calculating the average school grade which is an integer. The current code doesn't calculate the average grade correctly. Also when I enter 0 to exit it get an error (please run code on your end to find the issues with calculating the average grade and error when enter 0).
Control structures
Control structures are block of statements that analyze the value of variables and determine the flow of execution based on those values. When a program is running, the CPU executes the code line by line. After sometime, the program reaches the point where it has to make a decision on whether it has to go to another part of the code or repeat execution of certain part of the code. These results affect the flow of the program's code and these are called control structures.
Switch Statement
The switch statement is a key feature that is used by the programmers a lot in the world of programming and coding, as well as in information technology in general. The switch statement is a selection control mechanism that allows the variable value to change the order of the individual statements in the software execution via search.
need help finishing Python code. I need help calculating the average school grade which is an integer. The current code doesn't calculate the average grade correctly. Also when I enter 0 to exit it get an error (please run code on your end to find the issues with calculating the average grade and error when enter 0).
CODE
import decimal
from decimal import Decimal
class GradeBook:
count = 0
def __init__(self, name, grades=[]):
self.name = name
GradeBook.count += 1
print("There are",GradeBook.count,"students in the GradeBook")
self.grades = []
def quizScore(self,score):
self.grades.append(score)
def currentAverage(self):
sum = 0
for i in self.grades:
sum += i
avg = sum/len(self.grades)
return avg
name1 = input("Please enter the name for Student 1:")
Student1 = GradeBook(name1)
name2 = input("Please enter the name for Student 2:")
Student2 = GradeBook(name2)
menu = """
Grade Book
0: Exit
1: Enter quiz grade for Student 1
2: Enter quiz grade for Student 2
3: Display current grades for all students
"""
done = False
while not done:
print(menu)
selection = input('Please enter a choice: ')
if selection == "0":
done = True
print('Exiting Grade Book Now!')
if selection == '1':
print('Student 1')
grade = float(input("Enter the grade for the test: "))
Student1.quizScore(grade)
elif selection == '2':
print('Student 2')
grade = float(input("Enter the grade for the test: "))
Student2.quizScore(grade)
elif selection == '3':
print("Current grades for Students")
print("Name:",Student1.name)
print("Current Average:",Student1.currentAverage())
print("Name:",Student2.name)
print("Current Average:",Student2.currentAverage())
Score(" ", [])
obj.quizScore([])
obj.display()
OUTPUT OF CODE
Please enter the name for Student 1:
bob
There are 1 students in the GradeBook
Please enter the name for Student 2:
tom
There are 2 students in the GradeBook
Grade Book
0: Exit
1: Enter quiz grade for Student 1
2: Enter quiz grade for Student 2
3: Display current grades for all students
Please enter a choice:
1
Student 1
Enter the grade for the test:
33
Grade Book
0: Exit
1: Enter quiz grade for Student 1
2: Enter quiz grade for Student 2
3: Display current grades for all students
Please enter a choice:
1
Student 1
Enter the grade for the test:
22
Grade Book
0: Exit
1: Enter quiz grade for Student 1
2: Enter quiz grade for Student 2
3: Display current grades for all students
Please enter a choice:
3
Current grades for Students
Name: bob
Current Average: 16.5
Name: tom
Current Average: None
Grade Book
0: Exit
1: Enter quiz grade for Student 1
2: Enter quiz grade for Student 2
3: Display current grades for all students
Please enter a choice: ** Process Stopped **
Press Enter to exit terminal

Built in Sum () is used to sum up the individual list items.
To avoid divide by zero error in case of blank grades list for any of the students, length of the list becomes 1 and as sum gives 0, it returns None as in the sample output.
Selection Choice =0 , works fine. No change required.
Importing Decimal not needed as no use of it.
Step by step
Solved in 3 steps with 1 images

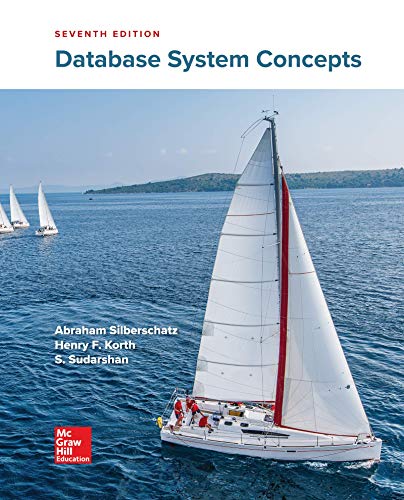
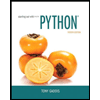
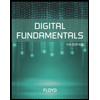
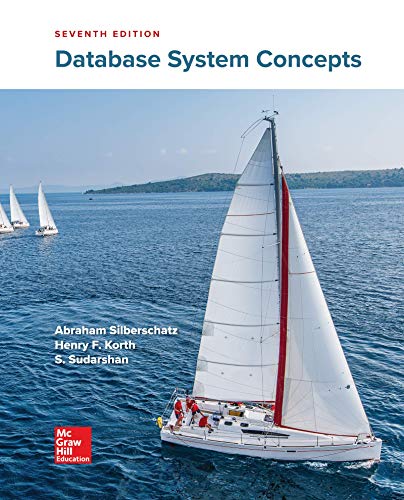
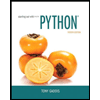
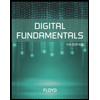
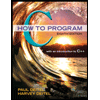
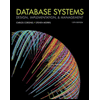
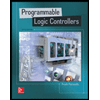