Can Someone help me fix this error the code is written in java... import java.util.*; class HPF { int at, bt, pri, pno; Process(int pno, int at, int bt, int pri) { this.pno = pno; this.pri = pri; this.at = at; this.bt = bt; } } class GChart { int pno, stime, ctime, wtime, ttime; } class MyComparator implements Comparator { public int compare(Object o1, Object o2) { Process p1 = (Process)o1; Process p2 = (Process)o2; if (p1.at < p2.at) return (-1); else if (p1.at == p2.at && p1.pri > p2.pri) return (-1); else return (1); } } class FindGantChart { void findGc(LinkedList queue) { int time = 0; TreeSet prique = new TreeSet(new MyComparator()); LinkedList result = new LinkedList(); while (queue.size() > 0) prique.add((Process)queue.removeFirst()); Iterator it = prique.iterator(); time = ((Process)prique.first()).at; while (it.hasNext()) { Process obj = (Process)it.next(); GChart gc1 = new GChart(); gc1.pno = obj.pno; gc1.stime = time; time += obj.bt; gc1.ctime = time; gc1.ttime = gc1.ctime - obj.at; gc1.wtime = gc1.ttime - obj.bt; result.add(gc1); } new ResultOutput(result); } }
Can Someone help me fix this error the code is written in java...
import java.util.*;
class HPF {
int at, bt, pri, pno;
Process(int pno, int at, int bt, int pri)
{
this.pno = pno;
this.pri = pri;
this.at = at;
this.bt = bt;
}
}
class GChart {
int pno, stime, ctime, wtime, ttime;
}
class MyComparator implements Comparator {
public int compare(Object o1, Object o2)
{
Process p1 = (Process)o1;
Process p2 = (Process)o2;
if (p1.at < p2.at)
return (-1);
else if (p1.at == p2.at && p1.pri > p2.pri)
return (-1);
else
return (1);
}
}
class FindGantChart {
void findGc(LinkedList queue)
{
int time = 0;
TreeSet prique = new TreeSet(new MyComparator());
LinkedList result = new LinkedList();
while (queue.size() > 0)
prique.add((Process)queue.removeFirst());
Iterator it = prique.iterator();
time = ((Process)prique.first()).at;
while (it.hasNext()) {
Process obj = (Process)it.next();
GChart gc1 = new GChart();
gc1.pno = obj.pno;
gc1.stime = time;
time += obj.bt;
gc1.ctime = time;
gc1.ttime = gc1.ctime - obj.at;
gc1.wtime = gc1.ttime - obj.bt;
result.add(gc1);
}
new ResultOutput(result);
}
}


Trending now
This is a popular solution!
Step by step
Solved in 2 steps

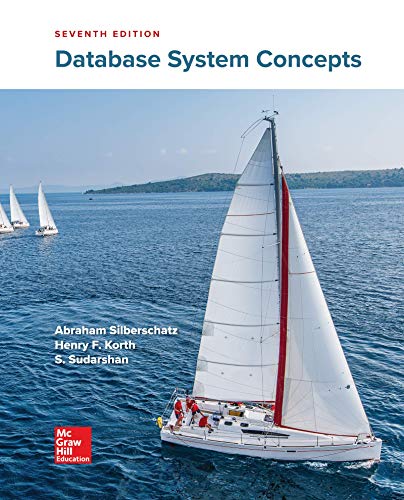
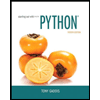
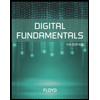
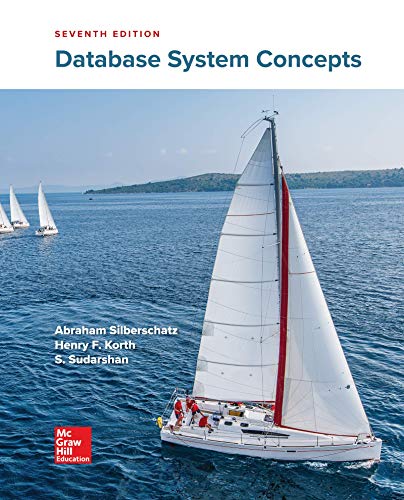
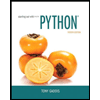
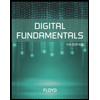
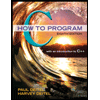
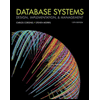
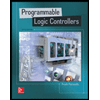