Can you please clean up my code? I think there is an implementation problem. Thank you. #include #include #include using namespace std; void enqQ(int&); void deqQ(int&); void clearQ(int&, int&); bool isEmpty(int, int); bool isFull(int, int); struct planes // data type plane declared via struct { stringrequest; stringflightNum; stringstartingPoint; stringdestination; stringnumberOfPassengers; }; void enqQ(int& rear) // adds data to queue { rear = (rear + 1) % 10; } void deqQ(int& front) // delets data from queue { front = (front + 1) % 10; } void clearQ(int& front, int& rear) // clears queue { front = -1; rear = -1; } bool isEmpty(int front, int rear) // tells if queue is empty or not { if (rear == front) return true; else return false; } bool isFull(int front, int rear) // tells if queue is full or not { if ((rear + 1) % 10 == front) return true; else return false; } int main() { planes normalQueue[15]; planes emergencyQueue[10]; planes plane; fstream fin; int normalFront; int normalRear; int emergencyFront; int emergencyRear; int time; string planeInfo; clearQ(normalFront, normalRear); clearQ(emergencyFront, emergencyRear); fin.open("Airport Queue Data.txt"); // makes sure that named text is there if (!fin) { cerr << "\ncould not open file"; exit(-1); } time = 1; // time is declared to 1 while (!fin.eof()) // reads data until end of file { cout << "Time Cycle = " << time << ":00" << endl; // prints time cycle getline(fin, planeInfo); // gets entire line and puts it in planeInfo which is temp variable plane.request = planeInfo.substr(0, 8); // these function reads specific characters from planeInfo and stores them into proper areas of the struct plane.flightNum = planeInfo.substr(8, 3); plane.startingPoint = planeInfo.substr(11, 8); plane.destination = planeInfo.substr(19, 8); plane.numberOfPassengers = planeInfo.substr(27, 3); if (plane.request == "NORMAL ") // if plane request is normal then it will be place into normal queue { cout << "Plane added to Normal Queue" << endl; enqQ(normalRear); // normalRear increases normalQueue[normalRear] = plane; // adds plane to normalqueue and prints out plane info cout << plane.flightNum << "\t"; cout << plane.startingPoint << "\t"; cout << plane.destination << "\t"; cout << plane.numberOfPassengers << "\n"; cout << "---------------############---------------------\n" << endl; } else { cout << "Plane added to Emergency Queue" << endl; enqQ(emergencyRear); // emergency rear increases emergencyQueue[emergencyRear] = plane; // adds plane to emergency Queue and prints out plane info cout << plane.flightNum << "\t"; cout << plane.startingPoint << "\t"; cout << plane.destination << "\t"; cout << plane.numberOfPassengers << "\n"; cout << "---------------############---------------------\n" << endl; } if (time % 2 == 0) // plane will land only if time is even { if (!isEmpty(emergencyFront, emergencyRear)) // checks if emergency queue is empty if not than it will be prioritize to land { cout << "Emergency Plane Landed" << endl; deqQ(emergencyFront); // increments emergency front and print plane info cout << emergencyQueue[emergencyFront].flightNum << "\t"; cout << emergencyQueue[emergencyFront].startingPoint << "\t"; cout << emergencyQueue[emergencyFront].destination << "\t"; cout << emergencyQueue[emergencyFront].numberOfPassengers << endl; cout << "---------------############---------------------\n" << endl; } else { cout << "Normal Plane Landed" << endl; deqQ(normalFront); // increments normal front and prints plane info cout << normalQueue[normalFront].flightNum << "\t"; cout << normalQueue[normalFront].startingPoint << "\t"; cout << normalQueue[normalFront].destination << "\t"; cout << normalQueue[normalFront].numberOfPassengers << endl; cout << "---------------############---------------------\n" << endl; } } time++; // increments time if (time > 24) // check time cycle resets to 1 when it reaches 24 { time = 1; } } fin.close(); // closes fin file cout << "\n\n\n Flights still in Queues" << endl; // prints planes still left in queues cout << "\nNormal Queue: " << endl; while (!isEmpty(normalFront, normalRear)) // prints planes that are left in normal queue { deqQ(normalFront); cout << normalQueue[normalFront].request << "\t"; cout << normalQueue[normalFront].flightNum << "\t"; cout << normalQueue[normalFront].startingPoint << "\t"; cout << normalQueue[normalFront].destination << "\t"; cout << normalQueue[normalFront].numberOfPassengers << endl; } cout << "\nEmergency Queue: " << endl; while (!isEmpty(emergencyFront, emergencyRear)) // prints planes that are left in emergency queue { deqQ(emergencyFront); cout << emergencyQueue[emergencyFront].request << "\t"; cout << emergencyQueue[emergencyFront].flightNum << "\t"; cout << emergencyQueue[emergencyFront].startingPoint << "\t"; cout << emergencyQueue[emergencyFront].destination << "\t"; cout << emergencyQueue[emergencyFront].numberOfPassengers << endl; } return 0; }
Can you please clean up my code? I think there is an implementation problem. Thank you.
