Can you please help me write the code using the instruction below because my code won't run, it just crashed and I dont know why. Please help, i will give you a good rating! Thanks. Here is the main intruction ( I also attached the example output too): A client with a completed main function is provided. The main function should not be modified. You must update the client by creating and implementing the various functions described below. readFile – Loads the parameter array with Billionaire objects. The data for each Billionaire is read from the given data file which is in CSV format (comma delimited - 1 line per record with fields separated by a comma.) The function should read each line from the file, pass the line read to the Billionaire class constructor and store the resulting object in the parameter array. The second parameter represents the maximum number of Billionaire objects that can be stored. displayAll - Displays a list of the Billionaires stored in the array. getRange – Determines the smallest wealth value and the largest wealth value within the array. These values are returned to the caller via reference parameters. getWealthiest – Returns the Billionaire within the array with the greatest wealth. getUS – Returns a count of the number of individuals in the array with United States citizenship. Here is the incomplete main code: /** Client application that reads wealth data from a file, creates * a Billionaire object for each person listed, * and adds the object to an array. * The array of Billionaire objects is displayed, particular statistics * are calculated and displayed. * * @author Prof Gregory * @author YOUR NAME */ #include #include #include #include #include #include "Billionaire.h" using namespace std; // You may comment out this line and use std:: instead // Any prototypes? void readFile (string FILENAME, Billionaire*, const int count); void displayAll(Billionaire[], const int count); void getRange(Billionaire[], const int count, float&, float&); Billionaire getWealthiest(Billionaire[], const int count); int getUs (Billionaire[], const int count); int main() { const string FILENAME = "WealthyTechies.csv"; const int COUNT = 30; Billionaire billionaire[COUNT]; // Load billionaire array with Billionaire objects read from data file readFile(FILENAME,billionaire,COUNT); // Display the array of Billionaire objects displayAll(billionaire,COUNT); cout << endl; // Determine the range of wealth values and display float low; float high; getRange(billionaire,COUNT,low,high); cout << "The wealth of the billionaires range from $" << low << " B to $" << high << " B" << endl; // Determine the wealthiest Billionaire and display Billionaire richPerson = getWealthiest(billionaire,COUNT); cout << "The wealthiest technology person is " << endl; richPerson.printBillionaire(); // Determine how many are from the US and display int usTotal = getUs(billionaire, COUNT); cout << "There are " << usTotal << " US billionaires in the top 30!"; return 0; }
Can you please help me write the code using the instruction below because my code won't run, it just crashed and I dont know why. Please help, i will give you a good rating! Thanks.
Here is the main intruction ( I also attached the example output too):
A client with a completed main function is provided. The main function should not be modified. You must update the client by creating and implementing the various functions described below.
- readFile – Loads the parameter array with Billionaire objects. The data for each Billionaire is read from the given data file which is in CSV format (comma delimited - 1 line per record with fields separated by a comma.) The function should read each line from the file, pass the line read to the Billionaire class constructor and store the resulting object in the parameter array. The second parameter represents the maximum number of Billionaire objects that can be stored.
- displayAll - Displays a list of the Billionaires stored in the array.
- getRange – Determines the smallest wealth value and the largest wealth value within the array. These values are returned to the caller via reference parameters.
- getWealthiest – Returns the Billionaire within the array with the greatest wealth.
- getUS – Returns a count of the number of individuals in the array with United States citizenship.
Here is the incomplete main code:
/** Client application that reads wealth data from a file, creates
* a Billionaire object for each person listed,
* and adds the object to an array.
* The array of Billionaire objects is displayed, particular statistics
* are calculated and displayed.
*
* @author Prof Gregory
* @author YOUR NAME
*/
#include <iostream>
#include <fstream>
#include <iomanip>
#include <string>
#include <sstream>
#include "Billionaire.h"
using namespace std; // You may comment out this line and use std:: instead
// Any prototypes?
void readFile (string FILENAME, Billionaire*, const int count);
void displayAll(Billionaire[], const int count);
void getRange(Billionaire[], const int count, float&, float&);
Billionaire getWealthiest(Billionaire[], const int count);
int getUs (Billionaire[], const int count);
int main()
{
const string FILENAME = "WealthyTechies.csv";
const int COUNT = 30;
Billionaire billionaire[COUNT];
// Load billionaire array with Billionaire objects read from data file
readFile(FILENAME,billionaire,COUNT);
// Display the array of Billionaire objects
displayAll(billionaire,COUNT);
cout << endl;
// Determine the range of wealth values and display
float low;
float high;
getRange(billionaire,COUNT,low,high);
cout << "The wealth of the billionaires range from $" << low
<< " B to $" << high << " B" << endl;
// Determine the wealthiest Billionaire and display
Billionaire richPerson = getWealthiest(billionaire,COUNT);
cout << "The wealthiest technology person is " << endl;
richPerson.printBillionaire();
// Determine how many are from the US and display
int usTotal = getUs(billionaire, COUNT);
cout << "There are " << usTotal << " US billionaires in the top 30!";
return 0;
}


Trending now
This is a popular solution!
Step by step
Solved in 5 steps with 9 images

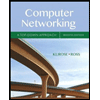
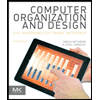
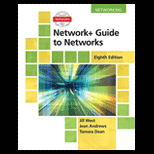
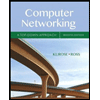
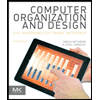
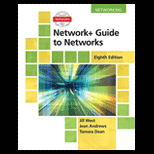
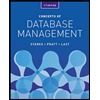
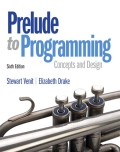
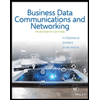