Based on the java code below, how to develop a Junit code to Test for public class Driver1 using JUNIT code package stubdriver; /** * Converts from one unit to another (e.g., inches to feet) * * @author Prof. David Bernstein, James Madison University * @version 1.0 (Implemented getMultiplier) */ public class UnitConverter1 { /** * Default Constructor */ public UnitConverter1() { } /** * Perform a conversion * * @param value The number to convert * @param from The units for value (e.g., "inches") * @param to The units to convert to (e.g., "feet") * @return The converted value */ publicdouble convert(double value, String from, String to) { double result; result = value * getMultiplier(from, to); return result; } /** * Get the multiplier needed for a conversion * * @param from The units to convert from (e.g., "inches") * @param to The units to convert to (e.g., "feet") * @return What "from" needs to be multiplied by to get "to" */ publicdouble getMultiplier(String from, String to) { double multiplier; multiplier = 1.0; if (from.equals("inches")) { if (to.equals("feet")) multiplier = 1.0/12.0; else if (to.equals("yards")) multiplier = 1.0/12.0/3.0; else if (to.equals("miles")) multiplier = 1.0/12.0/3.0/1760.0; } else if (from.equals("feet")) { if (to.equals("inches")) multiplier = 12.0; else if (to.equals("yards")) multiplier = 1.0/3.0; else if (to.equals("miles")) multiplier = 1.0/3.0/1760.0; } else if (from.equals("yards")) { if (to.equals("inches")) multiplier = 3.0*12.0; else if (to.equals("feet")) multiplier = 3.0; else if (to.equals("miles")) multiplier = 1.0/1760.0; } else if (from.equals("miles")) { if (to.equals("inches")) multiplier = 12.0*3.0*1760.0; else if (to.equals("yards")) multiplier = 1760.0; else if (to.equals("feet")) multiplier = 3.0*1760.0; } return multiplier; } }
Based on the java code below, how to develop a Junit code to Test for public class Driver1 using JUNIT code
package stubdriver;
/**
* Converts from one unit to another (e.g., inches to feet)
*
* @author Prof. David Bernstein, James Madison University
* @version 1.0 (Implemented getMultiplier)
*/
public class UnitConverter1
{
/**
* Default Constructor
*/
public UnitConverter1()
{
}
/**
* Perform a conversion
*
* @param value The number to convert
* @param from The units for value (e.g., "inches")
* @param to The units to convert to (e.g., "feet")
* @return The converted value
*/
publicdouble convert(double value, String from, String to)
{
double result;
result = value * getMultiplier(from, to);
return result;
}
/**
* Get the multiplier needed for a conversion
*
* @param from The units to convert from (e.g., "inches")
* @param to The units to convert to (e.g., "feet")
* @return What "from" needs to be multiplied by to get "to"
*/
publicdouble getMultiplier(String from, String to)
{
double multiplier;
multiplier = 1.0;
if (from.equals("inches")) {
if (to.equals("feet")) multiplier = 1.0/12.0;
else if (to.equals("yards")) multiplier = 1.0/12.0/3.0;
else if (to.equals("miles")) multiplier = 1.0/12.0/3.0/1760.0;
} else if (from.equals("feet")) {
if (to.equals("inches")) multiplier = 12.0;
else if (to.equals("yards")) multiplier = 1.0/3.0;
else if (to.equals("miles")) multiplier = 1.0/3.0/1760.0;
} else if (from.equals("yards")) {
if (to.equals("inches")) multiplier = 3.0*12.0;
else if (to.equals("feet")) multiplier = 3.0;
else if (to.equals("miles")) multiplier = 1.0/1760.0;
} else if (from.equals("miles")) {
if (to.equals("inches")) multiplier = 12.0*3.0*1760.0;
else if (to.equals("yards")) multiplier = 1760.0;
else if (to.equals("feet")) multiplier = 3.0*1760.0;
}
return multiplier;
}
}
|
package stubdriver; /**
original = 10.0; from = units[i]; // This could be outside the inner loop } |

Step by step
Solved in 2 steps with 1 images

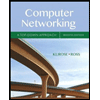
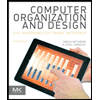
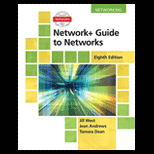
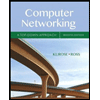
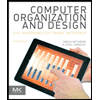
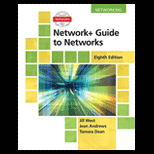
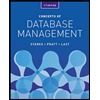
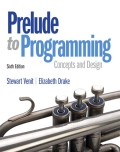
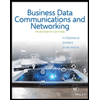