4. Using the divided difference method, print out the Hermite polynomial approximation matrix X 3.6 3.8 3.9 f(x) 1.675 1.436 1.318 f'(x) -1.195 -1.188 -1.182
Please help me solve this in python. Add the code used to solve it.
The expected output is:
[[ 3.6 1.675 0. 0. 0. 0. ]
[ 3.6 1.675 -1.195 0. 0. 0. ]
[ 3.8 1.436 -1.195 -0. 0. 0. ]
[ 3.8 1.436 -1.188 0.035 0.175 0. ]
[ 3.9 1.318 -1.18 0.08 0.15 -0.0833333]
[ 3.9 1.318 -1.182 -0.02 -1. -3.8333333]]


Here is a python code to solve the problem
import numpy as np
def divided_difference(x, y, y_prime):
n = len(x)
f = np.zeros((n, n))
f[:, 0] = y
f[:, 1] = y_prime
for j in range(2, n):
for i in range(n-j+1):
f[i, j] = (f[i+1, j-1] - f[i, j-1]) / (x[i+j-1] - x[i])
return f
x = [3.6, 3.8, 3.9]
y = [1.675, 1.436, 1.318]
y_prime = [-1.195, -1.188, -1.182]
approximation_matrix = divided_difference(x, y, y_prime)
print(approximation_matrix)
Trending now
This is a popular solution!
Step by step
Solved in 2 steps

This is the ouput i got when i ran the code:
[[ 1.675 -1.195 0.035]
[ 1.436 -1.188 0.06 ]
[ 1.318 -1.182 0. ]]
But it needs to be:
[[ 3.6 1.675 0. 0. 0. 0. ]
[ 3.6 1.675 -1.195 0. 0. 0. ]
[ 3.8 1.436 -1.195 -0. 0. 0. ]
[ 3.8 1.436 -1.188 0.035 0.175 0. ]
[ 3.9 1.318 -1.18 0.08 0.15 -0.0833333]
[ 3.9 1.318 -1.182 -0.02 -1. -3.8333333]]
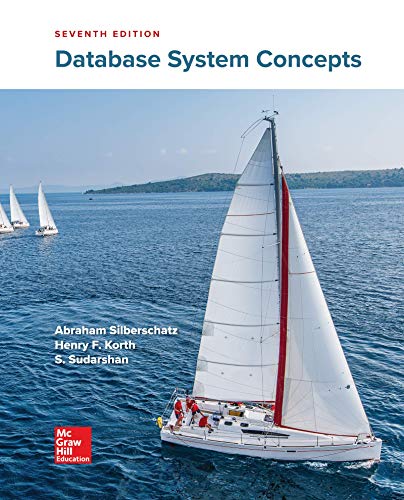
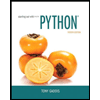
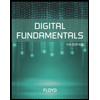
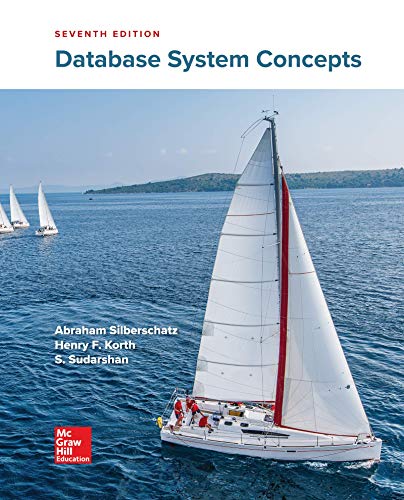
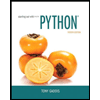
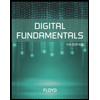
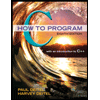
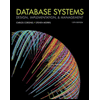
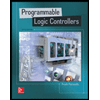