can you help debug my code? it's a JavaFX program to convert miles/kilometers or celsius/farenheit or pounds/kilograms. It looks like I haven't imported something, but I'm not sure what?? errors: lines 4, 12: package does not exist lines: 3, 1: javafx.swing does not exist lines 17, 9:, 25 cannot find symbol JFrame lines 18, 9, 25, 19: cannot find symbol JLabel lines 20, 9, 29, 21: cannot find symbol JTextField lines 22, 9, 24, 29, 25: cannot find jRadioButton lines 26, 9, 29: cannot find symbol ButtonGroup lines 28, 9, 48: cannot find symbol Action lines 28, 30, 48: cannot find symbol AbstractAction lines 30, 13, 50: method does not override or implement a method from a supertype lines 70, 9, 24: cannot find symbol JPanel my code: import java.awt.*; import java.awt.event.*; import javafx.swing.*; import java.Math; class A { public static double round(double value, int places) { if (places < 0) throw new IllegalArgumentException(); long factor = (long) Math.pow(10, places); value = value * factor; long tmp = Math.round(value); return (double) tmp / factor; } public static void main(String[] args) { JFrame f = new JFrame(); JLabel l1 = new JLabel("Miles"); JLabel l2 = new JLabel("KiloMeters"); JTextField t1 = new JTextField(10); JTextField t2 = new JTextField(10); JRadioButton r1=new JRadioButton("Miles - KiloMeters"); r1.setSelected(true); JRadioButton r2=new JRadioButton("Celsius and Fahrenheit"); JRadioButton r3=new JRadioButton("Pounds and Kilograms"); ButtonGroup b = new ButtonGroup(); b.add(r1);b.add(r2);b.add(r3); Action action1 = new AbstractAction() { @Override public void actionPerformed(ActionEvent e) { double d = Integer.parseInt(t1.getText()); double d1=0.0; if(r1.isSelected()){ d1 = d * 1.609344; } else if(r2.isSelected()){ d1 = d - 32 * 5/9; } else if(r3.isSelected()){ d1 = d * 0.45359237; } d1 = round(d1,2); t2.setText(d1+""); } }; Action action2 = new AbstractAction() { @Override public void actionPerformed(ActionEvent e) { double d = Integer.parseInt(t2.getText()); double d1=0.0; if(r1.isSelected()){ d1 = d / 1.609344; } else if(r2.isSelected()){ d1 = d * (9/5) + 32; } else if(r3.isSelected()){ d1 = d / 0.45359237; } d1 = round(d1,2); t1.setText(d1+""); } }; t1.addActionListener( action1 ); t2.addActionListener( action2 ); JPanel p = new JPanel(); p.add(l1); p.add(t1); p.add(l2); p.add(t2); p.add(r1); p.add(r2); p.add(r3); f.add(p); r1.addItemListener(new ItemListener() { public void itemStateChanged(ItemEvent e) { if(r1.isSelected()){ l1.setText("Miles"); l2.setText("KiloMeters"); } } }); r2.addItemListener(new ItemListener() { public void itemStateChanged(ItemEvent e) { if(r2.isSelected()){ l1.setText("Celsius"); l2.setText("Fahrenheit"); } } }); r3.addItemListener(new ItemListener() { public void itemStateChanged(ItemEvent e) { if(r3.isSelected()){ l1.setText("Pounds"); l2.setText("Kilograms"); } } }); f.setSize(500,500); f.setVisible(true); } }
can you help debug my code? it's a JavaFX program to convert miles/kilometers or celsius/farenheit or pounds/kilograms. It looks like I haven't imported something, but I'm not sure what??
