Can anyone convert this c++ code to python? //THIS PROGRAM IS CREATED TO DEMONSTRATE THE OPERATIONS PERFORMED ON STACK & ITS IMPLEMENTATION USING ARRAYS #include #include #include #include #include //Defining the maximum size of the stack #define MAXSIZE 7 using namespace std; //A class initialized with public and private variables and functions class STACK_ARRAY { int stack[MAXSIZE]; int Top; public: //constructor is called and Top pointer is initialized to -1 when an object is created for the class STACK_ARRAY() { Top = -1; } void push(); void pop(); void traverse(); }; //This function will add/insert an element to Top of the stack void STACK_ARRAY::push() { int item; //if the top pointer already reached the maximum allowed size then we can say that the stack is full or overflow if (Top == MAXSIZE-1) { cout << "\nThe Stack Is Full"; } //Otherwise an element can be added or inserted by incrementing the stack pointer Top as follows else { cout << "\nEnter The Element (number) To Be Inserted: "; cin >> item; stack[++Top] = item; } } //This function will delete an element from the Top of the stack void STACK_ARRAY::pop() { int item; //If the Top pointer points to NULL, then the stack is empty. That is NO element is there to delete or pop if (Top == -1) cout << "\nThe Stack Is Empty"; //Otherwise the top most element in the stack is popped or deleted by decrementing the Top pointer else { item = stack[Top--]; cout << "\nThe Deleted Element (number) Is: " << item; } } //This function to print all the existing elements in the stack void STACK_ARRAY::traverse(){ int i; //If the Top pointer points to NULL, then the stack is empty. That is NO element is there to delete or pop if (Top == -1) cout << "\nThe Stack is Empty"; //Otherwise all the elements in the stack is printed else { cout << "\nThe Element(s) in the Stack(s) is/are: "; for(i = Top; i >= 0; i--) cout << stack[i] << " "; cout << "\n"; } } int main() { int choice; char ch; //Declaring an object to the class STACK_ARRAY ps; do { //A menu for the stack operations cout << "MAIN MENU\n\n"; cout << "STACK using Array \n\n"; cout << "[1] PUSH (Add number)\n"; cout << "[2] POP (Delete number)\n"; cout << "[3] TRAVERSE (Display number)\n"; cout << "[4] QUIT\n\n"; cout << "Enter Your Choice: "; cin >> choice; switch(choice) { case 1://Calling push() function by class object ps.push(); break; case 2://calling pop() function ps.pop(); break; case 3://calling traverse() function ps.traverse(); break; case 4: exit(0); default: cout << "\nYou Entered wrong Choice"; } cout << "\n\nPress (Y/y) To Continue... "; cin >> ch; cout << "\n"; } while(ch == 'Y' || ch == 'y'); } And the out put should look like in the picture below.
Can anyone convert this c++ code to python?
//THIS PROGRAM IS CREATED TO DEMONSTRATE THE OPERATIONS PERFORMED ON STACK & ITS IMPLEMENTATION USING ARRAYS
#include <iostream>
#include <stdio.h>
#include <conio.h>
#include <stdlib.h>
#include <process.h>
//Defining the maximum size of the stack
#define MAXSIZE 7
using namespace std;
//A class initialized with public and private variables and functions
