6.8 Run the test bench of Program 6-6 for the 2-to-4 decoder module Vr2to4dec_s in Program 6-1, showing that there are no errors. Then do three more runs, each time inserting or deleting just one character in Vr2to4dec_s, resulting in the test bench reporting 2, 4, and 8 errors.
6.8 Run the test bench of Program 6-6 for the 2-to-4 decoder module Vr2to4dec_s in Program 6-1, showing that there are no errors. Then do three more runs, each time inserting or deleting just one character in Vr2to4dec_s, resulting in the test bench reporting 2, 4, and 8 errors.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
6.8 Run the test bench of Program 6-6 for the 2-to-4 decoder module Vr2to4dec_s in Program 6-1, showing that there are no errors. Then do three more runs, each time inserting or deleting just one character in Vr2to4dec_s, resulting in the test bench reporting 2, 4, and 8 errors.
![```verilog
Program 6-6 Test bench for a 2-to-4 decoder.
`timescale 1 ns / 100 ps
module Vr2to4dec_tb ();
reg A0, A1, ENs;
wire Y0s, Y1s, Y2s, Y3s;
integer i, errors;
reg [3:0] expectY;
Vr2to4dec_s UUT (.A0(A0s),.A1(A1s),.EN(ENs), // Instantiate
.Y0(Y0s),.Y1(Y1s),.Y2(Y2s),.Y3(Y3s) );
initial begin
errors = 0;
for (i=0; i<=7; i=i+1) begin // Apply test in
{ENs, A1s, A0s} = i;
#10
expectY = 4'b0000; // Expect no out
if (ENs==1) expectY[{A1s,A0s}] = 1'b1; // Else output
if ({Y3s,Y2s,Y1s,Y0s} !== expectY) begin
$display("Error: EN A1A0 = %b%b%b, Y3Y2Y1Y0 = %b%b%b%b",
ENs, A1s, A0s, Y3s, Y2s, Y1s, Y0s);
errors = errors + 1;
end
end
$display("Test complete, %d errors",errors);
end
endmodule
```
**Explanation:**
This Verilog code is a test bench for a 2-to-4 decoder. It tests the decoder by applying different combinations of inputs and comparing the outputs with expected results. Here's a breakdown of the key components:
- **Module Declaration:** The `Vr2to4dec_tb` module declares three registers (`A0`, `A1`, `ENs`) and four wires (`Y0s`, `Y1s`, `Y2s`, `Y3s`).
- **Integer and Register Declaration:** An integer `i` and `errors` are used for iteration and error tracking, respectively. A 4-bit register `expectY` is used to store expected output](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2Fbbfc8250-1df3-458c-8274-8bf80d6812bf%2F40bd5282-2527-483e-9b94-1e895227f6b3%2F5iyidsa_processed.png&w=3840&q=75)
Transcribed Image Text:```verilog
Program 6-6 Test bench for a 2-to-4 decoder.
`timescale 1 ns / 100 ps
module Vr2to4dec_tb ();
reg A0, A1, ENs;
wire Y0s, Y1s, Y2s, Y3s;
integer i, errors;
reg [3:0] expectY;
Vr2to4dec_s UUT (.A0(A0s),.A1(A1s),.EN(ENs), // Instantiate
.Y0(Y0s),.Y1(Y1s),.Y2(Y2s),.Y3(Y3s) );
initial begin
errors = 0;
for (i=0; i<=7; i=i+1) begin // Apply test in
{ENs, A1s, A0s} = i;
#10
expectY = 4'b0000; // Expect no out
if (ENs==1) expectY[{A1s,A0s}] = 1'b1; // Else output
if ({Y3s,Y2s,Y1s,Y0s} !== expectY) begin
$display("Error: EN A1A0 = %b%b%b, Y3Y2Y1Y0 = %b%b%b%b",
ENs, A1s, A0s, Y3s, Y2s, Y1s, Y0s);
errors = errors + 1;
end
end
$display("Test complete, %d errors",errors);
end
endmodule
```
**Explanation:**
This Verilog code is a test bench for a 2-to-4 decoder. It tests the decoder by applying different combinations of inputs and comparing the outputs with expected results. Here's a breakdown of the key components:
- **Module Declaration:** The `Vr2to4dec_tb` module declares three registers (`A0`, `A1`, `ENs`) and four wires (`Y0s`, `Y1s`, `Y2s`, `Y3s`).
