5.13 LAB: Password modifier Many user-created passwords are simple and easy to guess. Write a program that takes a simple password and makes it stronger by replacing characters using the key below, and by appending "!" to the end of the input string. i becomes 1 a becomes @ m becomes M B becomes 8 s becomes $ Ex: If the input is: mypassword the output is: Myp@$$word! Hint: Python strings are immutable, but support string concatenation. Store and build the stronger password in the given password variable. my code: please help me word = input() password = '' # Modify characters i, a, m, B, and o in password for character in word: if character == 'i': # IF character equals 'i' change to '!' password += '!' elif character == 'a': # ELSE IF character equals 'a' change to '@' password += '@' elif character == 'm': # ELSE IF character equals 'm' change to 'M' password += 'M' elif character == 'B': # ELSE IF character equals 'B' change to '8' password += '8' elif character == 'o': # ELSE IF character equals 'o' change to '.' password += '.' else: # When symbols do not get replaced password += character print(password + 'q*s') # Make password stronger by adding "q*s" to the end of # the input string
5.13 LAB: Password modifier
Many user-created passwords are simple and easy to guess. Write a program that takes a simple password and makes it stronger by replacing characters using the key below, and by appending "!" to the end of the input string.
- i becomes 1
- a becomes @
- m becomes M
- B becomes 8
- s becomes $
Ex: If the input is:
mypasswordthe output is:
Myp@$$word!Hint: Python strings are immutable, but support string concatenation. Store and build the stronger password in the given password variable.
my code: please help me
word = input() password = ''
# Modify characters i, a, m, B, and o in password
for character in word:
if character == 'i': # IF character equals 'i' change to '!'
password += '!'
elif character == 'a': # ELSE IF character equals 'a' change to '@'
password += '@'
elif character == 'm': # ELSE IF character equals 'm' change to 'M'
password += 'M'
elif character == 'B': # ELSE IF character equals 'B' change to '8'
password += '8'
elif character == 'o': # ELSE IF character equals 'o' change to '.'
password += '.'
else: # When symbols do not get replaced
password += character
print(password + 'q*s') # Make password stronger by adding "q*s" to the end of
# the input string


Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

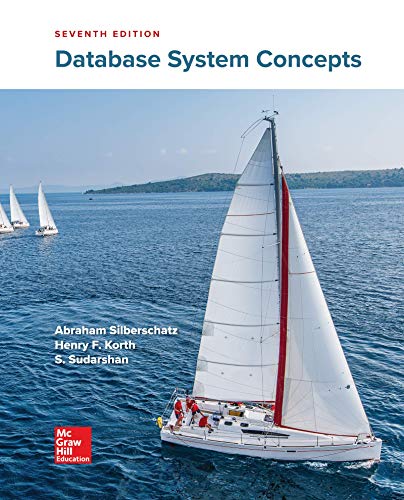
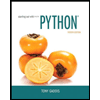
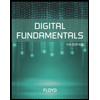
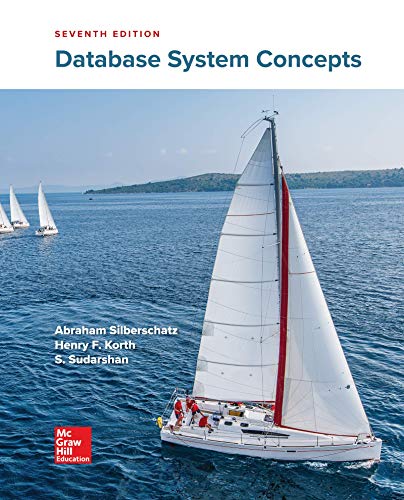
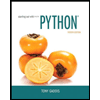
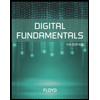
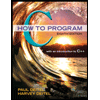
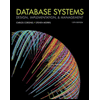
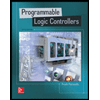