23.4 LAB: Student class (Use Python) In main.py define the Student class that has two attributes: name and gpa Implement the following instance methods: A constructor that sets name to "Louie" and gpa to 1.0 set_name(self, name) - set student's name to parameter name get_name(self) - return student's name set_gpa(self, gpa) - set student's gpa to parameter gpa get_gpa(self) - return student's gpa Ex. If a new Student object is created, the default output is: Louie/1.0 Ex. If the student's name is set to "Felix" and the gpa is set to 3.7, the output becomes: Felix/3.7
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
23.4 LAB: Student class (Use Python)
In main.py define the Student class that has two attributes: name and gpa
Implement the following instance methods:
- A constructor that sets name to "Louie" and gpa to 1.0
- set_name(self, name) - set student's name to parameter name
- get_name(self) - return student's name
- set_gpa(self, gpa) - set student's gpa to parameter gpa
- get_gpa(self) - return student's gpa
Ex. If a new Student object is created, the default output is:
Louie/1.0
Ex. If the student's name is set to "Felix" and the gpa is set to 3.7, the output becomes:
Felix/3.7


Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

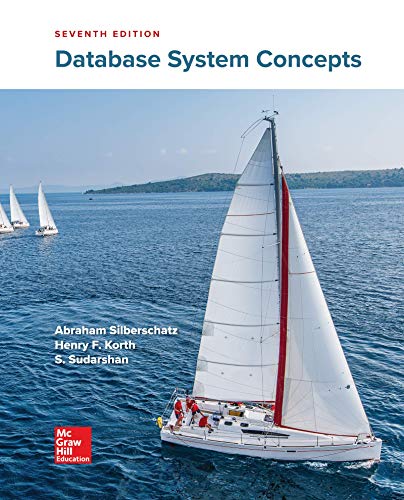
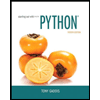
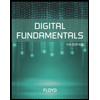
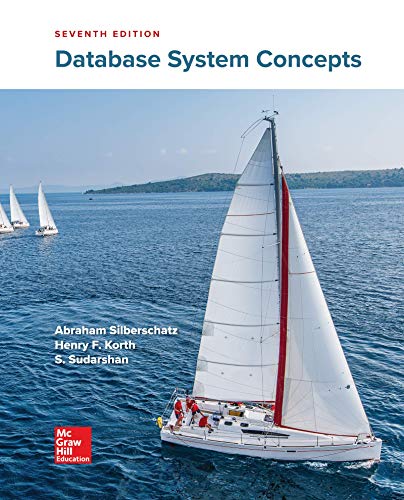
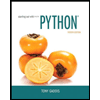
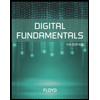
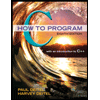
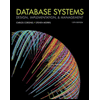
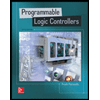