- **Integer and Register Declaration:** An integer `i` and `errors` are used for iteration and error tracking, respectively. A 4-bit register `expectY` is used to store expected output

Transcribed Image Text:**Program 6-1: Structural-style Verilog Module for the Decoder**
This Verilog program defines a structural-style module for a 2-to-4 decoder with enable. It is a part of the educational material related to Figure 6-15.
### Module Definition
```verilog
module Vr2to4dec_s(A0, A1, EN, Y0, Y1, Y2, Y3);
input A0, A1, EN;
output Y0, Y1, Y2, Y3;
wire NOTA0, NOTA1;
not U1 (NOTA0, A0);
not U2 (NOTA1, A1);
and U3 (Y0, NOTA0, NOTA1, EN);
and U4 (Y1, A0, NOTA1, EN);
and U5 (Y2, NOTA0, A1, EN);
and U6 (Y3, A0, A1, EN);
endmodule
```
### Explanation
- **Inputs:**
- `A0`, `A1`: Two input signals for the decoder.
- `EN`: Enable signal that activates the decoder.
- **Outputs:**
- `Y0`, `Y1`, `Y2`, `Y3`: Four output signals.
- **Wires:**
- `NOTA0`, `NOTA1`: Internal wires that hold the inverted values of `A0` and `A1`.
- **Logic Gates:**
- Two NOT gates (`U1` and `U2`) invert the `A0` and `A1` signals respectively.
- Four AND gates (`U3`, `U4`, `U5`, `U6`) produce the outputs based on the combinations of the inputs and the enable signal.
This module demonstrates how basic logic gate components can be connected in a structural style to construct a more complex digital circuit like a decoder.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
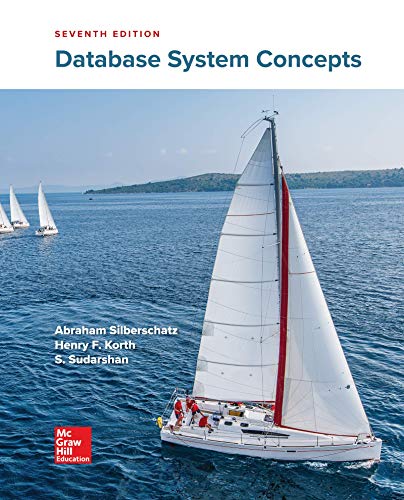
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
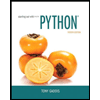
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
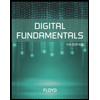
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
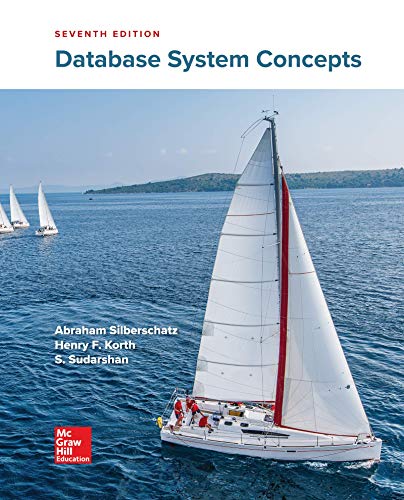
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
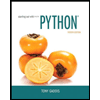
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
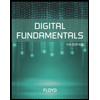
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
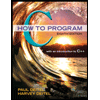
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
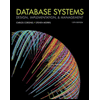
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
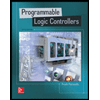
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education