a. Here is pseudocode for a program that inputs a value n from the user and theninserts n random numbers, ensuring that there are no duplicates:Input n from userCreate vector v of type intLoop i = 1 to nr = random integer between 0 and n-1Linearly search through v for value rif r is not in vector v then add r to the end of vEnd LoopPrint out number of elements added to v Implement this program with your own linear search routine and add wrap-per code that will time how long it takes to run. Test the program for different values of n. Depending on the speed of your system, you may need to inputlarge values for n so that the program takes at least one second to run. Here is asample that indicates how to calculate the difference in time (time.h is a librarythat should be available on your version of C++):#include <time.h>time_t start,end;double dif;time (&start); // Record start time// Rest of program goes here.time (&end); // Record end timedif = difftime(end,start);cout << "It took " << dif << " seconds to execute. " << endl;
a. Here is pseudocode for a
inserts n random numbers, ensuring that there are no duplicates:
Input n from user
Create
Loop i = 1 to n
r = random integer between 0 and n-1
Linearly search through v for value r
if r is not in vector v then add r to the end of v
End Loop
Print out number of elements added to v
Implement this program with your own linear search routine and add wrap-
per code that will time how long it takes to run. Test the program for different
values of n. Depending on the speed of your system, you may need to input
large values for n so that the program takes at least one second to run. Here is a
sample that indicates how to calculate the difference in time (time.h is a library
that should be available on your version of C++):
#include <time.h>
time_t start,end;
double dif;
time (&start); // Record start time
// Rest of program goes here.
time (&end); // Record end time
dif = difftime(end,start);
cout << "It took " << dif << " seconds to execute. " << endl;

Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

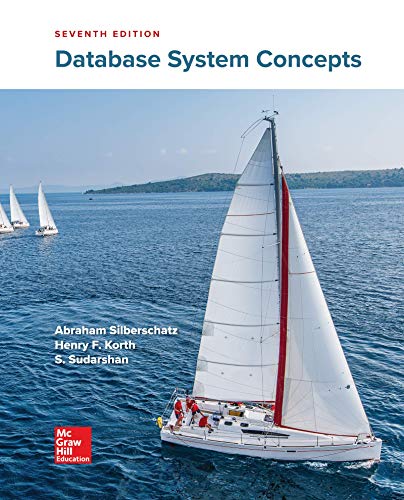
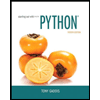
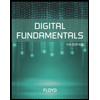
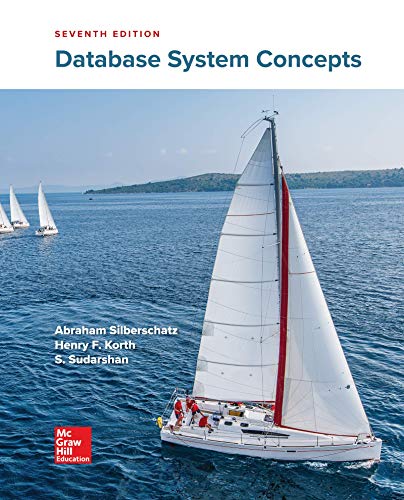
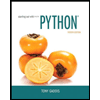
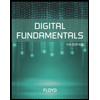
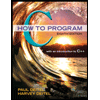
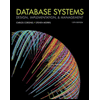
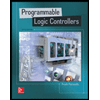