Building RESTful Web Services for Fibonacci Sequence Operations Using Spring Preface The Fibonacci sequence is a series of numbers where each number is the sum of the two preceding numbers. The sequence starts with 0 and 1, and each subsequent number is the sum of the two previous numbers. The sequence continues infinitely and goes as follows: 0, 1, 1, 2, 3, 5, 8, 13, 21, 34, 55, 89, 144, 233, 377, and so on. The sequence is named after Leonardo Fibonacci, an Italian mathematician who introduced the sequence to the Western world in his book Liber Abaci, which was published in 1202. The Fibonacci sequence has many interesting mathematical properties and has been observed in various natural phenomena, such as the branching of trees and the spirals in shells. Question In this assignment, you will be building a set of RESTful web services using Spring that will allow clients to perform several operations related to the Fibonacci sequence. The web services will be able to generate the Fibonacci sequence up to a specified number, generate the nth number in the sequence, calculate the sum of the first 6 numbers in the sequence, and check if a given number is a member of the sequence. Technical Tasks to do: 1. Start by creating a new Spring project in Spring Tool Suite (STS) or your preferred IDE, and then define suitable classes to handle the web service requests. 2. Implement the genFibonacci(n) web service to generate the Fibonacci sequence up to a specified number ‘n’ provided by the user in the URL. This should be done using a loop that generates each number in the sequence until the number exceeds the specified limit. A valid URL can be in the form of localhost:8080/assignment2/services/fiborestservice/genfibonacci/n where 'n' is an integer that satisfies 0 ≤ n < 100. 3. Implement the genFibo(n) web service to generate the nth number in the Fibonacci sequence, where n is a number provided by the user in the URL. This should be done using a loop that generates each number in the sequence until it reaches the nth number. A valid URL can be in the form of localhost:8080/assignment2/services/fiborestservice/genfibo/n where ‘n’ is an integer that satisfies 10 ≤ n ≤ 20. pg. 2 4. Implement the calcFib6Sum web service to calculate the sum of the first 6 numbers in the Fibonacci sequence. This should be done using a loop that generates each number in the sequence and adds it to a running total until it reaches the 6th number. A valid URL can be in the form of localhost:8080/assignment2/services/fiborestservice/calcfib6Sum 5. Implement the chkFib(n) web service to check if a given number ‘n’ is a member of the Fibonacci sequence. This should be done by generating each number in the sequence until the number is greater than n, and then checking if the last generated number in the sequence is equal to the given number. A valid URL can be in the form of localhost:8080/assignment2/services/fiborestservice/chkfib/n where ‘n’ is a non-negative integer excluding zero. 6. Ensure that the web services can produce and consume JSON objects. 7. Handle invalid data exceptions by using appropriate exception handlers in Spring. This should be done for all the web services where invalid input data exception handling makes sense. 8. Enrich the security of the web service by adding Basic Authentication. This will require clients to authenticate themselves using a username and password before they can access the web service. 9. Test all the developed endpoints in Postman to ensure that they function as expected.
Building RESTful Web Services for Fibonacci Sequence Operations Using Spring Preface The Fibonacci sequence is a series of numbers where each number is the sum of the two preceding numbers. The sequence starts with 0 and 1, and each subsequent number is the sum of the two previous numbers. The sequence continues infinitely and goes as follows: 0, 1, 1, 2, 3, 5, 8, 13, 21, 34, 55, 89, 144, 233, 377, and so on. The sequence is named after Leonardo Fibonacci, an Italian mathematician who introduced the sequence to the Western world in his book Liber Abaci, which was published in 1202. The Fibonacci sequence has many interesting mathematical properties and has been observed in various natural phenomena, such as the branching of trees and the spirals in shells. Question In this assignment, you will be building a set of RESTful web services using Spring that will allow clients to perform several operations related to the Fibonacci sequence. The web services will be able to generate the Fibonacci sequence up to a specified number, generate the nth number in the sequence, calculate the sum of the first 6 numbers in the sequence, and check if a given number is a member of the sequence. Technical Tasks to do: 1. Start by creating a new Spring project in Spring Tool Suite (STS) or your preferred IDE, and then define suitable classes to handle the web service requests. 2. Implement the genFibonacci(n) web service to generate the Fibonacci sequence up to a specified number ‘n’ provided by the user in the URL. This should be done using a loop that generates each number in the sequence until the number exceeds the specified limit. A valid URL can be in the form of localhost:8080/assignment2/services/fiborestservice/genfibonacci/n where 'n' is an integer that satisfies 0 ≤ n < 100. 