c++ Declare an Animal class that has the attributes of Name, Genus, and Weight. Declare four (4) specific animal classes that inherit attributes from the Animal class. In the lab we built two, Duck and Butterfly. Implement show() and speak() methods for each subclass. These methods will also be virtual classes in Animal and will allow the use of an array of Animal* in the main(). Your program should ask the user for the number of animals they would like in there zoo. Allow the user to select each animal from the animal subclasses you have implemented. Store the selections in an array of Animal*. After the user has selected all the animals, step thru the array and have each animal show() and speak() in a sort of parade of selected animals. I'm having a problem with the error message and why it won't display to ascii art and have the user input --- This is my code so far --- /* * File: Zoo.cpp * Author: * Course: CS I * Assignment: What's In Your Zoo? * Due date: 11/10/21 * * This program allows the user for the number of animals they would like in there zoo. Allow the user to select each animal from the animal subclasses that's implemented. * C++ */ #include using namespace std; class Animal { string name; string genus; double weight; public: Animal(string n, string g, double w) { name = n; genus = g; weight = w; } string to_string() { string str; str = "Name: " + name + "\nGenus: " + genus + "\nWeight: " + std::to_string(weight) + "grams\n"; return str; } virtualvoid show();//virtual - if it doesn't have a specific method or previous (allows it to take many different forms) virtualvoid speak(); }; class Duck : public Animal { public: Duck(string n, string g, double w) : Animal(n,g,w){} void show() { cout << " .. \n" << endl; cout << " ( '`< \n" << endl; cout << " )( \n" << endl; cout << " ( ----' '. \n" << endl; cout << " ( ; \n" << endl; cout << " (________,' \n" << endl; cout << "~^~^~^~^~^~^~^~^~^~^~ \n" << endl; cout << " \n" << endl; } void speak() { cout << " Quack! Quack!\n"; } }; class Fox: public Animal { public: Fox(string n, string g, double w) : Animal(n, g, w){} //Fox void show() //output image { cout << " /|_/| \n" << endl; cout << " / ^ ^(_o \n" << endl; cout << " / __.'' \n" << endl; cout << " / \\ \n" << endl; cout << " (_) (_) '._ \n" << endl; cout << " '.__ '. .-''-'. \n" << endl; cout << " ( '. ('.____.'' \n" << endl; cout << " _) )'_, ) \n" << endl; cout << " (__/ (__/ \n" << endl; } void speak() { cout << " ow-wow-wow-wow-wow! \n"; } }; class Parakeet: public Animal { public: Parakeet(string n, string g, double w) : Animal(n, g, w){} void show() //output image { cout << " \\ \n"; cout << " \\ (o> \n"; cout << " (o> //) \n"; cout << " _(()_____V_/_____ \n"; cout << " || || \n"; cout << " || \n"; } void speak() //output voice (txt) { cout << "Chirp, Chirp!\n"; } }; class Koala: public Animal { public: Koala(string n, string g, double w) : Animal(n, g, w){} void show() { cout << " (\\-'''-/) \n" << endl; cout << " | | \n" << endl; cout << " \\ ^ ^ / .-. \n" << endl; cout << " \\_o_/ / / \n" << endl; cout << " /` `\\// | \n" << endl; cout << " / \\ | \n" << endl; cout << " \\ ( ) / | \n" << endl; cout << " /\\_) (_/ \\ / \n" << endl; cout << "| (\\-/) | \n" << endl; cout << "\\ --^o^-- / \n" << endl; cout << " \\ '.___.' / \n" << endl; cout << " .' \\-=-/ '. \n" << endl; cout <<" / /` `\\ \\ \n" << endl; cout <<"(//./ \\.\\) \n" << endl; cout << "`'` `'` \n" << endl; } }; int main() { Animal* zoo[4]; zoo[0] = new Duck("Duck", "Anas", 900.0); zoo[0] -> to_string(); zoo[0] -> show(); zoo[0] -> speak(); for(int i=0; i<4; i++) { delete zoo[i]; } return 0; //int selection; /* Animal animal; cout << animal.to_string(); Duck.show; Duck.speak; return 0; */ }
c++
Declare an Animal class that has the attributes of Name, Genus, and Weight. Declare four (4) specific animal classes that inherit attributes from the Animal class. In the lab we built two, Duck and Butterfly. Implement show() and speak() methods for each subclass. These methods will also be virtual classes in Animal and will allow the use of an array of Animal* in the main().
Your program should ask the user for the number of animals they would like in there zoo. Allow the user to select each animal from the animal subclasses you have implemented. Store the selections in an array of Animal*. After the user has selected all the animals, step thru the array and have each animal show() and speak() in a sort of parade of selected animals.
I'm having a problem with the error message and why it won't display to ascii art and have the user input
--- This is my code so far ---
- * Due date: 11/10/21

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 5 images

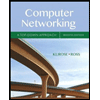
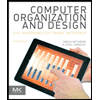
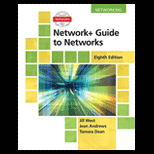
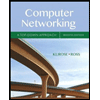
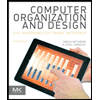
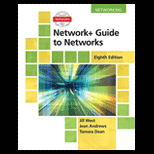
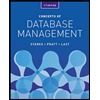
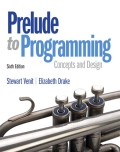
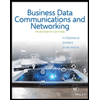