C++ program. On line 30, the reference variables are getting errors because there's an expected primary expression but I'm not sure what they are. What's underlined below are the errors. ReadRoomData(int &room_num, int &max_cap, int &enrolled); Text files: roomset_one.dat 426 25 25 327 18 14 420 20 15 317 100 101 −1 −1 −1 roomset_two.dat 55 10 5 102 12 10 111 15 16 250 20 25 4033 500 207 7810 800 800 8810 800 0 -2 -2 -2 roomset_three.dat -999 Program Requirements DO NOT open the file and use it within your program (input redirection) Example of input redirection: ./a.out < roomset_one.dat You must include the following functions: ReadRoomData: a void function that accepts three reference parameters (the room number, max capacity, and enrolled). It should read in all three values from stdin. DetermineStatus: a value-returning function that has one parameter (available enrollment). It should return a std::string containing the status of the room (OPEN, FULL, OVER) OutputRoomInfo: a void function that accepts three value parameters (room, max capacity, and enrolled). It should call DetermineStatus and output the formatted room information for that single set of data.
C++ program. On line 30, the reference variables are getting errors because there's an expected primary expression but I'm not sure what they are. What's underlined below are the errors. ReadRoomData(int &room_num, int &max_cap, int &enrolled); Text files: roomset_one.dat 426 25 25 327 18 14 420 20 15 317 100 101 −1 −1 −1 roomset_two.dat 55 10 5 102 12 10 111 15 16 250 20 25 4033 500 207 7810 800 800 8810 800 0 -2 -2 -2 roomset_three.dat -999 Program Requirements DO NOT open the file and use it within your program (input redirection) Example of input redirection: ./a.out < roomset_one.dat You must include the following functions: ReadRoomData: a void function that accepts three reference parameters (the room number, max capacity, and enrolled). It should read in all three values from stdin. DetermineStatus: a value-returning function that has one parameter (available enrollment). It should return a std::string containing the status of the room (OPEN, FULL, OVER) OutputRoomInfo: a void function that accepts three value parameters (room, max capacity, and enrolled). It should call DetermineStatus and output the formatted room information for that single set of data.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Hello I need help in a C++ program. On line 30, the reference variables are getting errors because there's an expected primary expression but I'm not sure what they are. What's underlined below are the errors.
ReadRoomData(int &room_num, int &max_cap, int &enrolled);
Text files:
- roomset_one.dat
- 426 25 25
327 18 14
420 20 15
317 100 101
−1 −1 −1
- 426 25 25
- roomset_two.dat
- 55 10 5
102 12 10
111 15 16
250 20 25
4033 500 207
7810 800 800
8810 800 0
-2 -2 -2
- 55 10 5
- roomset_three.dat
- -999
Program Requirements
-
DO NOT open the file and use it within your program (input redirection)
- Example of input redirection: ./a.out < roomset_one.dat
-
You must include the following functions:
- ReadRoomData: a void function that accepts three reference parameters (the room number, max capacity, and enrolled). It should read in all three values from stdin.
- DetermineStatus: a value-returning function that has one parameter (available enrollment). It should return a std::string containing the status of the room (OPEN, FULL, OVER)
- OutputRoomInfo: a void function that accepts three value parameters (room, max capacity, and enrolled). It should call DetermineStatus and output the formatted room information for that single set of data.