#include <iostream>
#include <fstream>
#include <string>
using namespace std;
void enqQ(int&);
void deqQ(int&);
void clearQ(int&, int&);
bool isEmpty(int, int);
bool isFull(int, int);
struct planes // data type plane declared via struct
{
stringrequest;
stringflightNum;
stringstartingPoint;
stringdestination;
stringnumberOfPassengers;
};
void enqQ(int& rear) // adds data to queue
{
rear = (rear + 1) % 10;
}
void deqQ(int& front) // delets data from queue
{
front = (front + 1) % 10;
}
void clearQ(int& front, int& rear) // clears queue
{
front = -1;
rear = -1;
}
bool isEmpty(int front, int rear) // tells if queue is empty or not
{
if (rear == front)
return true;
else
return false;
}
bool isFull(int front, int rear) // tells if queue is full or not
{
if ((rear + 1) % 10 == front)
return true;
else
return false;
}
int main()
{
planes normalQueue[15];
planes emergencyQueue[10];
planes plane;
fstream fin;
int normalFront;
int normalRear;
int emergencyFront;
int emergencyRear;
int time;
string planeInfo;
clearQ(normalFront, normalRear);
clearQ(emergencyFront, emergencyRear);
fin.open("Airport Queue Data.txt"); // makes sure that named text is there
if (!fin)
{
cerr << "\ncould not open file";
exit(-1);
}
time = 1; // time is declared to 1
while (!fin.eof()) // reads data until end of file
{
cout << "Time Cycle = " << time << ":00" << endl; // prints time cycle
getline(fin, planeInfo); // gets entire line and puts it in planeInfo which is temp variable
plane.request = planeInfo.substr(0, 8); // these function reads specific characters from planeInfo and stores them into proper areas of the struct
plane.flightNum = planeInfo.substr(8, 3);
plane.startingPoint = planeInfo.substr(11, 8);
plane.destination = planeInfo.substr(19, 8);
plane.numberOfPassengers = planeInfo.substr(27, 3);
if (plane.request == "NORMAL ") // if plane request is normal then it will be place into normal queue
{
cout << "Plane added to Normal Queue" << endl;
enqQ(normalRear); // normalRear increases
normalQueue[normalRear] = plane; // adds plane to normalqueue and prints out plane info
cout << plane.flightNum << "\t";
cout << plane.startingPoint << "\t";
cout << plane.destination << "\t";
cout << plane.numberOfPassengers << "\n";
cout << "---------------############---------------------\n" << endl;
}
else
{
cout << "Plane added to Emergency Queue" << endl;
enqQ(emergencyRear); // emergency rear increases
emergencyQueue[emergencyRear] = plane; // adds plane to emergency Queue and prints out plane info
cout << plane.flightNum << "\t";
cout << plane.startingPoint << "\t";
cout << plane.destination << "\t";
cout << plane.numberOfPassengers << "\n";
