a715eacead%2Fo5pwmu5_processed.jpeg&w=3840&q=75)
Transcribed Image Text:### Problem Description
A 2-dimensional 3x3 array of integers, named `tictactoe`, has been created and assigned. Write an expression whose value is `TRUE` if the elements of the first row are all equal.
### Solution
```plaintext
1 Enter your code
```
### Explanation
You are given a 3x3 array of integers and you need to determine if the elements in the first row of this array are all the same. This is commonly seen in problems related to game programming, such as checking for a winning condition in tic-tac-toe.
In the provided code input area, you need to write an expression that will return `TRUE` if all three elements in the first row of the `tictactoe` array are equal.
___Example Input:___
```python
tictactoe = [
[1, 1, 1],
[0, 2, 2],
[2, 0, 0]
]
```
___Example Output:___
The expression should return `TRUE` for the above `tictactoe` array because all the elements in the first row are equal.
### Pseudocode
Here’s a simple algorithm to solve this problem:
1. Access the first row of the `tictactoe` array.
2. Compare the first element with the second and the second element with the third.
3. If both comparisons are `TRUE`, then return `TRUE`, otherwise return `FALSE`.
### Implementation in Python
```python
tictactoe[0][0] == tictactoe[0][1] == tictactoe[0][2]
```
In this implementation:
- `tictactoe[0][0]` accesses the first element of the first row.
- `tictactoe[0][1]` and `tictactoe[0][2]` access the second and third elements of the first row respectively.
- The equality operator `==` checks if all the elements are equal.
This simple expression effectively checks if all three elements in the first row are equal and returns `TRUE` if they are, and `FALSE` otherwise.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
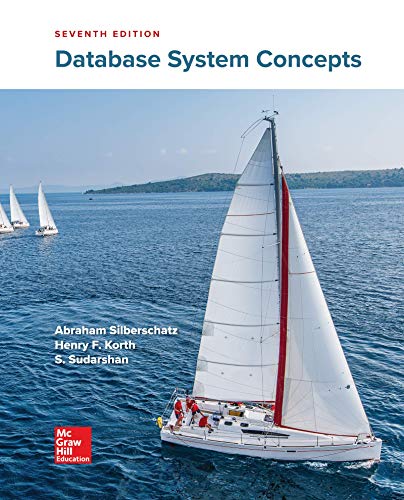
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
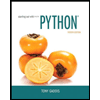
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
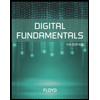
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
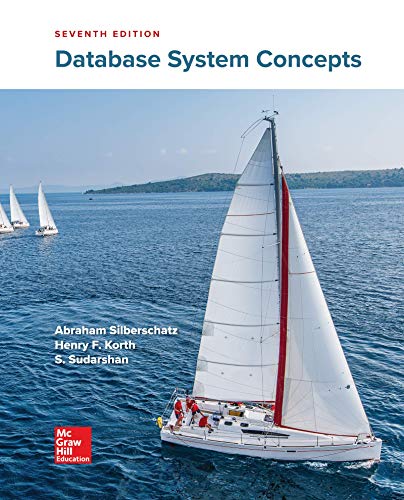
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
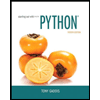
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
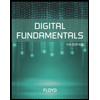
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
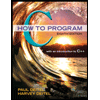
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
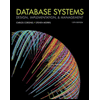
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
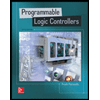
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education