Can someone please help with the following question. PLEASE READ THE END: I have to print the following syscalls: $ trace 32 grep hello README PID=8 (grep): syscall read --> 1023 PID=8 (grep): syscall read --> 961 PID=8 (grep): syscall read --> 321 PID=8 (grep): syscall read --> 0 $ $ trace 4294967295 grep hello README PID=12 (trace): syscall trace --> 0 PID=12 (grep): syscall exec --> 3 PID=12 (grep): syscall open --> 3 PID=12 (grep): syscall read --> 1023 PID=12 (grep): syscall read --> 961 PID=12 (grep): syscall read --> 321 PID=12 (grep): syscall read --> 0 PID=12 (grep): syscall close --> 0 $ $ trace 4294967295 echo hi PID=16 (trace): syscall trace --> 0 PID=16 (echo): syscall exec --> 2 hiPID=16 (echo): syscall write --> 2 PID=16 (echo): syscall write --> 1 $ Your trace program should use new system call trace(int mask), which takes a bitmask as a parameter. The bitmask indicates which system calls to trace. A bit value of ‘1’ in a position 2syscall_no means that the system call with the number syscall_no is to be traced. If the bit value is ‘0’, the system call is not to be traced. For example, to trace system call number 5 only, the user would specify bit mask 25=32. To trace system calls 5 and 2, the user would specify the bit mask 25 + 22 = 32+4 = 36. To trace all system calls from 0 to 31, the user can specify bitmask 232-1 = 4294967295. You will need to extend proc structure with a new field, which will keep the tracing bitmask. Do not forget to copy the bitmask value from the parent proc structure to the child proc structure inside the fork() system call. The printing of the tracing message should be performed just before returning from system call to the user process inside syscall() funciton. The format of the message is: PID=pid ( name_of_the_process ): syscall name_of_syscall --> value_returned_from_syscall Hints: • add $U/_trace to UPROGS in Makefile • create file trace.c in user/ subfolder of xv6-riscv • add new syscall number in kernel/syscall.h and a matching entry in user/usys.pl, which will automaticallt generate an assembly language function doing ecall in usys.S; consider adding trace() function declaration to user/user.h • write initial version of trace.c and make sure that it can run external programs with parameters supplied on command line. • Add new field to proc structure in kernel/proc.h to hold the tracing bitmask, and add sys_trace() function in kernel/sysproc.c to save the value passed as a syscall parameter into it. • modify fork() in kernel/proc.c to copy the tracing bitmask from the parent to the newly created child process. Version 1.0, Page 5 of 7 I'VE FILLED IN A LOT OF THE CODE ALREADY HOWEVER, I'M STRUGGLING PRINTING IT OUT CORRECTLY: THIS IS THE CODE WE'RE GIVEN TO WORK WITH: void syscall(void) { intnum; structproc *p = myproc(); num = p->trapframe->a7; if(num > 0 && num < NELEM(syscalls) && syscalls[num]) { // Use num to lookup the system call function for num, call it, // and store its return value in p->trapframe->a0 p->trapframe->a0 = syscalls[num](); } else { printf("%d%s: unknown sys call %d\n", p->pid, p->name, num); p->trapframe->a0 = -1; } }
Can someone please help with the following question. PLEASE READ THE END: I have to print the following syscalls: $ trace 32 grep hello README PID=8 (grep): syscall read --> 1023 PID=8 (grep): syscall read --> 961 PID=8 (grep): syscall read --> 321 PID=8 (grep): syscall read --> 0 $ $ trace 4294967295 grep hello README PID=12 (trace): syscall trace --> 0 PID=12 (grep): syscall exec --> 3 PID=12 (grep): syscall open --> 3 PID=12 (grep): syscall read --> 1023 PID=12 (grep): syscall read --> 961 PID=12 (grep): syscall read --> 321 PID=12 (grep): syscall read --> 0 PID=12 (grep): syscall close --> 0 $ $ trace 4294967295 echo hi PID=16 (trace): syscall trace --> 0 PID=16 (echo): syscall exec --> 2 hiPID=16 (echo): syscall write --> 2 PID=16 (echo): syscall write --> 1 $ Your trace program should use new system call trace(int mask), which takes a bitmask as a parameter. The bitmask indicates which system calls to trace. A bit value of ‘1’ in a position 2syscall_no means that the system call with the number syscall_no is to be traced. If the bit value is ‘0’, the system call is not to be traced. For example, to trace system call number 5 only, the user would specify bit mask 25=32. To trace system calls 5 and 2, the user would specify the bit mask 25 + 22 = 32+4 = 36. To trace all system calls from 0 to 31, the user can specify