Can someone please help me to correctly write the following program using
Can someone please help me to correctly write the following program using
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Can someone please help me to correctly write the following program using Java eclipse.

Transcribed Image Text:Part 3) Some basic stats -
The final part of the lab is concerned with the collection of a few basic stats, using counters.
(a) Modify the program to keep track of all objects input into the system into a counter (an
int variable). Increment the counter by one each time an object's name and mass is input.
(b) Modify the program to display the 'Total Input Count' at the end, after the user inputs -5
and before ending the program
(c) Modify the program again to have another counter, countValid (or something similar),
which is only incremented when the object's weight is accepted (between 1000 and 10).
Display this counter at the end as well
(d) Finally, modify the program to keep a running total of the weight in Newtons of all valid
objects. Objects that are too heavy or too light should not be included in this count.
(e) At the end, display the total valid weight as well as the average valid weight
(total/countValid). See Code Listing 4-13 for calculating the average after the while loop.
Sample Output at the end:
Enter the object's name>Honda Accord
Enter Honda Accord's mass>240
Honda Accord is too heavy.
Enter the object's name>Feather
Enter Feather's mass>0.001
Feather is too light.
Enter the object's name>Running Shoes
Enter Running Shoes's mass>2.5
Running Shoes weight is 24.5 Newtons
Enter the object's name>Kiwi
Enter Kiwi's mass>5.5
Kiwi weight is 53.9 Newtons
Enter the object's name>Panda
Enter Panda's mass>-5
Total input count: 4
Total valid count: 2
Total valid weight input: 78.4
Average valid weight: 39.2

Transcribed Image Text:Exercises
Part 1) Simple Input -
Mass and Weight. Scientists measure an object's mass in kilograms and its weight in Newtons.
Weight = Mass * 9.8
You should write code that does the following
(a) Create a scanner object for keyboard input and get an object's name from the user.
(b) Get the object's mass from the user. Display the object's name when asking for the
mass (See Part 2 for sample output). Note: Directly after calling nextDouble(), also
call nextLine(). Java console input can sometimes leave residual newlines, which
mess with future inputs. Please refer Code Listing 2-30 and 2-31 on pp 89-91.
(c) Calculate the weight with weight = mass * 9.8
(d) Write an if statement that checks the weight. If weight > 1000 Newtons, display
"objectName is too heavy!" (Where object name is the name input at step a). If the
object weighs less than 10, display "objectName is too light!" Otherwise, display
"objectName weighs X", where X is the weight from part c. Use String.format in
Section 3.10 to have it display only one decimal point.
(e) As always, output can be slightly different so long as the weight and objectName are
displayed.
(f) Verify that your code works for all three cases before moving on
Part 2) Basic Loop -
This part extends the code from Part 1 into a loop.
(a) Take the code from part 1 and put it into a while loop. The while loop should run
until the user inputs a -5, at which point the while loop should end. In this case, the
input is a 'sentinel value'. Note: you will have to get the first name and first mass
outside of the loop for things to work how I intend it to. See Code Listing 4-11.
(b) The input that ends the loop should be the object's mass, not the object's name, to
prevent the need to convert data types
Sample Output after Part 2:
Enter the object's name> Honda Accord
Enter Honda Accord's mass> 240
Honda Accord is too heavy.
Enter the object's name> Feather
Enter Feather's mass> 0.001
Feather is too light.
Enter the object's name> Running Shoes
Enter Running Shoes's mass> 2.5
Running Shoes's weight is 24.5 Newtons.
Enter the object's name> Panda
Enter Panda's mass>-5
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
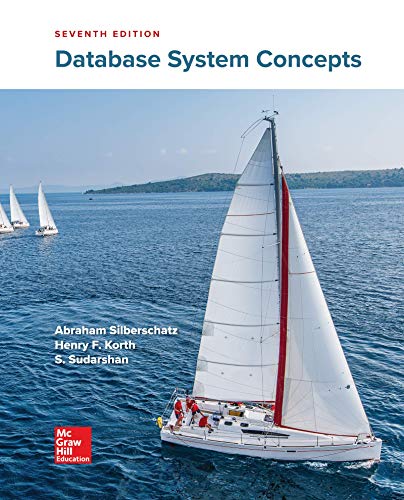
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
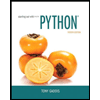
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
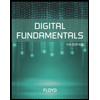
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
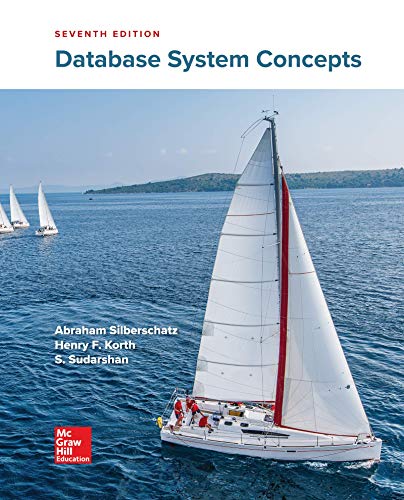
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
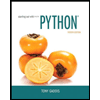
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
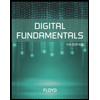
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
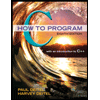
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
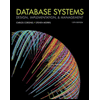
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
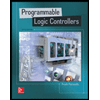
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education