Checkpoint 3.59 Assume the variables name1 and name2 reference two different String objects, containing different strings. Write code that displays the strings referenced by these variables in alphabetical order. System.out.println(name1); System.out.println(name2); } else { System.out.println(name2); System.out.println(name1); } Fill in the blank. + namel + alphabetically.");| + comes before
Checkpoint 3.59 Assume the variables name1 and name2 reference two different String objects, containing different strings. Write code that displays the strings referenced by these variables in alphabetical order. System.out.println(name1); System.out.println(name2); } else { System.out.println(name2); System.out.println(name1); } Fill in the blank. + namel + alphabetically.");| + comes before
Chapter8: Advanced Method Concepts
Section: Chapter Questions
Problem 8RQ
Related questions
Question
java

Transcribed Image Text:### Checkpoint 3.59
#### Problem Statement:
Assume the variables `name1` and `name2` reference two different `String` objects, containing different strings. Write code that displays the strings referenced by these variables in alphabetical order.
```java
________
{
System.out.println(name1);
System.out.println(name2);
}
else
{
System.out.println(name2);
System.out.println(name1);
}
```
#### Task:
Fill in the blank.
#### Incorrect Attempt:
An attempt was made to fill in the blank with:
```java
+ " comes before " + name1 + " alphabetically.");
```
This resulted in an incorrect response, as marked by the red 'X' and the message "Incorrect. Try again."
### Solution Explanation:
To correctly display `name1` and `name2` in alphabetical order, you need to compare the two strings using the `compareTo` method of the `String` class.
Here is the correct code:
```java
if (name1.compareTo(name2) < 0)
{
System.out.println(name1);
System.out.println(name2);
}
else
{
System.out.println(name2);
System.out.println(name1);
}
```
### Detailed Explanation:
1. **String Comparison:**
- `name1.compareTo(name2) < 0` checks if `name1` is alphabetically before `name2`. The `compareTo` method returns:
- A negative integer if `name1` comes before `name2`.
- Zero if `name1` and `name2` are equal.
- A positive integer if `name1` comes after `name2`.
2. **If-Else Structure:**
- If the condition `name1.compareTo(name2) < 0` is true, `name1` is printed first followed by `name2`.
- If the condition is false, `name2` is printed first followed by `name1`.
This ensures that the strings are always printed in alphabetical order.
Remember to always test the logic with different strings to ensure correctness.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
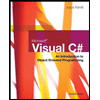
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
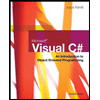
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,