errors:
lines 4, 12: package does not exist
lines: 3, 1: javafx.swing does not exist
lines 17, 9:, 25 cannot find symbol JFrame
lines 18, 9, 25, 19: cannot find symbol JLabel
lines 20, 9, 29, 21: cannot find symbol JTextField
lines 22, 9, 24, 29, 25: cannot find jRadioButton
lines 26, 9, 29: cannot find symbol ButtonGroup
lines 28, 9, 48: cannot find symbol Action
lines 28, 30, 48: cannot find symbol AbstractAction
lines 30, 13, 50: method does not override or implement a method from a supertype
lines 70, 9, 24: cannot find symbol JPanel
my code:
import java.awt.*;
import java.awt.event.*;
import javafx.swing.*;
import java.Math;
class A {
public static double round(double value, int places) {
if (places < 0) throw new IllegalArgumentException();
long factor = (long) Math.pow(10, places);
value = value * factor;
long tmp = Math.round(value);
return (double) tmp / factor;
}
public static void main(String[] args) {
JFrame f = new JFrame();
JLabel l1 = new JLabel("Miles");
JLabel l2 = new JLabel("KiloMeters");
JTextField t1 = new JTextField(10);
JTextField t2 = new JTextField(10);
JRadioButton r1=new JRadioButton("Miles - KiloMeters");
r1.setSelected(true);
JRadioButton r2=new JRadioButton("Celsius and Fahrenheit");
JRadioButton r3=new JRadioButton("Pounds and Kilograms");
ButtonGroup b = new ButtonGroup();
b.add(r1);b.add(r2);b.add(r3);
Action action1 = new AbstractAction()
{
@Override
public void actionPerformed(ActionEvent e)
{
double d = Integer.parseInt(t1.getText());
double d1=0.0;
if(r1.isSelected()){
d1 = d * 1.609344;
}
else if(r2.isSelected()){
d1 = d - 32 * 5/9;
}
else if(r3.isSelected()){
d1 = d * 0.45359237;
}
d1 = round(d1,2);
t2.setText(d1+"");
}
};
Action action2 = new AbstractAction()
{
@Override
public void actionPerformed(ActionEvent e)
{
double d = Integer.parseInt(t2.getText());
double d1=0.0;
if(r1.isSelected()){
d1 = d / 1.609344;
}
else if(r2.isSelected()){
d1 = d * (9/5) + 32;
}
else if(r3.isSelected()){
d1 = d / 0.45359237;
}
d1 = round(d1,2);
t1.setText(d1+"");
}
};
t1.addActionListener( action1 );
t2.addActionListener( action2 );
JPanel p = new JPanel();
p.add(l1);
p.add(t1);
p.add(l2);
p.add(t2);
p.add(r1);
p.add(r2);
p.add(r3);
f.add(p);
r1.addItemListener(new ItemListener()
{
public void itemStateChanged(ItemEvent e)
{
if(r1.isSelected()){
l1.setText("Miles");
l2.setText("KiloMeters");
}
}
});
r2.addItemListener(new ItemListener()
{
public void itemStateChanged(ItemEvent e)
{
if(r2.isSelected()){
l1.setText("Celsius");
l2.setText("Fahrenheit");
}
}
});
r3.addItemListener(new ItemListener()
{
public void itemStateChanged(ItemEvent e)
{
if(r3.isSelected()){
l1.setText("Pounds");
l2.setText("Kilograms");
}
}
});
f.setSize(500,500);
f.setVisible(true);
}
}

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 5 images

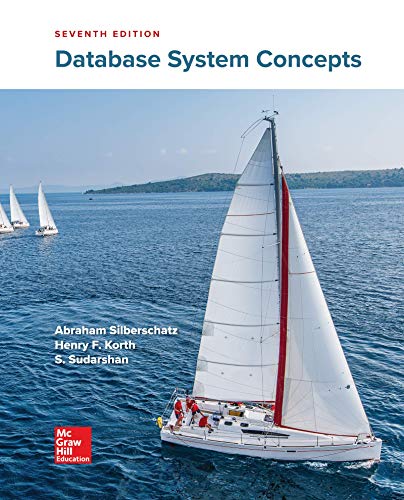
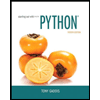
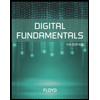
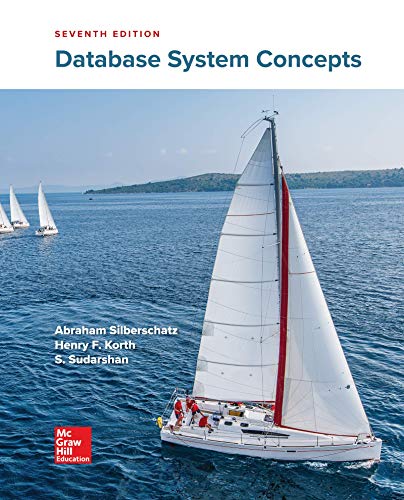
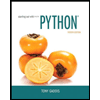
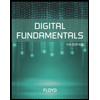
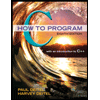
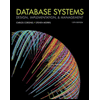
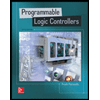