Can you please help me write a pseudocode in Java for the following questions
Can you please help me write a pseudocode in Java for the following questions
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Can you please help me write a pseudocode in Java for the following questions:

Transcribed Image Text:This assignment is adapted from Deitel & Deitel's textbook.
1. Write a program that reads in two integers and determines and prints if the first is a multiple of the second.
**Hint:** Use the modulus operator (%).
To achieve this, store the user's two numbers in `num1` and `num2` variables, and find the remainder when `num1` is divided by `num2`. Store this remainder in the `R` variable. If `R` is zero, then `num1` is a multiple of `num2`; otherwise, it is not.
**Example Screenshots:**
- First screenshot:
- Input: Enter two non-zero integers: `2892`, `2`
- Output: `2892 is a multiple of 2`
- Second screenshot:
- Input: Enter two non-zero integers: `26`, `3`
- Output: `26 is not a multiple of 3`
2. After your program works well, use a block comment to write your name and the purpose of this program.

Transcribed Image Text:### Program for Sorting Three Numbers
This educational content guides you through creating a simple program that requests three integers from the user and displays them in ascending order. The following is an example of the user interface:
---
**Example 1:**
```
Please enter any three integers:
900 200 400
You entered 900, 200 and 400.
The ascending order of them are 200, 400 and 900.
```
**Example 2:**
```
Please enter any three integers:
100 200 150
You entered 100, 200 and 150.
The ascending order of them are 100, 150 and 200.
```
---
### Flowchart Explanation
The accompanying flowchart provides a step-by-step algorithm for achieving this sorting task.
1. **Start**: Begin the program.
2. **Declare Memory Boxes**: Initialize four variables, `a`, `b`, `c`, and `temp`, to hold the data.
3. **Prompt User**: Display a message asking the user to enter three numbers.
4. **Input Numbers**: Assign the user's input to the variables `a`, `b`, and `c`.
5. **Swap and Sort Procedure**:
- **First Condition**: Check if `b < a`. If true, swap the values of `a` and `b`.
- **Second Condition**: Check if `c < a`. If true, swap the values of `a` and `c`.
- **Third Condition**: Check if `c < b`. If true, swap the values of `b` and `c`.
6. **Output**: Print the values of `a`, `b`, and `c`, which are now in ascending order.
7. **Stop**: End the program.
By following this structured approach, the chosen integers will automatically be sorted in ascending order upon execution of the program.
Expert Solution

Pseudocode for part 1
Input the value of num1
Input the value of num2
Declare a variable R
Store the value of remainder in R
R<- num1% num2;
if R==0 :
print num1 is multiple of num 2
else:
print num1 is not multiple of num2
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
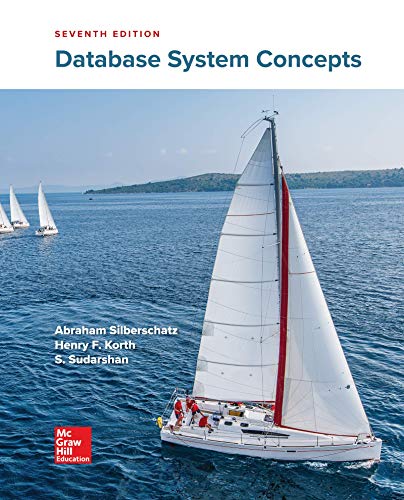
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
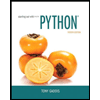
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
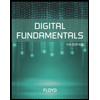
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
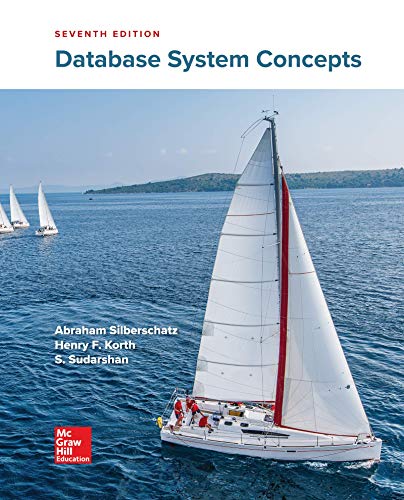
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
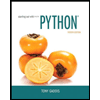
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
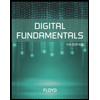
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
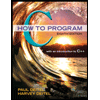
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
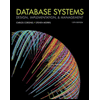
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
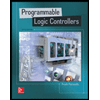
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education