Problem Description and Given Info For this assignment you are given the following Java source code files: • MyListIterator.java (This file is complete - make no changes to this file) • MyList.java (This file is complete - make no changes to this file) • MyArrayList.java (You must complete this file) • Main.java (You may use this file to write code to test your MyArrayList)
Problem Description and Given Info For this assignment you are given the following Java source code files: • MyListIterator.java (This file is complete - make no changes to this file) • MyList.java (This file is complete - make no changes to this file) • MyArrayList.java (You must complete this file) • Main.java (You may use this file to write code to test your MyArrayList)
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Please help with the following code in Java!
![Problem Description and Given Info
For this assignment you are given the following Java source code files:
• MyListIterator.java (This file is complete - make no changes to this file)
.
• MyList.java (This file is complete - make no changes to this file)
.
• MyArrayList.java (You must complete this file)
• Main.java (You may use this file to write code to test your MyArrayList)
You must complete the public class named MyArrayList with fields and methods as defined below. Your MyArrayList will
implement the MyList interface that is provided in the myList.java file.
MyListIterator <<Interface>>
+ hasNext(): boolean
+ next(): Object
↑
I
MyList<<interface>>
+ append(item: Object) : void
+insertAtfindex: int, item: Obiect); void
+removeAt(index:int):void
+ getAt(Indes: Int): Object
+ getSize(): int
+ getiterator(): MyListIterator
implements
implements
MyArrayList
-currentCapacity: int
-size: int
-storage: Object[]
+makeCapacity(minCapacity: int) : void
+ trimExcess():void
MyArrayListiterator
UML CLass Diagram: MyArrayList
Structure of the Fields
As described by the UML Class Diagram above, your MyArrayList class must have the following fields:
• a private field named current Capacity of type int, initialized to 8
• a private field named size of type int, initialized to 0
• a private field named storage of type Object[], initialized to an object array of 8 elements
Structure of the Methods
As described by the UML Class Diagram above, your MyArrayList class must have the following methods:
• a public method named append that takes an object argument and returns nothing
.
• a public method named insertAt that takes an int argument and an object argument and returns nothing
• a public method named removeAt that takes an int arguments and returns nothing
3. trimExcess method
. a public method named getAt that takes an int argument and returns an object
a publ: method named getsize that takes no arguments and returns an int
. a public method named makeCapacity that takes an int argument and returns noting
. a public method named trimExcess that takes no arguments and returns nothing
Note that five of these methods are declared in the MyList interface. You will be implementing these methods in this MyArrayList
concrete derived class.
Also note that the getIterator method and the MyArrayListIterator class are already implemented for you in the MyArrayList
class. Make no changes to this code.
Additional Information
MyArrayList
1. This concrete class will store its elements in an array of object. The initial capacity of this array will be 8 elements. Since an array is
a fixed size structure, you may need to allocate a new array with increased capacity in order to accommodate adding new elements.
For this purpose you must implement the makeCapacity method.
2. makeCapacity method
• This method will take a minCapacity as an int argument.
If minCapacity is less than current size or equal to the current Capacity, then this method should take no action.
• Otherwise the capacity of this MyArrayList must be changed to either 8 or minCapacity (whichever is greater).
• If current Capacity is to be changed, then this method will allocate a new array of Object sized to the new capacity
Then copy over all elements from the old array to the new array
. Then store the new array in the private storage variable for this instance
• This method will remove any excess capacity by simply calling the makeCapacity method with an argument value that is
equal to the current size of this list.
4. append method
• Appends new item to end of list. For example: given the list {1, 2, 3) and an instruction to append (99), the result would be
this (1, 2, 3, 99).
. If the current size is equal to the current capacity, then this list is full to its current capacity, and capacity will need to be
increased before we can append the new element. To increase the capacity, call the makeCapacity method with an argument
value that is twice the current capacity.
• This method will add the new element to the list at the next available index in the array storage.
5. insertAt method
• Makes a place at the specified index by moving all items at this index and beyond to the next larger index. For example: given
the list (1, 2, 3) and an instruction to insertAt (1, 99), the result would be this (1, 99, 2, 3).
• Throws a NoSuchElement Exception if the specified index is less than 0 or greater than size.
• If the current size is equal to the current capacity, then this list is full to its current capacity, and capacity will need to be
increased before we can insert the new element. To increase the capacity, call the makeCapacity method with an argument
value that is twice the current capacity.
