C++ Write a program that outputs a downwards facing arrow composed of a rectangle and a right triangle. Arrow dimensions are defined by user specified arrow base height, arrow base width, and arrow head width. Input the arrow base height (int) and width (int). Draw a rectangle using asterisks (height x width). Hint: use a nested loop in which the inner loop draws one row of *s, and the outer loop iterates a number of times equal to the height. Submit for grading to confirm two tests pass. Ex: If input is: 6 4 Sample output is: **** **** **** **** **** ****
C++
Write a program that outputs a downwards facing arrow composed of a rectangle and a right triangle. Arrow dimensions are defined by user specified arrow base height, arrow base width, and arrow head width.
Input the arrow base height (int) and width (int). Draw a rectangle using asterisks (height x width). Hint: use a nested loop in which the inner loop draws one row of *s, and the outer loop iterates a number of times equal to the height. Submit for grading to confirm two tests pass.
Ex: If input is:
6 4
Sample output is:
****
****
****
****
****
****

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

C++ continued
you already gave me the help from the first part. now they have added another set of instructions.
original code used.
Here I have created the main method.
Inside the main method, I have taken input from the user for height and width and stored them into different variables.
Next, I have created a loop that runs till it reaches the height of the rectangle.
Inside the loop, I have created another loop that runs till the width of the rectangle.
In the inner loop, I have printed the asterisk symbol.
After the inner loop, I have used endl to give break and move the pointer to the new line.
C++ code:
#include<iostream>
using namespace std;
int main()
{
int height, width;
// user input
cin >> height >> width;
// outer loop
for(int i = 0; i < height; i++)
{
// inner loop
for(int j = 0; j < width; j++)
{
//printing the * symbol
cout << "*";
}
//changing the line
cout << endl;
}
}
Now Input the arrow head width and draw a right triangle. Hint: use a nested loop.
input: 4 3 4
Finally, Modify the program to only accept an arrow head width that is larger than the arrow base width. Use a loop to continue inputting the arrow head width until the value is larger than the arrow base width.
for the second input: 4 3 3 2 4
final output:
***
***
***
***
****
***
**
*
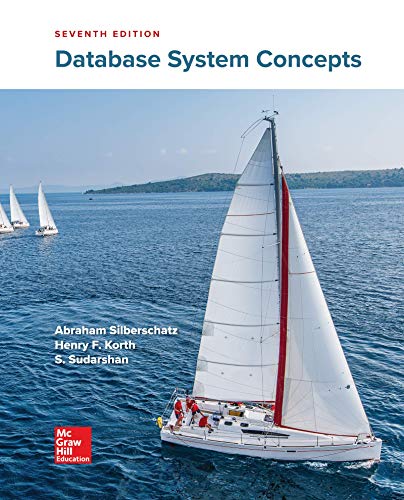
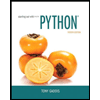
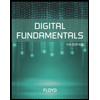
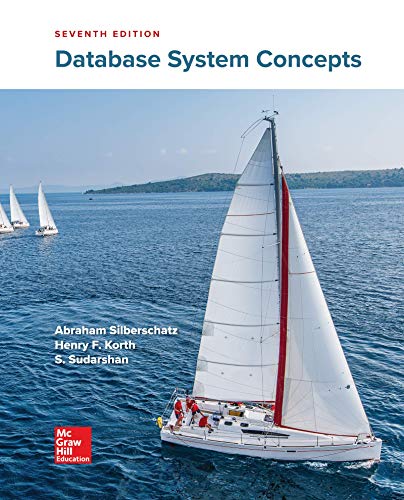
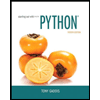
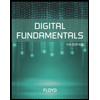
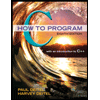
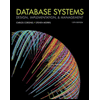
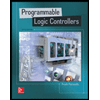