A fibonacci series is defined as a series where the number at the current index, is the value of the summation of the index preceding it (index -1) and (index-2). Essentially, for a list fibonacci_numbers which is the fibonacci numbers series, fibonacci_numbers[i] = fibonacci_numbers[i-1] + fibonacci_numbers[i-2] The fibonacci series always begins with 0, and then a 1 follows. So an example for fibonacci series up to the first 7 values would be - 0, 1, 1, 2, 3, 5, 8, 13 Complete the fibonacci(n) function, which takes in an index, n, and returns the nth value in the sequence. Any negative index values should return -1. Ex: If the input is: 7 the output is: fibonacci(7) is 13 Important Note Use recursion and DO NOT use any loops. Review the Week 9 class recording to see a variation of this solution. def fibonacci(n): if (n < 0 ): return -1 else: return n fibonacci_numbers = (fibonacci[n - 1] + fibonacci[n - 2]) return fibonacci_numbers(n) # TODO: Write recursive fibonacci() function if __name__ == "__main__": start_num = int(input()) print(f'fibonacci({start_num}) is {fibonacci(start_num)}') Input 20 Your output fibonacci(20) is 20 Expected output fibonacci(20) is 6765 Your function is not implemented using recursion
A fibonacci series is defined as a series where the number at the current index, is the value of the summation of the index preceding it (index -1) and (index-2). Essentially, for a list fibonacci_numbers which is the fibonacci numbers series, fibonacci_numbers[i] = fibonacci_numbers[i-1] + fibonacci_numbers[i-2]
The fibonacci series always begins with 0, and then a 1 follows. So an example for fibonacci series up to the first 7 values would be -
0, 1, 1, 2, 3, 5, 8, 13
Complete the fibonacci(n) function, which takes in an index, n, and returns the nth value in the sequence. Any negative index values should return -1.
Ex: If the input is:
7
the output is:
fibonacci(7) is 13
Important Note
- Use recursion and DO NOT use any loops.
- Review the Week 9 class recording to see a variation of this solution.
def fibonacci(n):
if (n < 0 ):
return -1
else:
return n
fibonacci_numbers = (fibonacci[n - 1] + fibonacci[n - 2])
return fibonacci_numbers(n)# TODO: Write recursive fibonacci() function
if __name__ == "__main__":
start_num = int(input())
print(f'fibonacci({start_num}) is {fibonacci(start_num)}') -
Input20Your outputfibonacci(20) is 20Expected outputfibonacci(20) is 6765Your function is not implemented using recursion

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

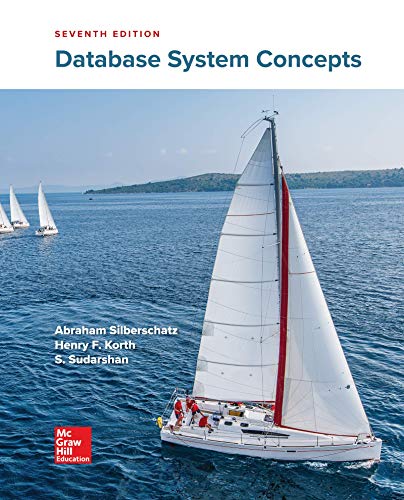
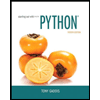
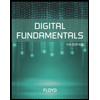
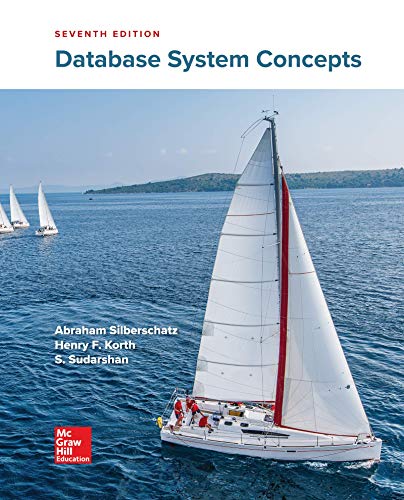
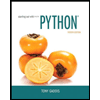
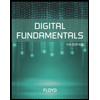
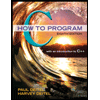
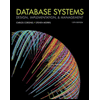
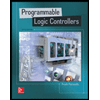