6. removeAt method
• Removes the element at the specified index and moves all elements beyond that index to the next lower index. For example:
given the list {1, 2, 3) and an instruction to removeAt (1), the result would be this {1, 3).
• Throws a NoSuchElementException if the specified index is less than 0 or greater than or equal to size.
7. getAt method
• Returns the item at the specified index. For example: given the list (1, 2, 3) and an instruction to getAt (1), the return value
would be 2.
• Throws a NoSuchElementException if the specified index is less than 0 or greater than or equal to size.
8. getSize method
• Returns the number of elements currently stored in the list.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F1e26a685-55e2-4925-ba70-9f39a5b1116e%2Ff44666f2-bf99-4693-9d0b-89da0616104e%2Fpgq2lsh_processed.png&w=3840&q=75)
Transcribed Image Text:Problem Description and Given Info
For this assignment you are given the following Java source code files:
• MyListIterator.java (This file is complete - make no changes to this file)
.
• MyList.java (This file is complete - make no changes to this file)
.
• MyArrayList.java (You must complete this file)
• Main.java (You may use this file to write code to test your MyArrayList)
You must complete the public class named MyArrayList with fields and methods as defined below. Your MyArrayList will
implement the MyList interface that is provided in the myList.java file.
MyListIterator <<Interface>>
+ hasNext(): boolean
+ next(): Object
↑
I
MyList<<interface>>
+ append(item: Object) : void
+insertAtfindex: int, item: Obiect); void
+removeAt(index:int):void
+ getAt(Indes: Int): Object
+ getSize(): int
+ getiterator(): MyListIterator
implements
implements
MyArrayList
-currentCapacity: int
-size: int
-storage: Object[]
+makeCapacity(minCapacity: int) : void
+ trimExcess():void
MyArrayListiterator
UML CLass Diagram: MyArrayList
Structure of the Fields
As described by the UML Class Diagram above, your MyArrayList class must have the following fields:
• a private field named current Capacity of type int, initialized to 8
• a private field named size of type int, initialized to 0
• a private field named storage of type Object[], initialized to an object array of 8 elements
Structure of the Methods
As described by the UML Class Diagram above, your MyArrayList class must have the following methods:
• a public method named append that takes an object argument and returns nothing
.
• a public method named insertAt that takes an int argument and an object argument and returns nothing
• a public method named removeAt that takes an int arguments and returns nothing
3. trimExcess method
. a public method named getAt that takes an int argument and returns an object
a publ: method named getsize that takes no arguments and returns an int
. a public method named makeCapacity that takes an int argument and returns noting
. a public method named trimExcess that takes no arguments and returns nothing
Note that five of these methods are declared in the MyList interface. You will be implementing these methods in this MyArrayList
concrete derived class.
Also note that the getIterator method and the MyArrayListIterator class are already implemented for you in the MyArrayList
class. Make no changes to this code.
Additional Information
MyArrayList
1. This concrete class will store its elements in an array of object. The initial capacity of this array will be 8 elements. Since an array is
a fixed size structure, you may need to allocate a new array with increased capacity in order to accommodate adding new elements.
For this purpose you must implement the makeCapacity method.
2. makeCapacity method
• This method will take a minCapacity as an int argument.
If minCapacity is less than current size or equal to the current Capacity, then this method should take no action.
• Otherwise the capacity of this MyArrayList must be changed to either 8 or minCapacity (whichever is greater).
• If current Capacity is to be changed, then this method will allocate a new array of Object sized to the new capacity
Then copy over all elements from the old array to the new array
. Then store the new array in the private storage variable for this instance
• This method will remove any excess capacity by simply calling the makeCapacity method with an argument value that is
equal to the current size of this list.
4. append method
• Appends new item to end of list. For example: given the list {1, 2, 3) and an instruction to append (99), the result would be
this (1, 2, 3, 99).
. If the current size is equal to the current capacity, then this list is full to its current capacity, and capacity will need to be
increased before we can append the new element. To increase the capacity, call the makeCapacity method with an argument
value that is twice the current capacity.
• This method will add the new element to the list at the next available index in the array storage.
5. insertAt method
• Makes a place at the specified index by moving all items at this index and beyond to the next larger index. For example: given
the list (1, 2, 3) and an instruction to insertAt (1, 99), the result would be this (1, 99, 2, 3).
• Throws a NoSuchElement Exception if the specified index is less than 0 or greater than size.
