c++ my code is attached, along with an image of what problem i am experiencing. I know the code works fine, but when running it in the window in this particular form, my results show zero per the image i have attached and highlighted. *please note, i enter the input as follows. 5 space 10, enter then 2, enter then 1 i have also tried 5, enter, 10, enter then 2, enter then 1 and nothing works. Amanda and Tyler opened a business that specializes in shipping liquids, such as milk, juice, and water, in cylindrical containers. The shipping charges depend on the amount of the liquid in the container. (For simplicity, you may assume that the container is filled to the top.) They also provide the option to paint the outside of the container for a reasonable amount. Write program that does the following: Prompts the user to input the dimensions (in feet) of the container (radius of the base and the height). Prompts the user to input the shipping cost per liter. Prompts the user to input the paint cost per square foot. (Assume that the entire container including the top and bottom needs to be painted.) Separately outputs the shipping cost and the cost of painting. Your program must use the class cylinderType (designed in Programming Exercise 3) to store the radius of the base and the height of the container. (Note that 1 cubic feet = 28.32 liters or 1 liter = 0.353146667 cubic feet.) Format your output with setprecision(2) to ensure the proper number of decimals for testing! Also, beware of floating-point errors in your calculations. #include #include using namespace std; #define pi 3.141592 // pi is the value of 22/7 #define convert 28.320075 //28.32 will be used as convert, which is the conversion value of 1 cubic foot to litre class cylinderType{ double radius, height,volume,surface_area; //stores radius, height, volume and total surface area public: cylinderType(double radius,double height){ //constructor this->radius=radius; this->height=height; this->volume=pi*radius*radius*height; //calculate volume this->surface_area=2.0*pi*radius*(radius+ height); //Assuming that paint in applied on the top and bottom as well // cout<<"vol "<volume<<" "<surface_area<volume*convert)*shipping_rate; double total_painting_cost=this->surface_area*painting_rate; //This part prints Output cout<<"\nTotal Cost for Shipping: "<>rad>>len; cylinderType container(rad,len); //container is the instance of class cylinderType //This part takes Shipping rate as input cout<<"Enter Shipping Cost per Litre: "; cin>>shipping_rate; //This part takes painting rate as input cout<<"Enter the paint cost per square foot: "; cin>>painting_rate; //Call to print_total_cost() function to get results container.print_total_cost(shipping_rate,painting_rate); return 0; }
c++
my code is attached, along with an image of what problem i am experiencing. I know the code works fine, but when running it in the window in this particular form, my results show zero per the image i have attached and highlighted.
*please note, i enter the input as follows.
5 space 10, enter then 2, enter then 1
i have also tried 5, enter, 10, enter then 2, enter then 1 and nothing works.
Amanda and Tyler opened a business that specializes in shipping liquids, such as milk, juice, and water, in cylindrical containers. The shipping charges depend on the amount of the liquid in the container. (For simplicity, you may assume that the container is filled to the top.) They also provide the option to paint the outside of the container for a reasonable amount. Write program that does the following:
- Prompts the user to input the dimensions (in feet) of the container (radius of the base and the height).
- Prompts the user to input the shipping cost per liter.
- Prompts the user to input the paint cost per square foot. (Assume that the entire container including the top and bottom needs to be painted.)
- Separately outputs the shipping cost and the cost of painting. Your program must use the class cylinderType (designed in
Programming Exercise 3) to store the radius of the base and the height of the container. (Note that 1 cubic feet = 28.32 liters or 1 liter = 0.353146667 cubic feet.)
Format your output with setprecision(2) to ensure the proper number of decimals for testing! Also, beware of floating-point errors in your calculations.
#include <iostream>
#include<iomanip>
using namespace std;
#define pi 3.141592 // pi is the value of 22/7
#define convert 28.320075 //28.32 will be used as convert, which is the conversion value of 1 cubic foot to litre
class cylinderType{
double radius, height,volume,surface_area; //stores radius, height, volume and total surface area
public:
cylinderType(double radius,double height){ //constructor
this->radius=radius;
this->height=height;
this->volume=pi*radius*radius*height; //calculate volume
this->surface_area=2.0*pi*radius*(radius+ height); //Assuming that paint in applied on the top and bottom as well
// cout<<"vol "<<this->volume<<" "<<this->surface_area<<endl;
}
void print_total_cost(double shipping_rate,double painting_rate){ //Function to calculate and print results
//This part calculates total cost for shipping and painting
double total_shipping_cost=(this->volume*convert)*shipping_rate;
double total_painting_cost=this->surface_area*painting_rate;
//This part prints Output
cout<<"\nTotal Cost for Shipping: "<<fixed<<setprecision(2)<<total_shipping_cost<<endl;
cout<<"Total Cost for Painting: "<<fixed<<setprecision(2)<<total_painting_cost<<endl;
}
};
int main()
{
//This part takes Dimension as User Input & creates object of cylinderType
double len,rad,shipping_rate,painting_rate;
cout<<"Enter the radius and height(in feet) for container: ";
cin>>rad>>len;
cylinderType container(rad,len); //container is the instance of class cylinderType
//This part takes Shipping rate as input
cout<<"Enter Shipping Cost per Litre: ";
cin>>shipping_rate;
//This part takes painting rate as input
cout<<"Enter the paint cost per square foot: ";
cin>>painting_rate;
//Call to print_total_cost() function to get results
container.print_total_cost(shipping_rate,painting_rate);
return 0;
}


Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 3 images

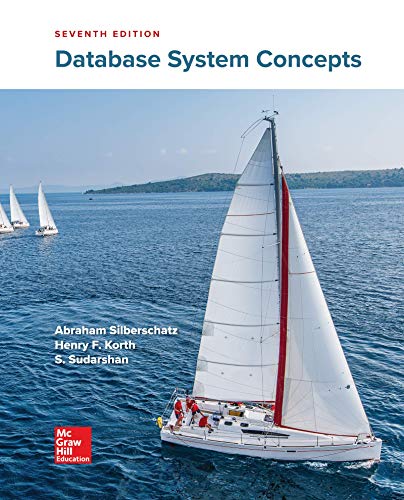
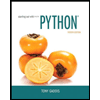
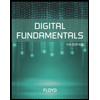
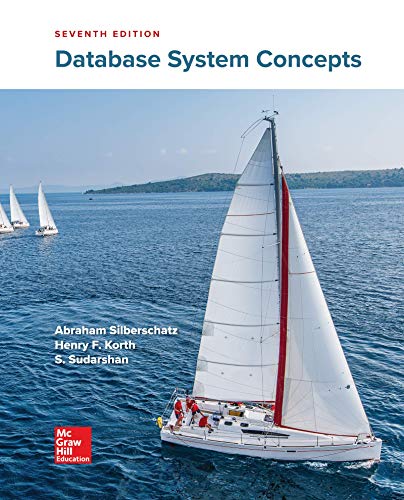
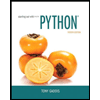
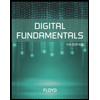
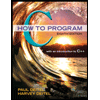
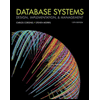
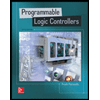