bitmask 232-1 = 4294967295. You will need to extend proc structure with a new field, which will keep the tracing bitmask. Do not forget to copy the bitmask value from the parent proc structure to the child proc structure inside the fork() system call. The printing of the tracing message should be performed just before returning from system call to the user process inside syscall() funciton. The format of the message is: PID=pid ( name_of_the_process ): syscall name_of_syscall --> value_returned_from_syscall Hints: • add $U/_trace to UPROGS in Makefile • create file trace.c in user/ subfolder of xv6-riscv • add new syscall number in kernel/syscall.h and a matching entry in user/usys.pl, which will automaticallt generate an assembly language function doing ecall in usys.S; consider adding trace() function declaration to user/user.h • write initial version of trace.c and make sure that it can run external programs with parameters supplied on command line. • Add new field to proc structure in kernel/proc.h to hold the tracing bitmask, and add sys_trace() function in kernel/sysproc.c to save the value passed as a syscall parameter into it. • modify fork() in kernel/proc.c to copy the tracing bitmask from the parent to the newly created child process. Version 1.0, Page 5 of 7 I'VE FILLED IN A LOT OF THE CODE ALREADY HOWEVER, I'M STRUGGLING PRINTING IT OUT CORRECTLY: THIS IS THE CODE WE'RE GIVEN TO WORK WITH: void syscall(void) { intnum; structproc *p = myproc(); num = p->trapframe->a7; if(num > 0 && num < NELEM(syscalls) && syscalls[num]) { // Use num to lookup the system call function for num, call it, // and store its return value in p->trapframe->a0 p->trapframe->a0 = syscalls[num](); } else { printf("%d%s: unknown sys call %d\n", p->pid, p->name, num); p->trapframe->a0 = -1; } }
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Topic Video
Question
Can someone please help with the following question.
PLEASE READ THE END:
I have to print the following syscalls:
$ trace 32 grep hello README
PID=8 (grep): syscall read --> 1023
PID=8 (grep): syscall read --> 961
PID=8 (grep): syscall read --> 321
PID=8 (grep): syscall read --> 0
$
$ trace 4294967295 grep hello README
PID=12 (trace): syscall trace --> 0
PID=12 (grep): syscall exec --> 3
PID=12 (grep): syscall open --> 3
PID=12 (grep): syscall read --> 1023
PID=12 (grep): syscall read --> 961
PID=12 (grep): syscall read --> 321
PID=12 (grep): syscall read --> 0
PID=12 (grep): syscall close --> 0
$
$ trace 4294967295 echo hi
PID=16 (trace): syscall trace --> 0
PID=16 (echo): syscall exec --> 2
hiPID=16 (echo): syscall write --> 2
PID=16 (echo): syscall write --> 1
$
Your trace program should use new system call trace(int mask), which takes a bitmask as a
parameter. The bitmask indicates which system calls to trace. A bit value of ‘1’ in a position 2syscall_no
means that the system call with the number syscall_no is to be traced. If the bit value is ‘0’, the
system call is not to be traced. For example, to trace system call number 5 only, the user would
specify bit mask 25=32. To trace system calls 5 and 2, the user would specify the bit mask 25 + 22 =
32+4 = 36. To trace all system calls from 0 to 31, the user can specify bitmask 232-1 =
4294967295.
You will need to extend proc structure with a new field, which will keep the tracing bitmask. Do not
forget to copy the bitmask value from the parent proc structure to the child proc structure inside the
fork() system call.
The printing of the tracing message should be performed just before returning from system call to
the user process inside syscall() funciton. The format of the message is:
PID=pid ( name_of_the_process ): syscall name_of_syscall --> value_returned_from_syscall
Hints:
• add $U/_trace to UPROGS in Makefile
• create file trace.c in user/ subfolder of xv6-riscv
• add new syscall number in kernel/syscall.h and a matching entry in user/usys.pl, which will
automaticallt generate an assembly language function doing ecall in usys.S; consider
adding trace() function declaration to user/user.h
• write initial version of trace.c and make sure that it can run external programs with
parameters supplied on command line.
• Add new field to proc structure in kernel/proc.h to hold the tracing bitmask, and add
sys_trace() function in kernel/sysproc.c to save the value passed as a syscall parameter
into it.
• modify fork() in kernel/proc.c to copy the tracing bitmask from the parent to the newly
created child process.