3. Implement the genFibo(n) web service to generate the nth number in the Fibonacci sequence, where n is a number provided by the user in the URL. This should be done using a loop that generates each number in the sequence until it reaches the nth number. A valid URL can be in the form of localhost:8080/assignment2/services/fiborestservice/genfibo/n where ‘n’ is an integer that satisfies 10 ≤ n ≤ 20. pg. 2 4. Implement the calcFib6Sum web service to calculate the sum of the first 6 numbers in the Fibonacci sequence. This should be done using a loop that generates each number in the sequence and adds it to a running total until it reaches the 6th number. A valid URL can be in the form of localhost:8080/assignment2/services/fiborestservice/calcfib6Sum 5. Implement the chkFib(n) web service to check if a given number ‘n’ is a member of the Fibonacci sequence. This should be done by generating each number in the sequence until the number is greater than n, and then checking if the last generated number in the sequence is equal to the given number. A valid URL can be in the form of localhost:8080/assignment2/services/fiborestservice/chkfib/n where ‘n’ is a non-negative integer excluding zero. 6. Ensure that the web services can produce and consume JSON objects. 7. Handle invalid data exceptions by using appropriate exception handlers in Spring. This should be done for all the web services where invalid input data exception handling makes sense. 8. Enrich the security of the web service by adding Basic Authentication. This will require clients to authenticate themselves using a username and password before they can access the web service. 9. Test all the developed endpoints in Postman to ensure that they function as expected.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Building RESTful Web Services for Fibonacci Sequence Operations Using Spring Preface The Fibonacci sequence is a series of numbers where each number is the sum of the two preceding numbers. The sequence starts with 0 and 1, and each subsequent number is the sum of the two previous numbers. The sequence continues infinitely and goes as follows: 0, 1, 1, 2, 3, 5, 8, 13, 21, 34, 55, 89, 144, 233, 377, and so on. The sequence is named after Leonardo Fibonacci, an Italian mathematician who introduced the sequence to the Western world in his book Liber Abaci, which was published in 1202. The Fibonacci sequence has many interesting mathematical properties and has been observed in various natural phenomena, such as the branching of trees and the spirals in shells. Question In this assignment, you will be building a set of RESTful web services using Spring that will allow clients to perform several operations related to the Fibonacci sequence. The web services will be able to generate the Fibonacci sequence up to a specified number, generate the nth number in the sequence, calculate the sum of the first 6 numbers in the sequence, and check if a given number is a member of the sequence. Technical Tasks to do: 1. Start by creating a new Spring project in Spring Tool Suite (STS) or your preferred IDE, and then define suitable classes to handle the web service requests. 2. Implement the genFibonacci(n) web service to generate the Fibonacci sequence up to a specified number ‘n’ provided by the user in the URL. This should be done using a loop that generates each number in the sequence until the number exceeds the specified limit. A valid URL can be in the form of localhost:8080/assignment2/services/fiborestservice/genfibonacci/n where 'n' is an integer that satisfies 0 ≤ n < 100. 3. Implement the genFibo(n) web service to generate the nth number in the Fibonacci sequence, where n is a number provided by the user in the URL. This should be done using a loop that generates each number in the sequence until it reaches the nth number. A valid URL can be in the form of localhost:8080/assignment2/services/fiborestservice/genfibo/n where ‘n’ is an integer that satisfies 10 ≤ n ≤ 20. pg. 2 4. Implement the calcFib6Sum web service to calculate the sum of the first 6 numbers in the Fibonacci sequence. This should be done using a loop that generates each number in the sequence and adds it to a running total until it reaches the 6th number. A valid URL can be in the form of localhost:8080/assignment2/services/fiborestservice/calcfib6Sum 5. Implement the chkFib(n) web service to check if a given number ‘n’ is a member of the Fibonacci sequence. This should be done by generating each number in the sequence until the number is greater than n, and then checking if the last generated number in the sequence is equal to the given number. A valid URL can be in the form of localhost:8080/assignment2/services/fiborestservice/chkfib/n where ‘n’ is a non-negative integer excluding zero. 6. Ensure that the web services can produce and consume JSON objects. 7. Handle invalid data exceptions by using appropriate exception handlers in Spring. This should be done for all the web services where invalid input data exception handling makes sense. 8. Enrich the security of the web service by adding Basic Authentication. This will require clients to authenticate themselves using a username and password before they can access the web service. 9. Test all the developed endpoints in Postman to ensure that they function as expected.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
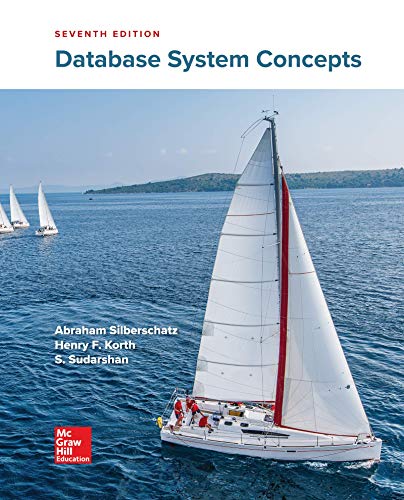
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
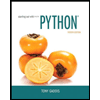
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
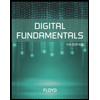
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
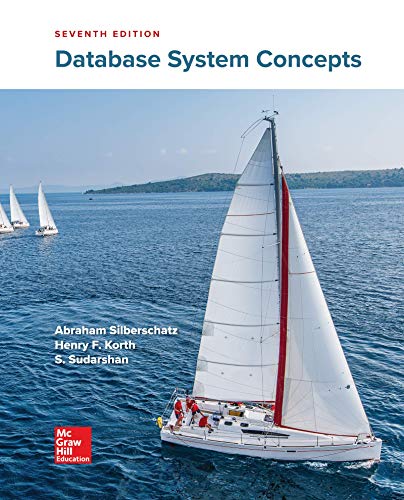
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
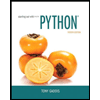
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
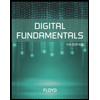
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
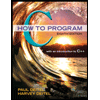
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
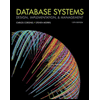
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
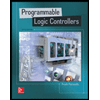
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education