C# PROGRAM convert this spaghetti code to modular (oop) use functions
C#
convert this spaghetti code to modular (oop) use functions
source code:
using System;
class Program {
static void Main() {
int opt; //to read the user input for option
bool running = true;
String[] sentenceArr = new String[] {"How are you?", "It is lovely day. Isn't it?", "It's a pleasure to meet you.","Hello.","A pleasant morning to you."}; //array of sentences
Random rand = new Random(); //to generate random Number
int walkCount = 0; //total number of walks
int walk = 0; //total number of time walk invoked
int hop = 0; //total number of times hop invoked
int wordCount = 0; //total number of words spoken
while(running){
Console.WriteLine("\n1 - Walk");
Console.WriteLine("2 - Speak");
Console.WriteLine("3 - Hop");
Console.WriteLine("4 - Show Distance");
Console.WriteLine("5 - Show Number of Words Spoken");
Console.WriteLine("-----------------------------------");
Console.Write("Select Option: ");
opt = Convert.ToInt32(Console.ReadLine()); //reading the user input for option and converting it to integer
switch(opt){
case 1:
//Case when option selected is Walk
{
//Increment walk counter
walk++;
//One walk means one Walk in Distance
walkCount +=1;
Console.WriteLine("walking");
break;
}
case 2:
//Case when option selected is Speak
{
//pick a random index of sentenceArr, and display the value at the index
int i = rand.Next(0,sentenceArr.Length); //generate a random value between 0 and last index
//display the value
String sentence = sentenceArr[i]; //sentence about to be spoken
String[] words = sentence.Split(' '); //split the sentence into words
wordCount += words.Length; //increment total number of words spoken
Console.WriteLine(sentence);
break;
}
case 3:
//Case when option selected is Hop
{
hop++; //increment hop counter
//One hop means 2 walk in Distance
walkCount +=2;
Console.WriteLine("hop");
break;
}
case 4:
//Case when option selected is Show Distance
{
//Show the details of hop and walk with total distance covered
Console.WriteLine($"Total number of time Hop invoked: {hop}");
Console.WriteLine($"Total number of time Walk invoked: {walk}");
Console.WriteLine($"Total distance walked: {walkCount}");
break;
}
case 5:
//Case when option selected is Show Number of Words Spoken
{
//Print total word Spoken
Console.WriteLine($"Total word spoken: {wordCount}");
break;
}
default:
//If any other value than the menu option is given, end the program
Console.WriteLine("Error: Invalid Option!");
Console.WriteLine("Exiting...!");
running = false; //stop the program
break;
}
}
}
}

Step by step
Solved in 2 steps

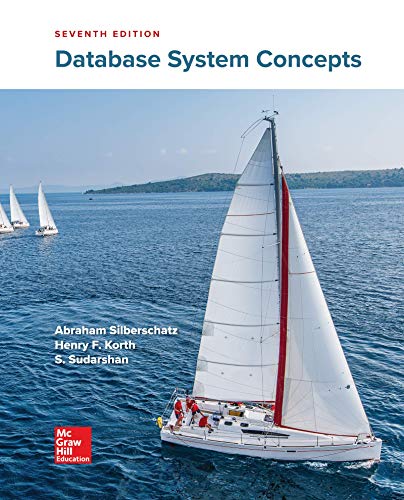
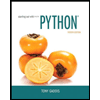
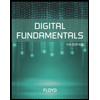
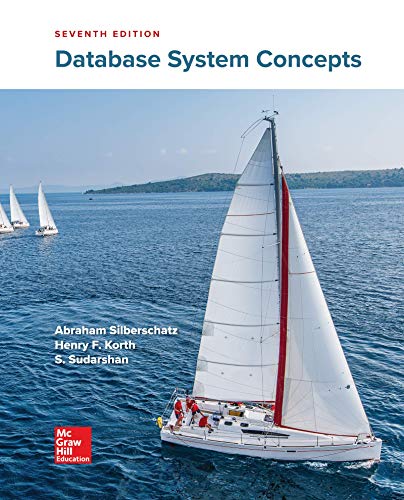
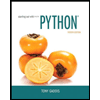
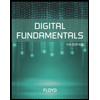
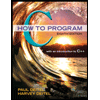
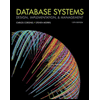
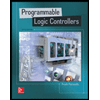