• If the current size is equal to the current capacity, then this list is full to its current capacity, and capacity will need to be
increased before we can insert the new element. To increase the capacity, call the makeCapacity method with an argument
value that is twice the current capacity.
6. removeAt method
• Removes the element at the specified index and moves all elements beyond that index to the next lower index. For example:
given the list {1, 2, 3) and an instruction to removeAt (1), the result would be this {1, 3).
• Throws a NoSuchElementException if the specified index is less than 0 or greater than or equal to size.
7. getAt method
• Returns the item at the specified index. For example: given the list (1, 2, 3) and an instruction to getAt (1), the return value
would be 2.
• Throws a NoSuchElementException if the specified index is less than 0 or greater than or equal to size.
8. getSize method
• Returns the number of elements currently stored in the list.
![Current file: MyArrayList.java
1 // Complete the implementation of your My MyArrayList.java
2
MyList.java
3
4 public class MyArrayList implements MyList
5
private int capacity = 16;
6
7 private Object[] array = new Object [cape Main.java
8
9 private int size = 0;
10
11 @Override
12
13 public void add(Object o) {
14
15 if (size >= capacity) {
16
MyListiterator.java
is file
Load default template.....](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F1e26a685-55e2-4925-ba70-9f39a5b1116e%2Ff44666f2-bf99-4693-9d0b-89da0616104e%2Fgkdgt2_processed.png&w=3840&q=75)
Transcribed Image Text:Current file: MyArrayList.java
1 // Complete the implementation of your My MyArrayList.java
2
MyList.java
3
4 public class MyArrayList implements MyList
5
private int capacity = 16;
6
7 private Object[] array = new Object [cape Main.java
8
9 private int size = 0;
10
11 @Override
12
13 public void add(Object o) {
14
15 if (size >= capacity) {
16
MyListiterator.java
is file
Load default template.....
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

Follow-up Questions
Read through expert solutions to related follow-up questions below.
Follow-up Question
I tried this and I get the following errors:
4 errors
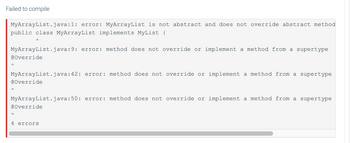
Transcribed Image Text:Failed to compile
MyArrayList.java:1: error: MyArrayList is not abstract and does not override abstract method
public class MyArrayList implements MyList {
MyArrayList.java:9: error: method does not override or implement a method from a supertype
@Override
A
MyArrayList.java:42: error: method does not override or implement a method from a supertype
@Override
^
MyArrayList.java:50: error: method does not override or implement a method from a supertype
@Override
4 errors
Solution
Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
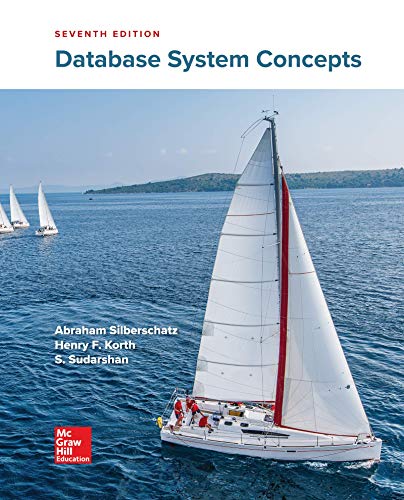
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
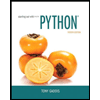
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
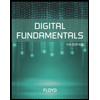
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
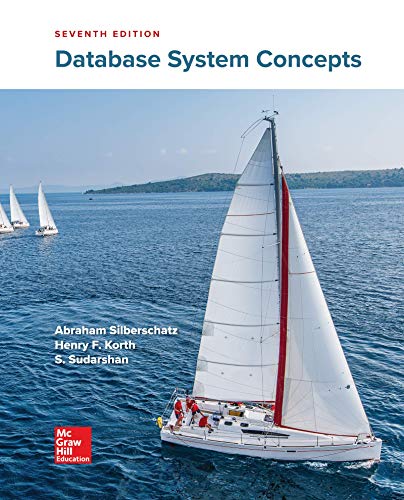
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
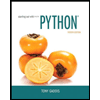
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
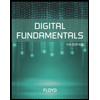
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
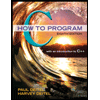
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
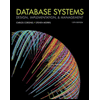
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
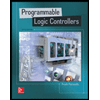
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education