Use C++ to program a hash with a couple of people and their interconnected birth years. Next, show the people's names and their birth years, and have the user enter one. Reverse the hash and show the birth years, having the user choose a birth year. Then, show the person's name for that birth year.
Use C++ to

1. Create an empty unordered_map to store birth years and corresponding names (birthYearToName).
2. Populate the hash map with pairs of birth years and names:
- Insert key-value pairs into the birthYearToName hash map.
3. Display the available birth years and corresponding names:
- For each pair (birthYear, name) in birthYearToName:
- Print "Birth Year: birthYear, Name: name".
4. Prompt the user to enter a birth year.
- Read the user input into selectedBirthYear.
5. Use the find function to look up the corresponding name for the entered birth year:
- Set 'found' to the result of birthYearToName.find(selectedBirthYear).
6. If 'found' is not equal to birthYearToName.end():
- Display the name associated with the entered birth year:
- Print "Name for birth year selectedBirthYear: found->second".
- Else, if 'found' is equal to birthYearToName.end():
- Print "No person found for the entered birth year."
7. End the program.
Step by step
Solved in 5 steps with 2 images

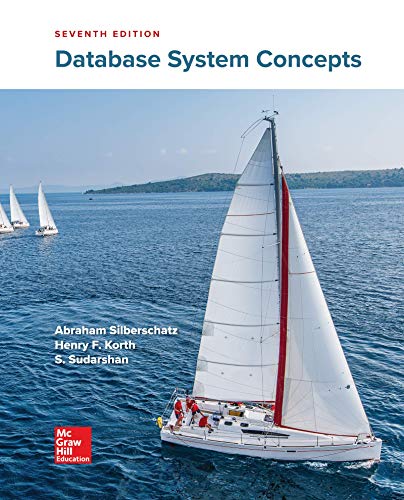
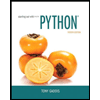
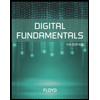
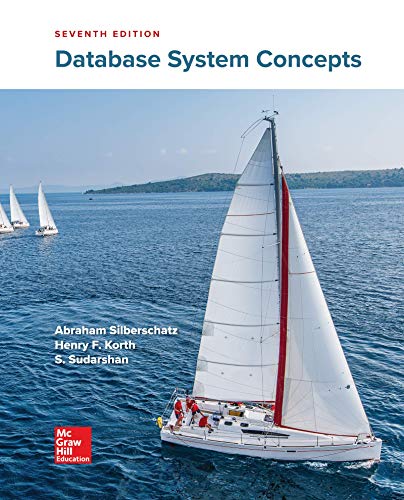
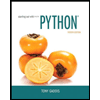
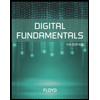
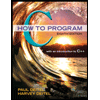
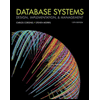
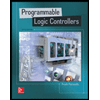