Version 1.0, Page 5 of 7
PID=8 (grep): syscall read --> 1023
PID=8 (grep): syscall read --> 961
PID=8 (grep): syscall read --> 321
PID=8 (grep): syscall read --> 0
$
$ trace 4294967295 grep hello README
PID=12 (trace): syscall trace --> 0
PID=12 (grep): syscall exec --> 3
PID=12 (grep): syscall open --> 3
PID=12 (grep): syscall read --> 1023
PID=12 (grep): syscall read --> 961
PID=12 (grep): syscall read --> 321
PID=12 (grep): syscall read --> 0
PID=12 (grep): syscall close --> 0
$
$ trace 4294967295 echo hi
PID=16 (trace): syscall trace --> 0
PID=16 (echo): syscall exec --> 2
hiPID=16 (echo): syscall write --> 2
PID=16 (echo): syscall write --> 1
$
Your trace program should use new system call trace(int mask), which takes a bitmask as a
parameter. The bitmask indicates which system calls to trace. A bit value of ‘1’ in a position 2syscall_no
means that the system call with the number syscall_no is to be traced. If the bit value is ‘0’, the
system call is not to be traced. For example, to trace system call number 5 only, the user would
specify bit mask 25=32. To trace system calls 5 and 2, the user would specify the bit mask 25 + 22 =
32+4 = 36. To trace all system calls from 0 to 31, the user can specify bitmask 232-1 =
4294967295.
You will need to extend proc structure with a new field, which will keep the tracing bitmask. Do not
forget to copy the bitmask value from the parent proc structure to the child proc structure inside the
fork() system call.
The printing of the tracing message should be performed just before returning from system call to
the user process inside syscall() funciton. The format of the message is:
PID=pid ( name_of_the_process ): syscall name_of_syscall --> value_returned_from_syscall
Hints:
• add $U/_trace to UPROGS in Makefile
• create file trace.c in user/ subfolder of xv6-riscv
• add new syscall number in kernel/syscall.h and a matching entry in user/usys.pl, which will
automaticallt generate an assembly language function doing ecall in usys.S; consider
adding trace() function declaration to user/user.h
• write initial version of trace.c and make sure that it can run external programs with
parameters supplied on command line.
• Add new field to proc structure in kernel/proc.h to hold the tracing bitmask, and add
sys_trace() function in kernel/sysproc.c to save the value passed as a syscall parameter
into it.
• modify fork() in kernel/proc.c to copy the tracing bitmask from the parent to the newly
created child process.
Version 1.0, Page 5 of 7
I'VE FILLED IN A LOT OF THE CODE ALREADY HOWEVER, I'M STRUGGLING PRINTING IT OUT CORRECTLY: THIS IS THE CODE WE'RE GIVEN TO WORK WITH:
void
syscall(void)
{
intnum;
structproc *p = myproc();
num = p->trapframe->a7;
if(num > 0 && num < NELEM(syscalls) && syscalls[num]) {
// Use num to lookup the system call function for num, call it,
// and store its return value in p->trapframe->a0
p->trapframe->a0 = syscalls[num]();
} else {
printf("%d%s: unknown sys call %d\n",
p->pid, p->name, num);
p->trapframe->a0 = -1;
}
}
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 3 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
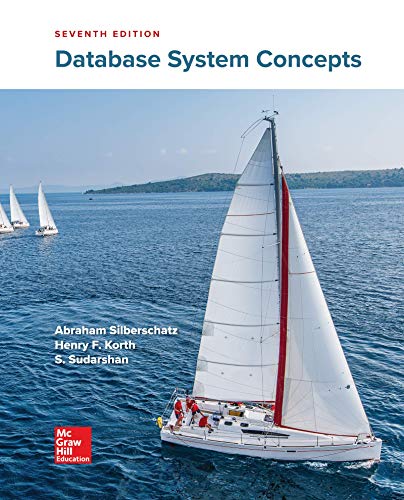
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
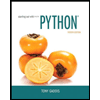
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
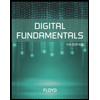
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
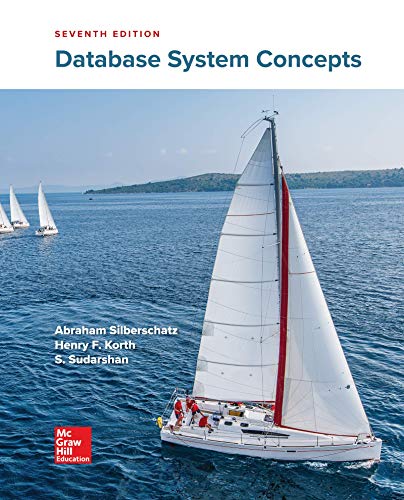
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
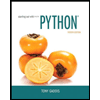
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
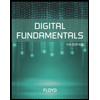
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
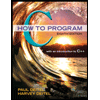
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
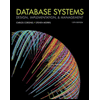
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
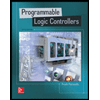
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education