A non-profit organization has asked you to build a computer programming system that keeps up with the number of hours a volunteer has worked each week. Since no volunteer will work more than three days, the organization wants the volunteers to be able to enter their name and the hours volunteered for each of the three days they came in each week. The program will also make sure that the data entered is valid. The program will make sure the hours entered are a positive number and that the hours are no more than 8 hours each day. If the volunteer enters an invalid number of hours, then the program will ask them to re-enter the value and repeat the data entry process. You can follow these steps to guide you through the process: 1. Create a public class named Volunteer and one named VolunteerEntry. 2. The Volunteer class will contain instance variables for each object to hold data pertaining to each object as needed. It will store the values for the volunteer’s name, ID and total hours worked for the week. a. The Volunteer ID should be automatically generated when the object is instantiated. The first volunteer will be 1 and the next volunteer will be 2, etc. b. The name should be entered by the volunteer in the main() and set using a mutator (set) method in the Volunteer class. c. The hours for 3 days should be entered by the volunteer in the main() and set in the Volunteer class. 3. The VolunteerEntry class will have contain the main() method that will: a. Instantiate a Volunteer object. b. Asks for the volunteer’s name. c. Ask the volunteer to enter hours for each of three days. d. Will determine if the volunteer has entered a valid entry (between 0 and 8 hours). e. If the user does not enter a valid entry, a message should be displayed asking them to re-enter a valid value and repeat the entry process until a valid value has been entered. This can be done with a nested loop or by a method call. f. Once the volunteer has entered three valid values, then the program should output the volunteer’s name, ID, and total hours volunteered for the three days. I have come up with this code so far but I need assistance completing it . import java.util.*; public class Volunteer { // declaring instant variables to be used String volunteers name, ID, total hours worked; float name, ID, total hours worked; public static void main(String args[]) // main functio
A non-profit organization has asked you to build a computer programming system that
keeps up with the number of hours a volunteer has worked each week.
Since no volunteer will work more than three days, the organization wants the volunteers
to be able to enter their name and the hours volunteered for each of the three days they
came in each week.
The program will also make sure that the data entered is valid. The program will make sure
the hours entered are a positive number and that the hours are no more than 8 hours each
day. If the volunteer enters an invalid number of hours, then the program will ask them to
re-enter the value and repeat the data entry process.
You can follow these steps to guide you through the process:
1. Create a public class named Volunteer and one named VolunteerEntry.
2. The Volunteer class will contain instance variables for each object to hold data
pertaining to each object as needed. It will store the values for the volunteer’s name, ID
and total hours worked for the week.
a. The Volunteer ID should be automatically generated when the object is instantiated.
The first volunteer will be 1 and the next volunteer will be 2, etc.
b. The name should be entered by the volunteer in the main() and set using a mutator
(set) method in the Volunteer class.
c. The hours for 3 days should be entered by the volunteer in the main() and set in the
Volunteer class.
3. The VolunteerEntry class will have contain the main() method that will:
a. Instantiate a Volunteer object.
b. Asks for the volunteer’s name.
c. Ask the volunteer to enter hours for each of three days.
d. Will determine if the volunteer has entered a valid entry (between 0 and 8 hours).
e. If the user does not enter a valid entry, a message should be displayed asking them
to re-enter a valid value and repeat the entry process until a valid value has been
entered. This can be done with a nested loop or by a method call.
f. Once the volunteer has entered three valid values, then the program should output
the volunteer’s name, ID, and total hours volunteered for the three days.
I have come up with this code so far but I need assistance completing it .
import java.util.*;
public class Volunteer
{
// declaring instant variables to be used
String volunteers name, ID, total hours worked;
float name, ID, total hours worked;
public static void main(String args[]) // main function

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 2 images

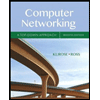
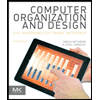
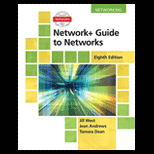
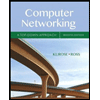
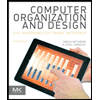
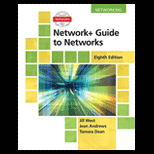
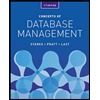
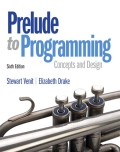
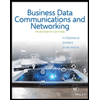