Transcribed Image Text:4 #include <iostream>>
3 #include <fstream>
0 #include <string>
7 #include <iomanip>
9
8 void ReadRoomData( int &, int &, int &);
void OutputRoomInfo(int, int, int);
10 std::string DetermineStatus(int
int main() {
11
12
std::string filename;
//let's create an fstream variable
std::ofstream output_file;
13
14
15
259287N
10
17
18 int total_max_cap = 0;
19
20
21
23
24
33
34
35
30
37
38
39
std::cout << std::setw(8) << std::left << "Room";
25 std::cout <<std::setw(8) << "Capacity";
20 std::cout <<std::setw(10) << std::left << "Enrolled";
41
42
43
44
int room_num;
int max_cap;
int enrolled;
27 std::cout <<std::setw(13) << std::left << "Empty Seats";
28 std::cout <<std::setw(9) << std::left << "Statues" << std::endl;
29
30
31
32
45
40
47
48
int total_room = 0;
int total enroll = 0;
33
30
int total_over_enro = 0;
int total_open_enroll = 0;
}
while (true){
ReadRoomData(int &room_num, int Smax_cap, int Senrolled);
if (room_num < 0){ //return "OPEN";
total_room++;
total_max_cap += max_cap;
total_enroll += enrolled;
}
empty_seats);
}
else if (max_cap - enrolled > 0) {|
total_open_enroll++;
}
else if(max_cap enrolled < 0){
total_over_enroll++;
OutputRoomInfo(room_num, max_cap, enrolled);
}
std::cout << ***************
std::cout << "Rooms: << total_room << std::endl;
std::cout << "Overall Capacity: << total_max_cap << std::endl;
std::cout << "Total Enrollment: << total_enroll << std::endl;
std::cout << "Number of OVER Enrolled Rooms: " << total_over_enroll << std::endl;
std::cout<<"Number of OPEN Rooms Available: << std::endl;
std::cout << *****************
************************";
49
50
51
}
32 void ReadRoomData(double &room_num, double &max_cap, double enrolled) {
53
54
std::cin>> room_num;
std::cin>> max_cap;
std::cin>>enrolled;
**************
///check if they need to be before or after brackets

Transcribed Image Text:51 }
32 void ReadRoomData(double &room_num, double &max_cap, double enrolled) {
53
54
55
30
57
38
59
00
01
02
03
64
73 v
74
}
std::cin>> room_num;
std::cin>> max_cap;
std::cin>>enrolled;
}
void OutputRoomInfo(int room, int max_cap, int enrolled){
int empty_seats = max_cap enrolled; //max.capacity - enrolled = empty seats
//int empty_seats = Determinestatus (max_cap - enrolled);
std::string status = Determinestatus (empty_seats);
std::cout << std::setw(8) <<< std::left << room;
std::cout<<std::setw(10) << std::right <<max_cap;
std::cout << std::setw(8) << std::right << enrolled;
std::cout << std::setw(11) << std::right << DetermineStatus (max_cap enrolled) << std::endl;
75
70 }
65
00 std::string Determinestaus(int empty_seats) {
07 - if(empty_seats > 0) {
08
return "OPEN";
09
70 -
71
72
///check if they need to be before or after brackets
}
else if (empty_seats == 0){
return "FULL";
}
else{
}
return "OVER";
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 4 steps with 5 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
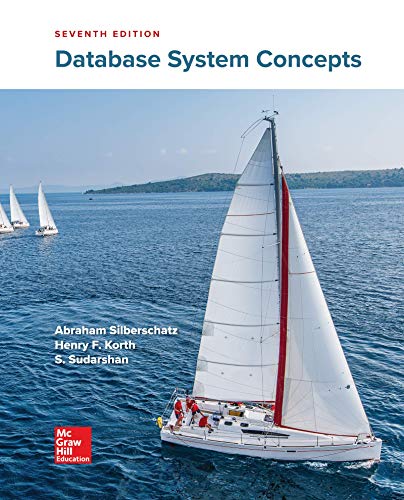
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
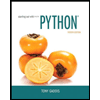
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
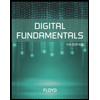
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
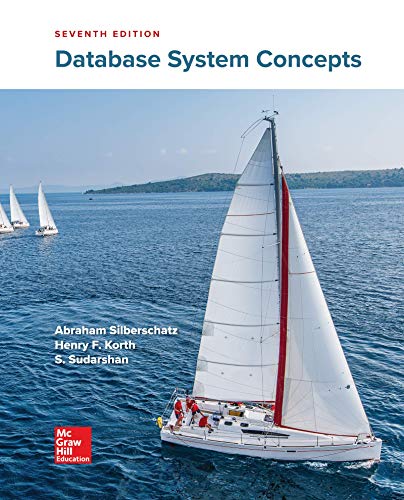
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
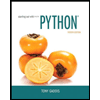
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
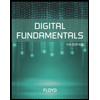
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
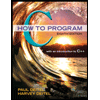
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
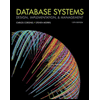
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
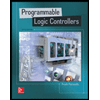
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education