cout << "---------------############---------------------\n" << endl;
}
if (time % 2 == 0) // plane will land only if time is even
{
if (!isEmpty(emergencyFront, emergencyRear)) // checks if emergency queue is empty if not than it will be prioritize to land
{
cout << "Emergency Plane Landed" << endl;
deqQ(emergencyFront); // increments emergency front and print plane info
cout << emergencyQueue[emergencyFront].flightNum << "\t";
cout << emergencyQueue[emergencyFront].startingPoint << "\t";
cout << emergencyQueue[emergencyFront].destination << "\t";
cout << emergencyQueue[emergencyFront].numberOfPassengers << endl;
cout << "---------------############---------------------\n" << endl;
}
else
{
cout << "Normal Plane Landed" << endl;
deqQ(normalFront); // increments normal front and prints plane info
cout << normalQueue[normalFront].flightNum << "\t";
cout << normalQueue[normalFront].startingPoint << "\t";
cout << normalQueue[normalFront].destination << "\t";
cout << normalQueue[normalFront].numberOfPassengers << endl;
cout << "---------------############---------------------\n" << endl;
}
}
time++; // increments time
if (time > 24) // check time cycle resets to 1 when it reaches 24
{
time = 1;
}
}
fin.close(); // closes fin file
cout << "\n\n\n Flights still in Queues" << endl; // prints planes still left in queues
cout << "\nNormal Queue: " << endl;
while (!isEmpty(normalFront, normalRear)) // prints planes that are left in normal queue
{
deqQ(normalFront);
cout << normalQueue[normalFront].request << "\t";
cout << normalQueue[normalFront].flightNum << "\t";
cout << normalQueue[normalFront].startingPoint << "\t";
cout << normalQueue[normalFront].destination << "\t";
cout << normalQueue[normalFront].numberOfPassengers << endl;
}
cout << "\nEmergency Queue: " << endl;
while (!isEmpty(emergencyFront, emergencyRear)) // prints planes that are left in emergency queue
{
deqQ(emergencyFront);
cout << emergencyQueue[emergencyFront].request << "\t";
cout << emergencyQueue[emergencyFront].flightNum << "\t";
cout << emergencyQueue[emergencyFront].startingPoint << "\t";
cout << emergencyQueue[emergencyFront].destination << "\t";
cout << emergencyQueue[emergencyFront].numberOfPassengers << endl;
}
return 0;
}


Step by step
Solved in 3 steps with 6 images

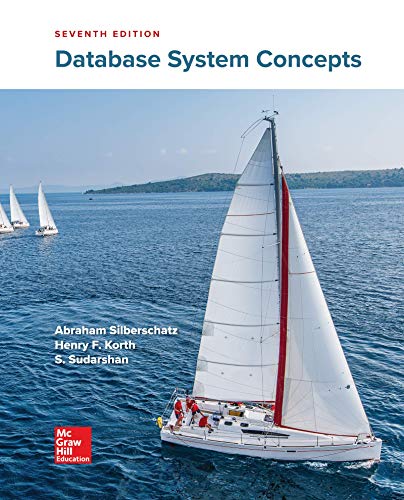
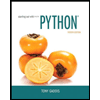
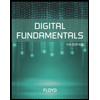
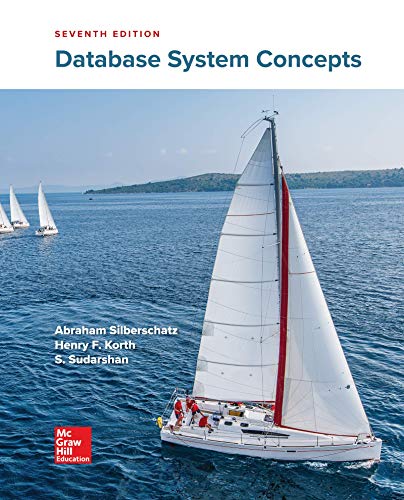
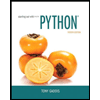
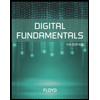
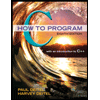
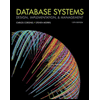
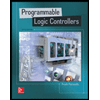