a: create abstract class and / or interface for the juke box code as below: import java.util.ArrayList; import java.util.List; import java.util.Queue; import java.util.Set; // The Jukebox class represents the body of the problem. Many of the interactions between the components of the system, // or between the system and the user, are channeled through here. public class JukeBox { private CDPlayer cdPlayer; private Set < CD > cdCollection;
Java:
create abstract class and / or interface for the juke box code as below:
import java.util.ArrayList;
import java.util.List;
import java.util.Queue;
import java.util.Set;
// The Jukebox class represents the body of the problem. Many of the interactions between the components of the system,
// or between the system and the user, are channeled through here.
public class JukeBox {
private CDPlayer cdPlayer;
private Set < CD > cdCollection;
private SongSelector ts;
private User user;
public JukeBox(CDPlayer cdPlayer, User user, Set < CD > cdCollection, SongSelector ts) {
this.cdPlayer = cdPlayer;
this.user = user;
this.cdCollection = cdCollection;
this.ts = ts;
}
public Song getCurrentSong() {
return ts.getCurrentSong();
}
public CD selectNext() {
return cdCollection.iterator().next();
}
public void setUser(User u) {
this.user = u;
}
}
class SongSelector {
private Song currentSong;
public SongSelector(Song s) {
currentSong = s;
}
public void setSong(Song s) {
currentSong = s;
}
public Song getCurrentSong() {
return currentSong;
}
}
// Like a real CD player, the CDP layer class supports storing just one CD at a time. The CDs that are not in play are stored in the jukebox.
class CDPlayer {
private Playlist p;
private CD c;
private Song s;
/* Constructors. */
public CDPlayer(CD c, Playlist p, Song S) {
this.c = c;
this.p = p;
this.s = s;
}
public CDPlayer(Playlist p) {
this.p = p;
}
public CDPlayer(CD c) {
this.c = c;
}
public CDPlayer(Song s) {
this.s = s;
}
/* Playsong */
public Song getSong() {
return s;
}
public void PlaySong(Song s) {
this.s = s;
}
/* Getters and setters */
public Playlist getPlaylist() {
return p;
}
public void setPlaylist(Playlist p) {
this.p = p;
}
public CD getCD() {
return c;
}
public void setCD(CD c) {
this.c = c;
}
}
class Playlist {
private Song song;
private Queue < Song > queue;
public Playlist(Song song, Queue < Song > queue) {
this.song = queue;
}
public Song getNextSongToPlay() {
return queue.peek();
}
public void queueUpSong(Song song) {
queue.add(song);
}
public void removeSong(Song song) {
queue.remove(song);
}
}
class CD {
/* data for id, artist, songs*/
private int id;
private String artist;
private String songs;
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getArtist() {
return artist;
}
public void setArtist(String artist) {
this.artist = artist;
}
public String getSongs() {
return songs;
}
public void setSongs(String song) {
this.songs = songs;
}
// play the music
public void play() {}
}
class Song {
/* data for id, CD (could be null), title, etc */
private int id;
private String title;
private String album;
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getTitle() {
return title;
}
public void setTitle(String title) {
this.title = title;
}
public String getAlbum() {
return album;
}
public void setAlbum(String album) {
this.album = album;
}
// play the music
public void play() {}
}
class User {
private String name;
private long ID;
public User(String name, long iD) {
this.name = name;
this.ID = iD;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public long getID() {
return ID;
}
public void setID(long iD) {
ID = iD;
}
public User getUser() {
return this;
}
public static User addUser(String name, long iD) {
return new User(name, iD);
}
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps

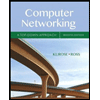
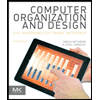
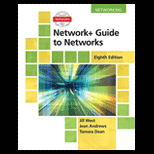
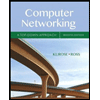
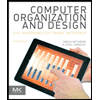
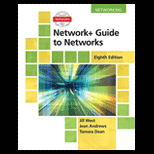
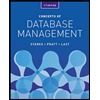
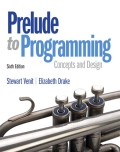
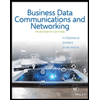