2.Create an employee class, basing it on Task 1. The member data should comprise an int for storing the employee number and a float for storing the employee’s compensation. Member functions should allow the user to enter this data and display it. Write a main() that allows the user to enter data for three employees and display it. -----------------------------------------------------------------------------------so this is my output code from task 1, please help me with this question #include using namespace std; //create the structure employee struct employee { //declaring integer type variable number int number; //declaring float type variable compensation float compensation; }; //defining the main method int main() { employee e1, e2, e3; //ask the user to enter employee number of first employee cout << "Enter employee number of first employee: "; //read the value cin >> e1.number; //ask the user to enter compensation of first employee cout << "Enter compensation of first employee: "; //read the value cin >> e1.compensation; //ask the user to enter employee number of second employee cout << "Enter employee number of second employee: "; //read the value cin >> e2.number; //ask the user to enter compensation of second employee cout << "Enter compensation of second employee: "; //read the value cin >> e2.compensation; //ask the user to enter employee number of third employee cout << "Enter employee number of third employee: "; //read the value cin >> e3.number; //ask the user to enter compensation of third employee cout << "Enter compensation of third employee: "; //read the value cin >> e3.compensation; //display the message cout << "Employee 1:" << endl; cout << "Employee number: " << e1.number << endl; cout << "Compensation: " << e1.compensation << endl << endl; cout << "Employee 2:" << endl; cout << "Employee n33umber: " << e2.number << endl; cout << "Compensation: " << e2.compensation << endl << endl; cout << "Employee 3:" << endl; cout << "Employee number: " << e3.number << endl; cout << "Compensation: " << e3.compensation << endl << endl; //return the value return 0; }
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
2.Create an employee class, basing it on Task 1. The member data should comprise an int for storing the employee number and a float for storing the employee’s compensation. Member functions should allow the user to enter this data and display it. Write a main() that allows the user to enter data for three employees and display it.
-----------------------------------------------------------------------------------so this is my output code from task 1, please help me with this question
#include <iostream>
using namespace std;
//create the structure employee
struct employee {
//declaring integer type variable number
int number;
//declaring float type variable compensation
float compensation;
};
//defining the main method
int main() {
employee e1, e2, e3;
//ask the user to enter employee number of first employee
cout << "Enter employee number of first employee: ";
//read the value
cin >> e1.number;
//ask the user to enter compensation of first employee
cout << "Enter compensation of first employee: ";
//read the value
cin >> e1.compensation;
//ask the user to enter employee number of second employee
cout << "Enter employee number of second employee: ";
//read the value
cin >> e2.number;
//ask the user to enter compensation of second employee
cout << "Enter compensation of second employee: ";
//read the value
cin >> e2.compensation;
//ask the user to enter employee number of third employee
cout << "Enter employee number of third employee: ";
//read the value
cin >> e3.number;
//ask the user to enter compensation of third employee
cout << "Enter compensation of third employee: ";
//read the value
cin >> e3.compensation;
//display the message
cout << "Employee 1:" << endl;
cout << "Employee number: " << e1.number << endl;
cout << "Compensation: " << e1.compensation << endl << endl;
cout << "Employee 2:" << endl;
cout << "Employee n33umber: " << e2.number << endl;
cout << "Compensation: " << e2.compensation << endl << endl;
cout << "Employee 3:" << endl;
cout << "Employee number: " << e3.number << endl;
cout << "Compensation: " << e3.compensation << endl << endl;
//return the value
return 0;
}

Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 2 images

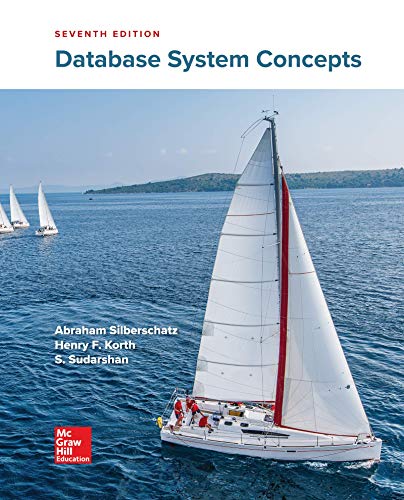
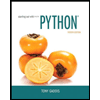
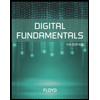
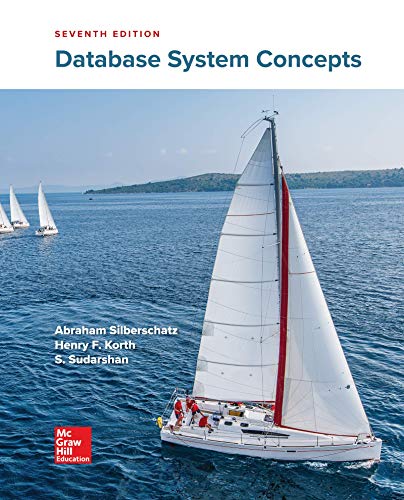
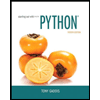
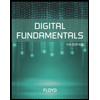
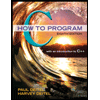
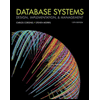
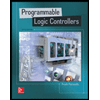