(a) A default constructor. (b) A constructor that takes a C string as argument. The characters of the constructed string should be copies of the characters of the C string. (c) The method length (). (d) An indexing operator. (e) The method c_str(). (This method should return a pointer to a C string that belongs to the String object.) 1 The easiest way to implement this class is to use an STL vector to store the characters of the string. But for the purposes of this assignment, you are not allowed to do that. Instead, you have to directly use a dynamically allocated array
(a) A default constructor. (b) A constructor that takes a C string as argument. The characters of the constructed string should be copies of the characters of the C string. (c) The method length (). (d) An indexing operator. (e) The method c_str(). (This method should return a pointer to a C string that belongs to the String object.) 1 The easiest way to implement this class is to use an STL vector to store the characters of the string. But for the purposes of this assignment, you are not allowed to do that. Instead, you have to directly use a dynamically allocated array
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
Attached is the question.
C++
Create a class String similar to the standard class string. Include the following operations:

Transcribed Image Text:### Assignment Requirements for String Class Implementation
This assignment involves creating a custom `String` class in C++. Your implementation should include the following functionalities:
1. **Default Constructor**:
- Implement a default constructor for the `String` class.
2. **Constructor with C String Argument**:
- Implement a constructor that accepts a C string (null-terminated char array) as an argument.
- The characters of the constructed `String` object should be copies of the characters from the C string.
3. **Length Method**:
- Implement a method named `length()` that returns the length of the string.
4. **Indexing Operator**:
- Implement an indexing operator to access individual characters of the `String`.
5. **C_String Method**:
- Implement a method named `c_str()` that returns a pointer to a C string that belongs to the `String` object.
**Note**:
- The easiest way to implement this class is by using an STL vector to store the characters of the string. However, for the purposes of this assignment, **you are not allowed to do that**. Instead, you must directly use a dynamically allocated array.
### Additional Information:
- You must manage memory dynamically and ensure no memory leaks occur.
- Test your class thoroughly to ensure all functionalities work as expected.
The purpose of this assignment is to deepen your understanding of dynamic memory management, constructors, destructors, and operator overloading in C++.
### Summary
- **(a)** Default Constructor
- **(b)** Constructor with C String Argument (Deep Copy)
- **(c)** `length()` Method
- **(d)** Indexing Operator
- **(e)** `c_str()` Method (Returns C String Pointer)
**Implementation Constraint**: Do **not** use STL vectors; use dynamically allocated array instead.
Good luck with your implementation!
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
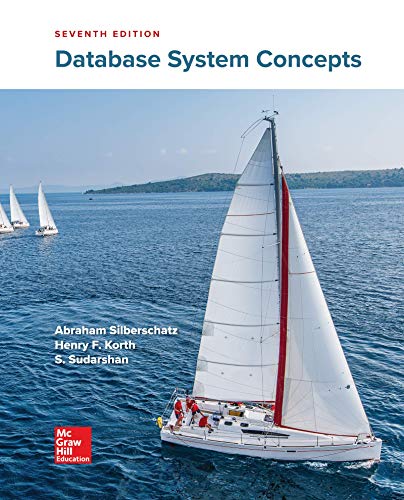
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
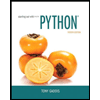
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
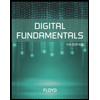
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
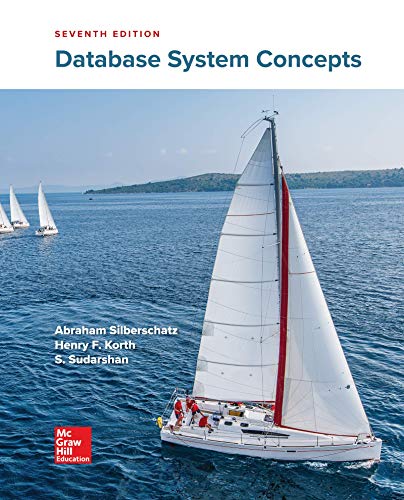
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
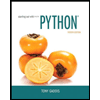
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
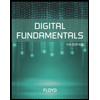
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
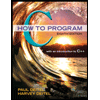
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
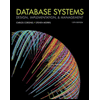
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
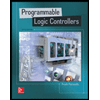
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education