Alter insertion sort below to sort a list of strings instead of numbers. It shouldn't return anything - it should sort the list "in place". The sorting should ignore case. For example "Zebra" should come after "apple", "maRker" should come after "marble", etc. Name this function string_sort. After calling this function, the list that was passed in should now contain the exact same strings it did before, but in sorted order. def insertion_sort(a_list): """ Sorts a_list in ascending order """ for index in range(1, len(a_list)): value = a_list[index] pos = index - 1 while pos >= 0 and a_list[pos] > value: a_list[pos + 1] = a_list[pos] pos -= 1 a_list[pos + 1] = value
Alter insertion sort below to sort a list of strings instead of numbers. It shouldn't return anything - it should sort the list "in place". The sorting should ignore case. For example "Zebra" should come after "apple", "maRker" should come after "marble", etc. Name this function string_sort. After calling this function, the list that was passed in should now contain the exact same strings it did before, but in sorted order.
def insertion_sort(a_list):
"""
Sorts a_list in ascending order
"""
for index in range(1, len(a_list)):
value = a_list[index]
pos = index - 1
while pos >= 0 and a_list[pos] > value:
a_list[pos + 1] = a_list[pos]
pos -= 1
a_list[pos + 1] = value

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

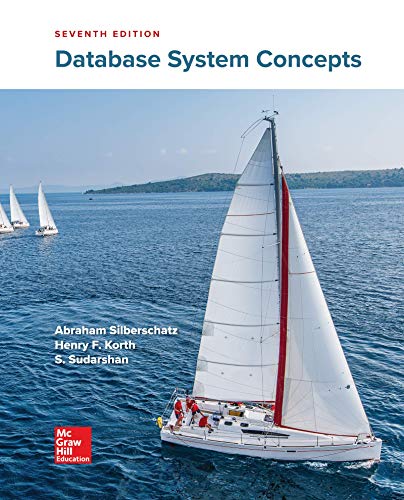
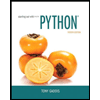
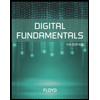
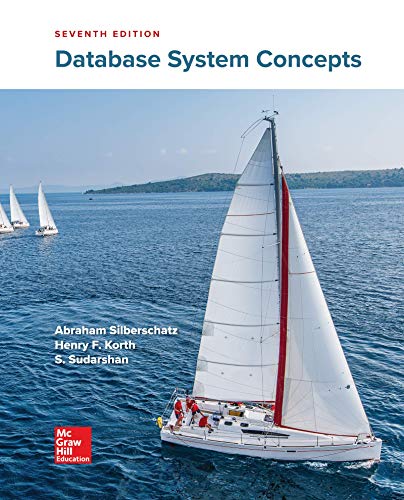
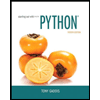
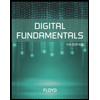
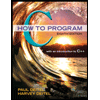
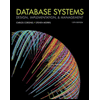
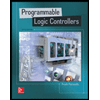