BST - Binary Search Tree - implement a BSTNode ADT with a data attribute and two pointer attributes, one for the left child and the other for the right child. Implement the usual getters/setters for these attributes -implement a BST as a link-based ADT whose data will be Dollar objects - the data will be inserted based on the actual money value of your Dollar objects as a combination of the whole value and fractional value attributes. - BST, implement the four traversal methods as well as methods for the usual search, insert, delete, print, count, isEmpty, empty operations and any other needed. - BST - Binary Search Tree - implement a BSTNode ADT with a data attribute and two-pointer attributes, one for the left child and the other for the right child. Implement the usual getters/setters for these attributes -implement a BST as a link-based ADT whose data will be Dollar objects - the data will be inserted based on the actual money value of your Dollar objects as a combination of the whole value and fractional value attributes. - BST, implement the four traversal methods as well as methods for the usual search, insert, delete, print, count, isEmpty, empty operations and any other needed. pgm will have at least 10 Dollar objects created from data specified in your main to seed the tree. Read the data of your main to create your Dollar database using the BST. Also, create an output file to write program output as specified in one or more instructions below. Perform adequate data validation when reading data from the user into the tree - if any data item is invalid, ignore the data item and continue to next item but print a message to output (both screen and output file) to indicate which data items were ignored. Provide interactivity for the user to add/search/delete nodes from the console after the data has been seeded into the application, including data validation.
Types of Linked List
A sequence of data elements connected through links is called a linked list (LL). The elements of a linked list are nodes containing data and a reference to the next node in the list. In a linked list, the elements are stored in a non-contiguous manner and the linear order in maintained by means of a pointer associated with each node in the list which is used to point to the subsequent node in the list.
Linked List
When a set of items is organized sequentially, it is termed as list. Linked list is a list whose order is given by links from one item to the next. It contains a link to the structure containing the next item so we can say that it is a completely different way to represent a list. In linked list, each structure of the list is known as node and it consists of two fields (one for containing the item and other one is for containing the next item address).
BST - Binary Search Tree
- implement a BSTNode ADT with a data attribute and two pointer attributes, one for the left child and the other for the right child. Implement the usual getters/setters for these attributes
-implement a BST as a link-based ADT whose data will be Dollar objects - the data will be inserted based on the actual money value of your Dollar objects as a combination of the whole value and fractional value attributes.
- BST, implement the four traversal methods as well as methods for the usual search, insert, delete, print, count, isEmpty, empty operations and any other needed.
-
BST - Binary Search Tree
- implement a BSTNode ADT with a data attribute and two-pointer attributes, one for the left child and the other for the right child. Implement the usual getters/setters for these attributes
-implement a BST as a link-based ADT whose data will be Dollar objects - the data will be inserted based on the actual money value of your Dollar objects as a combination of the whole value and fractional value attributes.
- BST, implement the four traversal methods as well as methods for the usual search, insert, delete, print, count, isEmpty, empty operations and any other needed.
- pgm will have at least 10 Dollar objects created from data specified in your main to seed the tree.
- Read the data of your main to create your Dollar
database using the BST. - Also, create an output file to write program output as specified in one or more instructions below.
- Perform adequate data validation when reading data from the user into the tree - if any data item is invalid, ignore the data item and continue to next item but print a message to output (both screen and output file) to indicate which data items were ignored.
- Provide interactivity for the user to add/search/delete nodes from the console after the data has been seeded into the application, including data validation.
USE THIS CLASS:
class Currency {
protected:
int whole;
int fraction;
virtual string currName() = 0;
public:
Currency() {
whole = 0;
fraction = 0;
}
Currency(double value) {
if (value < 0)
throw "Invalid value";
whole = int(value);
fraction = round(100 * (value - whole));
}
Currency(const Currency &obj) {
whole = obj.whole;
fraction = obj.fraction;
}
~Currency() {}
void setWhole(int setW) {
if (setW >= 0)
whole = setW;
}
void setFraction(int setFrac) {
if (setFrac >= 0 && setFrac < 100)
fraction = setFrac;
}
int getWhole() { return whole; }
int getFraction() { return fraction; }
void add(const Currency *obj) {
whole += obj->whole;
fraction += obj->fraction;
if (fraction > 100) {
whole++;
fraction %= 100;
}
}
void subtract(const Currency *obj) {
if (!isGreater(*obj))
throw "Invalid Subtraction";
whole -= obj->whole;
if (fraction < obj->fraction) {
fraction = fraction + 100 - obj->fraction;
whole--;
} else {
fraction -= obj->fraction;
}
}
bool isEqual(const Currency &obj) {
return obj.whole == whole && obj.fraction == fraction;
}
bool isGreater(const Currency &obj) {
if (whole < obj.whole)
return false;
if (whole == obj.whole && fraction < obj.fraction)
return false;
return true;
}
void print() { cout << whole << "." << fraction << " " << currName() << " "; }
};

Step by step
Solved in 2 steps with 1 images

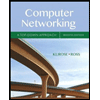
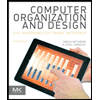
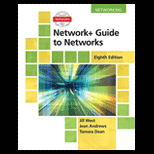
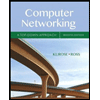
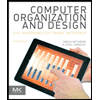
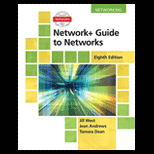
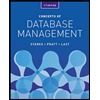
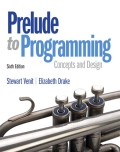
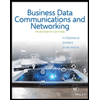