Assume n is a positive integer representing input size for the following pseudocodes. Determine a function T(n) that relates input size n to number of runtime steps. (If you can't get the exact T(n), what it should roughly look like is enough. E.g. T(n)= an^2+k) What Big O set does this function belong to? Use common Big O sets when possible. Show your thought process. Note: The ellipsis (...) is meant to indicate a repetition until the pattern is finished. (i.e. you can rewrite (1, 2, 3, 4, 5, 6, 7, 8, 9, 10) as (1, 2, ..., 10)). For fl it means the print() is repeated until you reach print(n). For f6, the looping is repeated until you reach for i_n in range(n). print() may be thought of as always being O(1). Hint: Treat these snippets not as code that would actually be written and run in python. Rather treat this as pseudocodes (especially the ones with ellipses). Think of what algorithm is represented (instead of how it is written) and how it scales with respect to n def f1 (n): def f5 (n) : while n > 0: print (n) n = (n/2) + 0.1 for i 1 in range (n): for i 2 in range (n): for def f7 (n, b = ""): if n > 1: print (1) print (2) print (n-4) print (n-3) total = 0 for z in range (n): def f2 (n) : for i in range (n): for j in range (i): total + 1 1 for j in range (n): break print (n) def f4 (n, list_of_length_n): print (list_of_length_n) def f3 (n): 1 for i in range (n): def f6 (n) : in in range (n): f3 (n) f7 (n/3, b + "0") f7 (n/3, b + "1") f7 (n/3, b + "2") f7 (n/3, b + "3") print (b) else:
Assume n is a positive integer representing input size for the following pseudocodes. Determine a function T(n) that relates input size n to number of runtime steps. (If you can't get the exact T(n), what it should roughly look like is enough. E.g. T(n)= an^2+k) What Big O set does this function belong to? Use common Big O sets when possible. Show your thought process. Note: The ellipsis (...) is meant to indicate a repetition until the pattern is finished. (i.e. you can rewrite (1, 2, 3, 4, 5, 6, 7, 8, 9, 10) as (1, 2, ..., 10)). For fl it means the print() is repeated until you reach print(n). For f6, the looping is repeated until you reach for i_n in range(n). print() may be thought of as always being O(1). Hint: Treat these snippets not as code that would actually be written and run in python. Rather treat this as pseudocodes (especially the ones with ellipses). Think of what algorithm is represented (instead of how it is written) and how it scales with respect to n def f1 (n): def f5 (n) : while n > 0: print (n) n = (n/2) + 0.1 for i 1 in range (n): for i 2 in range (n): for def f7 (n, b = ""): if n > 1: print (1) print (2) print (n-4) print (n-3) total = 0 for z in range (n): def f2 (n) : for i in range (n): for j in range (i): total + 1 1 for j in range (n): break print (n) def f4 (n, list_of_length_n): print (list_of_length_n) def f3 (n): 1 for i in range (n): def f6 (n) : in in range (n): f3 (n) f7 (n/3, b + "0") f7 (n/3, b + "1") f7 (n/3, b + "2") f7 (n/3, b + "3") print (b) else:
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question

Transcribed Image Text:Assume n is a positive integer representing input size for the following
pseudocodes. Determine a function T(n) that relates input size n to number of runtime steps. (If you can't get the
exact T(n), what it should roughly look like is enough. E.g. T(n) = an^2 +k) What Big O set does this function
belong to? Use common Big O sets when possible. Show your thought process.
Note: The ellipsis (...) is meant to indicate a repetition until the pattern is finished. (i.e. you can rewrite (1, 2, 3, 4, 5, 6,
7, 8, 9, 10) as (1, 2, ..., 10)). For fl it means the print() is repeated until you reach print(n). For f6, the looping is
repeated until you reach for i_n in range(n).print() may be thought of as always being O(1).
Hint: Treat these snippets not as code that would actually be written and run in python. Rather treat this as
pseudocodes (especially the ones with ellipses). Think of what algorithm is represented (instead of how it is written)
and how it scales with respect to n
def f1 (n):
def f5 (n):
while n > 0:
print (n)
n = (n/2) + 0.1
for i 1 in range (n):
print (1)
print (2)
print (n-4)
print (n-3)
total = 0
for z in range (n):
def f2 (n):
for i in range (n):
for j in range (i):
total + 1
for j in range(n):
break
print (n)
def f4 (n, list_of_length_n):
print (list_of_length_n)
def f3 (n):
for i in range(n):
def f6 (n):
for i 2 in range (n):
for in in range (n):
f3 (n)
def f7 (n, b = ""):
if n > 1:
f7 (n/3, b +
"0")
f7 (n/3, b + "1")
f7 (n/3, b + "2")
f7 (n/3, b + "3")
print (b)
else:
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps

Recommended textbooks for you
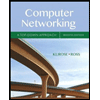
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
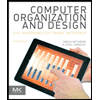
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
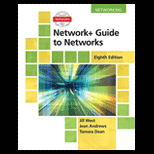
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
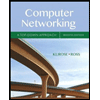
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
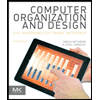
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
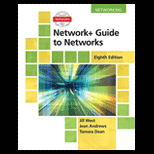
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
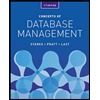
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
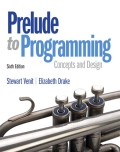
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
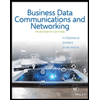
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY