ASSIGNMENT: Write a program to use the capability of Recursion to calculate factorials. For example, 5 factorial is normally written as 5! 5! = 5*4*3*2*1 5! = 120 Use recursive function calling to multiply. 5*4*3*2*1 And then print the result. 120 Your output should resemble the image below. >sh -c java d. -type f >java -cla 5 2 1 5! = 120 Note: 5! is use in this example but your program should calculate the factorial for any number entered.


in java
import java.util.Scanner;
public class Factorial
{
public static void main(String[] args)
{
Scanner scan=new Scanner(System.in);
System.out.print("Enter a number to find its factorial value : ");
//read an integer value from keboard
int num=Integer.parseInt(scan.nextLine());
//Calling fact method
System.out.printf("Facorial of %d is %d ", num,fact(num));
}
/*Recursive method, fact
* Method name:fact
* Input arguments:n as integer
* Output arguments: an integer value
* */
public static int fact(int n)
{
//Base case :
//Return 1 if n value is either 0 or 1
if(n==0 || n==1)
return 1;
else
//calling method, fact with n-1
return n*fact(n-1);
}//
}//
Step by step
Solved in 2 steps with 1 images

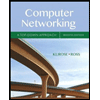
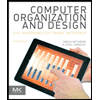
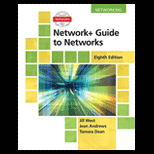
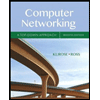
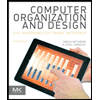
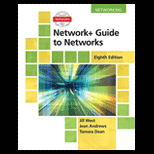
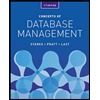
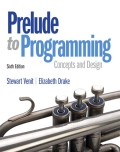
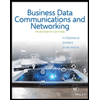