7.24 LAB: Car value (classes) Given main(), complete the Car class (in file Car.java) with methods to set and get the purchase price of a car (setPurchasePrice(), getPurchasePrice()), and to output the car's information (printInfo()). Ex: If the input is: 2011 18000 2018 where 2011 is the car's model year, 18000 is the purchase price, and 2018 is the current year, the output is: Car's information: Model year: 2011 Purchase price: $18000 Current value: $5770 Note: printInfo() should use two spaces for indentation. solution: import java.util.Scanner; import java.lang.Math; public class CarValue { public static void main(String[] args) { Scanner scnr = new Scanner(System.in); Car myCar = new Car(); int userYear = scnr.nextInt(); int userPrice = scnr.nextInt(); int userCurrentYear = scnr.nextInt(); myCar.setModelYear(userYear); myCar.setPurchasePrice(userPrice); myCar.calcCurrentValue(userCurrentYear); myCar.printInfo(); } } it says my solutions is missing $ and other things
7.24 LAB: Car value (classes)
Given main(), complete the Car class (in file Car.java) with methods to set and get the purchase price of a car (setPurchasePrice(), getPurchasePrice()), and to output the car's information (printInfo()).
Ex: If the input is:
2011 18000 2018where 2011 is the car's model year, 18000 is the purchase price, and 2018 is the current year, the output is:
Car's information: Model year: 2011 Purchase price: $18000 Current value: $5770Note: printInfo() should use two spaces for indentation.
solution:
import java.util.Scanner;
import java.lang.Math;
public class CarValue {
public static void main(String[] args) {
Scanner scnr = new Scanner(System.in);
Car myCar = new Car();
int userYear = scnr.nextInt();
int userPrice = scnr.nextInt();
int userCurrentYear = scnr.nextInt();
myCar.setModelYear(userYear);
myCar.setPurchasePrice(userPrice);
myCar.calcCurrentValue(userCurrentYear);
myCar.printInfo();
}
}
it says my solutions is missing $ and other things
![Bb My Syllabus X Bb 88276839 × Bb 88276840 X Bb Chapter 1 × Bb 39437066 X
learn.zybooks.com/zybook/CUNYCST1201WuFall2022/chapter/7/section/24
eeeeeeeeeeeeeee...
bible
[Solved] 7.24 L....html
eTOTALS | Welco...
how much does it...
Your output
=zyBooks My library > CST 1201: Programming Fundamentals home > 7.24: LAB: Car value (classes)
Model year: 1995
Purchase price: 32000
Current value: 550
Expected output
5:Unit test ^
5 Common Encryp...
Test feedback
165576779578....jpeg
Car's information:
Model year: 1995
Purchase price: $32000
Current value: $550
zy Section 7.24 X
Car's information:
Model year: 2015
Activity summary for assignment: Lab 7. 21 -7.28
Due: 12/25/2022, 11:59 PM EST
Purchase price: 35000
Current value: 13200
Free Grammar Ch...
1655767777314....jpeg
Tests printInfo() outputs information for car with model Year = 2015, purchase Price = 35000, and currentYear = 2021.
printInfo() method incorrectly outputted:
python - cst ×
Success Co X
Exact Change wit...
Search resu X
EzyBooks catalog
0/2
(56) reddit. X
Feedback?
? Help/FAQ 8 Justin Bowdoin
h
14 / 60 pts
☐ J
Show All
>
X](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F05a24295-2424-4b2b-bc74-9d8e0f4c7622%2F0c049190-6e2a-4660-9a6e-4be96383af1a%2Fa66nsdp_processed.png&w=3840&q=75)


Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 3 images

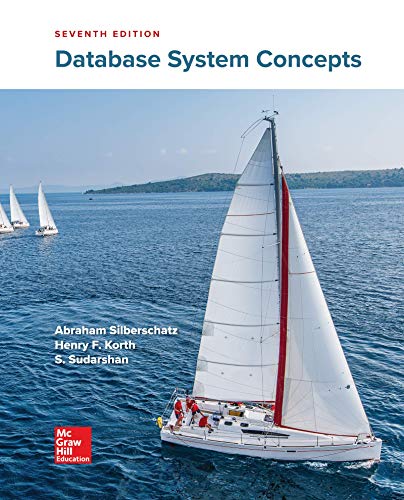
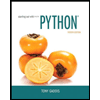
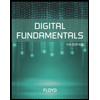
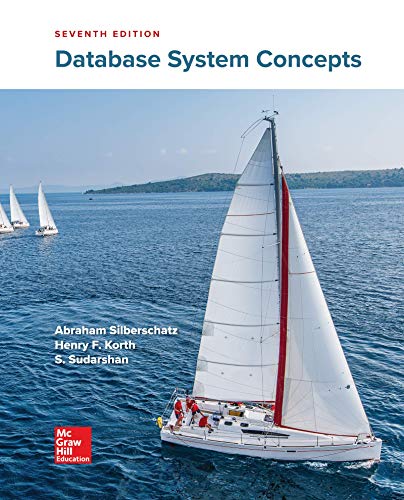
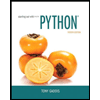
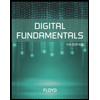
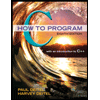
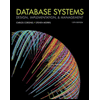
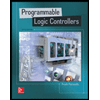