A company office processes print jobs using a priority status. The class PrintJob describes the job using the data member priority to rank the job and data member time to measure its length. When comparing two jobs, the one with the lower value for priority is considered the most important. Complete the PrintJob class definition (Note: You cannot change anything for the class except adding code to complete the definition of its methods and constructor) class PrintJob { private int priority; private int time; PrintJob(int pty, int t) { // constructor } int getPriority ( ) { // return the priority value of the job } int getTime( ) { // return the length of the job } void setPrintData(int pty, int t) { // Reset the priority and length of the job } } Create a JobSort class that contains only a static method sort(PrintJob pj[], int n). The sort method will sort the PrintJob objects pj[] in ascending order based the job priority. class JobSort { static void sort(PrintJob pj[], int n) { } } Write a class JobReport including a main method that: (1) Defines a PrintJob array and necessary variables (2) Reads job’s priority and time from keyboard, and stores them as an item (PrintJob object) in the array (3) Repeat (2) for more items until user presses a specific key to stop it (4) Sorts the array by calling the method sort() (5) Print the jobs stored in the array in their order of priority. For example, if the following jobs are read: 3 9 2 8 1 9 3 5 1 4 2 12 3 10 2 7 1 5 3 7 The output of the program should be: The print jobs in the order of their priorities are: 1 5 1 4 1 9 2 7 2 12 2 8 3 10 3 5 3 9 3 7 class JobReport { public static void main(String [] args){ // Define an array of PrintJob // Read jobs until a specific key is pressed // Sorts the array by calling the method sort() // Print the jobs in the order of priority } }
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
A company office processes print jobs using a priority status. The class PrintJob describes the job using the data member priority to rank the job and data member time to measure its length. When comparing two jobs, the one with the lower value for priority is considered the most important.
Complete the PrintJob class definition (Note: You cannot change anything for the class except adding code to complete the definition of its methods and constructor)
class PrintJob {
private int priority;
private int time;
PrintJob(int pty, int t) { // constructor
}
int getPriority ( ) { // return the priority value of the job
}
int getTime( ) { // return the length of the job
}
void setPrintData(int pty, int t) { // Reset the priority and length of the job
}
}
Create a JobSort class that contains only a static method sort(PrintJob pj[], int n). The sort method will sort the PrintJob objects pj[] in ascending order based the job priority.
class JobSort {
static void sort(PrintJob pj[], int n) {
}
}
Write a class JobReport including a main method that:
(1) Defines a PrintJob array and necessary variables
(2) Reads job’s priority and time from keyboard, and stores them as an item (PrintJob object) in the array
(3) Repeat (2) for more items until user presses a specific key to stop it
(4) Sorts the array by calling the method sort()
(5) Print the jobs stored in the array in their order of priority.
For example, if the following jobs are read:
3 9
2 8
1 9
3 5
1 4
2 12
3 10
2 7
1 5
3 7
The output of the program should be:
The print jobs in the order of their priorities are:
1 5
1 4
1 9
2 7
2 12
2 8
3 10
3 5
3 9
3 7
class JobReport {
public static void main(String [] args){
// Define an array of PrintJob
// Read jobs until a specific key is pressed
// Sorts the array by calling the method sort()
// Print the jobs in the order of priority
}
}

Step by step
Solved in 3 steps with 1 images

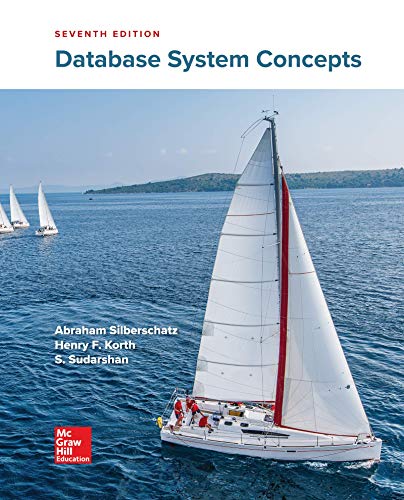
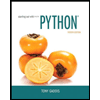
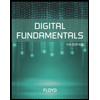
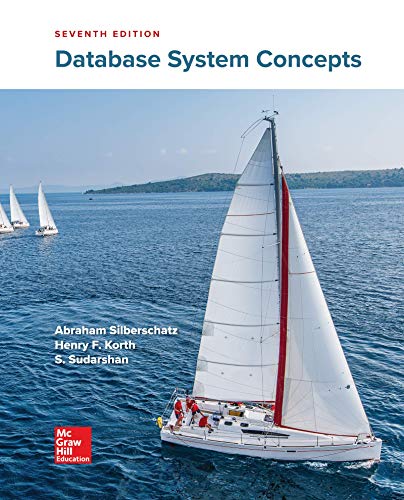
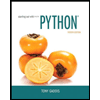
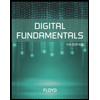
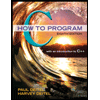
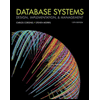
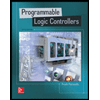