8. Write function makeMove to do the following d. Return type void e. Parameter list includes i. Character array for the player's name (i.e., playerName, size is 20, use macro NAME) ii. 2-d character array (i.e., board), size 6 rows and 7 columns (i.e., use macros ROW and COL) f. Declare variable to store the user input for their move, data type character array, size 2 (i.e., move, use macro TWO) g. Declare variable to store if player move is valid, data type integer, initialize to 0 (i.e., valid, use macro FALSE) Loop while the player's move input is not valid (i.e., FALSE) h. i. Write a printf statement to prompt the player to enter their move ii. Write a scanf statement to store the player's move in local variable move iii. Write a printf statement to display to the player the move they entered iv. Declare variable to store the length of the player's 5 move input, data type integer (i.e., length) set equal to explicit type cast to integer of return value from function call strlen(), pass as an argument variable move v. Evaluate variable length 1. If it is equal to 1, set variable valid equal to true (i.e., use macros ONE and TRUE) 2. If it is not equal to 1, set variable valid equal to false (i.e., use macros ONE and FALSE vi. Evaluate variable valid, if it is false, write a printf statement to notify the player their move input was not valid (i.e., use macro FALSE)
8. Write function makeMove to do the following d. Return type void e. Parameter list includes i. Character array for the player's name (i.e., playerName, size is 20, use macro NAME) ii. 2-d character array (i.e., board), size 6 rows and 7 columns (i.e., use macros ROW and COL) f. Declare variable to store the user input for their move, data type character array, size 2 (i.e., move, use macro TWO) g. Declare variable to store if player move is valid, data type integer, initialize to 0 (i.e., valid, use macro FALSE) Loop while the player's move input is not valid (i.e., FALSE) h. i. Write a printf statement to prompt the player to enter their move ii. Write a scanf statement to store the player's move in local variable move iii. Write a printf statement to display to the player the move they entered iv. Declare variable to store the length of the player's 5 move input, data type integer (i.e., length) set equal to explicit type cast to integer of return value from function call strlen(), pass as an argument variable move v. Evaluate variable length 1. If it is equal to 1, set variable valid equal to true (i.e., use macros ONE and TRUE) 2. If it is not equal to 1, set variable valid equal to false (i.e., use macros ONE and FALSE vi. Evaluate variable valid, if it is false, write a printf statement to notify the player their move input was not valid (i.e., use macro FALSE)
Programming Logic & Design Comprehensive
9th Edition
ISBN:9781337669405
Author:FARRELL
Publisher:FARRELL
Chapter8: Advanced Data Handling Concepts
Section: Chapter Questions
Problem 7PE
Related questions
Question
I need assistance I tried to solve number 8 as well but my code still doesn't run please help

Transcribed Image Text:8. Write function makeMove to do the following
d. Return type void
e. Parameter list includes
i. Character array for the player's name (i.e.,
playerName, size is 20, use macro NAME)
ii. 2-d character array (i.e., board), size 6 rows and 7
columns (i.e., use macros ROW and COL)
f. Declare variable to store the user input for their move,
data type character array, size 2 (i.e., move, use macro
TWO)
g. Declare variable to store if player move is valid, data type
integer, initialize to 0 (i.e., valid, use macro FALSE)
h. Loop while the player's move input is not valid (i.e.,
FALSE)
i. Write a printf statement to prompt the player to
enter their move
ii.
Write a scanf statement to store the player's move
in local variable move
iii. Write a printf statement to display to the player
the move they entered
iv. Declare variable to store the length of the player's
move input, data type integer (i.e., length) set
equal to explicit type cast to integer of return value
from function call strlen(), pass as an argument
variable move
v. Evaluate variable length
1. If it is equal to 1, set variable valid equal to
true (i.e., use macros ONE and TRUE)
2. If it is not equal to 1, set variable valid
equal to false (i.e., use macros ONE and
FALSE)
vi. Evaluate variable valid, if it is false, write a printf
statement to notify the player their move input was
not valid (i.e., use macro FALSE)
![for (int i =0; i <ROW; i++)
{
}
for (int j = 0; j < COL; j++)
{
printf("%c ", board[i][j]);
}
}
void initializeBoard (char board [ROW] [COL])
{
}
printf("\n");
for (int i = 0; i < ROW; i++)
{
for (int j =0; j < COL; j++)
{
board [i][j] = SPACE;
}
}
void makeMove (char playerName [NAME], char board [ROW] [COL])
{
// Implement what ??