class STACK_ARRAY
{
int stack[MAXSIZE];
int Top;
public:
//constructor is called and Top pointer is initialized to -1 when an object is created for the class
STACK_ARRAY()
{
Top = -1;
}
void push();
void pop();
void traverse();
};
//This function will add/insert an element to Top of the stack
void STACK_ARRAY::push()
{
int item;
//if the top pointer already reached the maximum allowed size then we can say that the stack is full or overflow
if (Top == MAXSIZE-1)
{
cout << "\nThe Stack Is Full";
}
//Otherwise an element can be added or inserted by incrementing the stack
pointer Top as follows
else
{
cout << "\nEnter The Element (number) To Be Inserted: "; cin >> item;
stack[++Top] = item;
}
}
//This function will delete an element from the Top of the stack
void STACK_ARRAY::pop()
{
int item;
//If the Top pointer points to NULL, then the stack is empty. That is NO element is there to delete or pop
if (Top == -1)
cout << "\nThe Stack Is Empty";
//Otherwise the top most element in the stack is popped or deleted by decrementing the Top pointer
else
{
item = stack[Top--];
cout << "\nThe Deleted Element (number) Is: " << item;
}
}
//This function to print all the existing elements in the stack
void STACK_ARRAY::traverse(){
int i;
//If the Top pointer points to NULL, then the stack is empty. That is NO element is there to delete or pop
if (Top == -1)
cout << "\nThe Stack is Empty";
//Otherwise all the elements in the stack is printed
else
{
cout << "\nThe Element(s) in the Stack(s) is/are: ";
for(i = Top; i >= 0; i--)
cout << stack[i] << " ";
cout << "\n";
}
}
int main()
{
int choice;
char ch;
//Declaring an object to the class
STACK_ARRAY ps;
do
{
//A menu for the stack operations
cout << "MAIN MENU\n\n";
cout << "STACK using Array \n\n";
cout << "[1] PUSH (Add number)\n";
cout << "[2] POP (Delete number)\n";
cout << "[3] TRAVERSE (Display number)\n";
cout << "[4] QUIT\n\n";
cout << "Enter Your Choice: "; cin >> choice;
switch(choice)
{
case 1://Calling push() function by class object
ps.push();
break;
case 2://calling pop() function
ps.pop();
break;
case 3://calling traverse() function
ps.traverse();
break;
case 4:
exit(0);
default:
cout << "\nYou Entered wrong Choice";
}
cout << "\n\nPress (Y/y) To Continue... ";
cin >> ch;
cout << "\n";
} while(ch == 'Y' || ch == 'y');
}
And the out put should look like in the picture below.
![eskton
D:\c++\practice1\bin\Debug\practice1.exe
ΜΑIΝ ΜΕΝU
STACK using Array
[1] PUSH (Add number)
[2] POP (Delete number)
[3] TRAVERSE (Display number)
[4] QUIT
Enter Your Choice:](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F9ff678a3-f2b9-4e6b-abff-dae357262e3c%2Fb93e6bbe-0d50-49b8-bb1b-72ad9caf8151%2Frng0sgp_processed.png&w=3840&q=75)

Step by step
Solved in 3 steps with 2 images

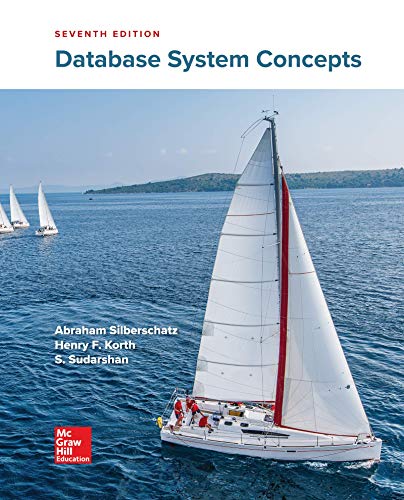
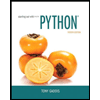
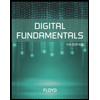
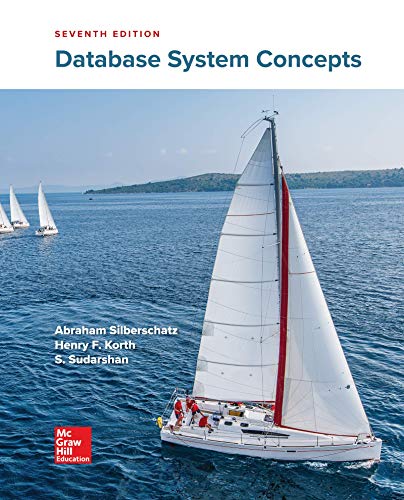
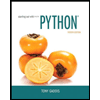
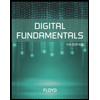
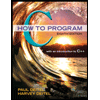
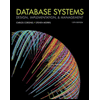
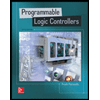