C++ 13.9.2 Pass by reference Define a function ScaleGrade() that takes two parameters: points: an integer, passed by value, for the student's score. grade: a char, passed by reference, for the student's letter grade. ScaleGrade() changes grade to A if the points are greater than or equal to 88 and less than or equal to 100, and grade is not A. Otherwise, grade is not changed. The function returns true if grade has changed, and returns false otherwise. Ex: If the input is 88 B, then the output is: Grade is A after curving. Using the following code #include using namespace std; // insert code here int main() { int studentPoints; char studentGrade; bool isChanged; cin >> studentPoints; cin >> studentGrade; isChanged = ScaleGrade(studentPoints, studentGrade); if (isChanged) { cout << "Grade is " << studentGrade << " after curving." << endl; } else { cout << "Grade " << studentGrade << " is not changed." << endl; } return 0; } * I was trying to use a code block like this: bool ScaleGrade(int& studentPoints, char& studentGrade){ if(studentPoints>=88 || studentPoints<=100){ studentGrade='A'; return false; } else{ return false; } } However I only can get half the checks to actually pass.
C++ 13.9.2 Pass by reference
Define a function ScaleGrade() that takes two parameters:
- points: an integer, passed by value, for the student's score.
- grade: a char, passed by reference, for the student's letter grade.
ScaleGrade() changes grade to A if the points are greater than or equal to 88 and less than or equal to 100, and grade is not A. Otherwise, grade is not changed. The function returns true if grade has changed, and returns false otherwise.
Ex: If the input is 88 B, then the output is:
Grade is A after curving.
Using the following code
#include <iostream>
using namespace std;
// insert code here
int main() {
int studentPoints;
char studentGrade;
bool isChanged;
cin >> studentPoints;
cin >> studentGrade;
isChanged = ScaleGrade(studentPoints, studentGrade);
if (isChanged) {
cout << "Grade is " << studentGrade << " after curving." << endl;
}
else {
cout << "Grade " << studentGrade << " is not changed." << endl;
}
return 0;
}
* I was trying to use a code block like this:
bool ScaleGrade(int& studentPoints, char& studentGrade){
if(studentPoints>=88 || studentPoints<=100){
studentGrade='A';
return false;
}
else{
return false;
}
}
However I only can get half the checks to actually pass.

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

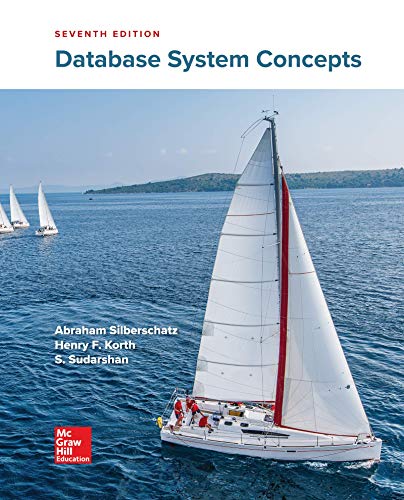
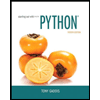
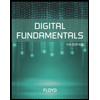
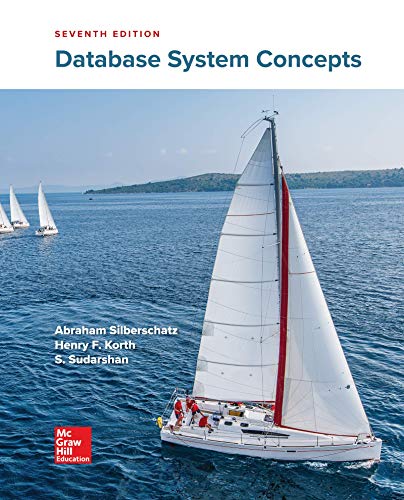
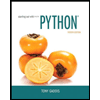
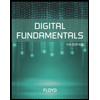
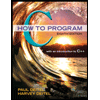
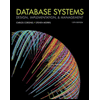
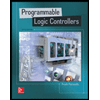