}
// stubbing out a function
void playGame ()
{
// Variables
char yellow [NAME];
char red [NAME];
int currentPlayer = YELLOW;
int loop = ZERO;
// Declare board array
char board [ROW] [COL];
// Ask Player Yellow to enter a name
printf ("Player Yellow, Enter your name\n");
scanf ("%s", yellow);
// Ask Player Red to enter a name
printf ("Player Red, Enter your name\n");
scanf ("%s", red);
printf("%s and %s let's play Connect Four!\n", yellow, red);
}
// InitializeBoard
initializeBoard (board);
// While for game loop
while (loop < FOUR)
{
// Board for each turn
displayBoard (board);
// Replace printf with makeMove
makeMove((currentPlayer == YELLOW)? yellow red, board);
currentPlayer = (currentPlayer ==YELLOW) ? RED: YELLOW;
loop++;
if (currentPlayer == YELLOW)
{
printf("Make a move");
currentPlayer = RED;
}
else if (currentPlayer == RED)
{
}
printf("%s, it is your turn\n", red);
currentPlayer = YELLOW;
loop++;](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F60a19010-4e8f-4f2f-9c4a-c1e2addd892b%2F62f55abf-bdaa-497e-a50f-0767ed792cef%2Ftv2ntp_processed.png&w=3840&q=75)
Transcribed Image Text:for (int i =0; i <ROW; i++)
{
}
for (int j = 0; j < COL; j++)
{
printf("%c ", board[i][j]);
}
}
void initializeBoard (char board [ROW] [COL])
{
}
printf("\n");
for (int i = 0; i < ROW; i++)
{
for (int j =0; j < COL; j++)
{
board [i][j] = SPACE;
}
}
void makeMove (char playerName [NAME], char board [ROW] [COL])
{
// Implement what ??
}
// stubbing out a function
void playGame ()
{
// Variables
char yellow [NAME];
char red [NAME];
int currentPlayer = YELLOW;
int loop = ZERO;
// Declare board array
char board [ROW] [COL];
// Ask Player Yellow to enter a name
printf ("Player Yellow, Enter your name\n");
scanf ("%s", yellow);
// Ask Player Red to enter a name
printf ("Player Red, Enter your name\n");
scanf ("%s", red);
printf("%s and %s let's play Connect Four!\n", yellow, red);
}
// InitializeBoard
initializeBoard (board);
// While for game loop
while (loop < FOUR)
{
// Board for each turn
displayBoard (board);
// Replace printf with makeMove
makeMove((currentPlayer == YELLOW)? yellow red, board);
currentPlayer = (currentPlayer ==YELLOW) ? RED: YELLOW;
loop++;
if (currentPlayer == YELLOW)
{
printf("Make a move");
currentPlayer = RED;
}
else if (currentPlayer == RED)
{
}
printf("%s, it is your turn\n", red);
currentPlayer = YELLOW;
loop++;
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
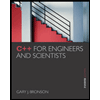
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
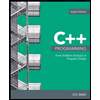
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
Programming Logic & Design Comprehensive
Computer Science
ISBN:
9781337669405
Author:
FARRELL
Publisher:
Cengage
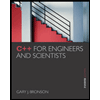
C++ for Engineers and Scientists
Computer Science
ISBN:
9781133187844
Author:
Bronson, Gary J.
Publisher:
Course Technology Ptr
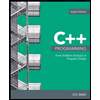
C++ Programming: From Problem Analysis to Program…
Computer Science
ISBN:
9781337102087
Author:
D. S. Malik
Publisher:
Cengage Learning
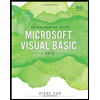
Programming with Microsoft Visual Basic 2017
Computer Science
ISBN:
9781337102124
Author:
Diane Zak
Publisher:
Cengage Learning
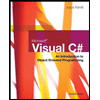
Microsoft Visual C#
Computer Science
ISBN:
9781337102100
Author:
Joyce, Farrell.
Publisher:
Cengage Learning,
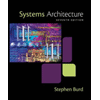
Systems Architecture
Computer Science
ISBN:
9781305080195
Author:
Stephen D. Burd
Publisher:
Cengage Learning