Can you debug my code? It is supposed to be a candy machine using three objects but there is an error I cannot find. Thank you Main: public class Main { public static void main(String[] args){ System.out.println("Press any number to start"); CandyMachine candyMachine = new CandyMachine();//asks for a number candyMachine.On(); } } Candy Machine: import java.util.*; public class CandyMachine{ CashRegister cashRegister; Dispenser candy; Dispenser chips; Dispenser gum; Dispenser cookies; CashRegister register; public CandyMachine(){ CashRegister register = new CashRegister(); Dispenser candy = new Dispenser(0.50, 20); Dispenser chips = new Dispenser(0.60, 20); Dispenser gum = new Dispenser(0.25, 20); Dispenser cookies = new Dispenser(0.85, 20); Scanner console = new Scanner(System.in); cashRegister = new CashRegister(console.nextInt()); } public void On(){ showSelection(); int choice; do{ Scanner input = new Scanner(System.in); choice = input.nextInt(); switch (choice) { case 1: System.out.println("That is not an option, please try again."); sellProduct(candy, register); break; case 2: sellProduct(chips, register); break; case 3: sellProduct(gum, register); break; case 4: sellProduct(cookies, register); break; case 9: break; default: System.out.println("That is not an option, please try again."); } } while (choice != 9); } private void showSelection(){ System.out.println("*** Welcome ***"); System.out.println("To select an item, enter "); System.out.println("0 for Adding more cash"); System.out.println("1 for Candy"); System.out.println("2 for Chips"); System.out.println("3 for Gum"); System.out.println("4 for Cookies"); System.out.println("9 to exit"); } private void sellProduct(Dispenser product, CashRegister cashRegister){ Scanner console = new Scanner(System.in); if(product.getCount() > 0){ if(product.getProductCost() <= cashRegister.currentBalance()) { cashRegister.deductAmount(product.getProductCost()); product.makeSale(); System.out.println("Collect item"); }else{ System.out.println("Plese try again! Insufficent funds"); } }else{ System.out.println("Your choosen item is sold out, choose another one"); } } } Register: public class CashRegister{ private int cashOnHand=0; public CashRegister(){ cashOnHand = 10; } public CashRegister(int cashIn){ cashOnHand = cashIn; //return cashIn; } public int currentBalance(){ return cashOnHand; } public void acceptAmount(int amountIn){ if(amountIn <= 0){ System.out.println("Sorry, but you have to add money."); }else{ cashOnHand = cashOnHand + amountIn; } } public void deductAmount(int amountCost) { cashOnHand= amountCost--; } } Dispenser: public class Dispenser{ private int numberOfItems; private int cost; private int prodQty; private double prodCost; Dispenser(double cost, int numberOfItems) { //prodCost = cost; prodQty = numberOfItems; } double getProdCost() { return prodCost; } int getProdQty() { return prodQty; } void setProdQty(int numberOfItems) { prodQty -= numberOfItems; } public Dispenser(int setNoOfItems, int setCost){ //prodCost = cost; prodQty = numberOfItems; } public int getCount(){ return numberOfItems; } public int getProductCost(){ return cost; // -- Your Code Here } public void makeSale(){ numberOfItems--; } }
Can you debug my code? It is supposed to be a candy machine using three objects but there is an error I cannot find. Thank you
Main:
public class Main {
public static void main(String[] args){
System.out.println("Press any number to start");
CandyMachine candyMachine = new CandyMachine();//asks for a number
candyMachine.On();
}
}
Candy Machine:
import java.util.*;
public class CandyMachine{
CashRegister cashRegister;
Dispenser candy;
Dispenser chips;
Dispenser gum;
Dispenser cookies;
CashRegister register;
public CandyMachine(){
CashRegister register = new CashRegister();
Dispenser candy = new Dispenser(0.50, 20);
Dispenser chips = new Dispenser(0.60, 20);
Dispenser gum = new Dispenser(0.25, 20);
Dispenser cookies = new Dispenser(0.85, 20);
Scanner console = new Scanner(System.in);
cashRegister = new CashRegister(console.nextInt());
}
public void On(){
showSelection();
int choice;
do{
Scanner input = new Scanner(System.in);
choice = input.nextInt();
switch (choice) {
case 1:
System.out.println("That is not an option, please try again.");
sellProduct(candy, register);
break;
case 2:
sellProduct(chips, register);
break;
case 3:
sellProduct(gum, register);
break;
case 4:
sellProduct(cookies, register);
break;
case 9:
break;
default:
System.out.println("That is not an option, please try again.");
}
}
while (choice != 9);
}
private void showSelection(){
System.out.println("*** Welcome ***");
System.out.println("To select an item, enter ");
System.out.println("0 for Adding more cash");
System.out.println("1 for Candy");
System.out.println("2 for Chips");
System.out.println("3 for Gum");
System.out.println("4 for Cookies");
System.out.println("9 to exit");
}
private void sellProduct(Dispenser product, CashRegister cashRegister){
Scanner console = new Scanner(System.in);
if(product.getCount() > 0){
if(product.getProductCost() <= cashRegister.currentBalance()) {
cashRegister.deductAmount(product.getProductCost());
product.makeSale();
System.out.println("Collect item");
}else{
System.out.println("Plese try again! Insufficent funds");
}
}else{
System.out.println("Your choosen item is sold out, choose another one");
}
}
}
Register:
public class CashRegister{
private int cashOnHand=0;
public CashRegister(){
cashOnHand = 10;
}
public CashRegister(int cashIn){
cashOnHand = cashIn;
//return cashIn;
}
public int currentBalance(){
return cashOnHand;
}
public void acceptAmount(int amountIn){
if(amountIn <= 0){
System.out.println("Sorry, but you have to add money.");
}else{
cashOnHand = cashOnHand + amountIn;
}
}
public void deductAmount(int amountCost)
{
cashOnHand= amountCost--;
}
}
Dispenser:
public class Dispenser{
private int numberOfItems;
private int cost;
private int prodQty;
private double prodCost;
Dispenser(double cost, int numberOfItems) {
//prodCost = cost;
prodQty = numberOfItems;
}
double getProdCost() {
return prodCost;
}
int getProdQty() {
return prodQty;
}
void setProdQty(int numberOfItems) {
prodQty -= numberOfItems;
}
public Dispenser(int setNoOfItems, int setCost){
//prodCost = cost;
prodQty = numberOfItems;
}
public int getCount(){
return numberOfItems;
}
public int getProductCost(){
return cost;
// -- Your Code Here
}
public void makeSale(){
numberOfItems--;
}
}

Step by step
Solved in 4 steps with 2 images

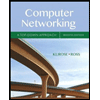
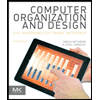
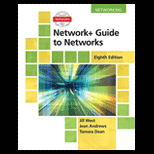
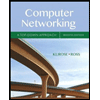
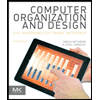
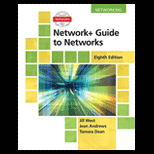
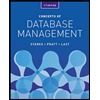
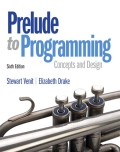
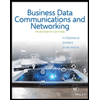