5. Matrix Operations Suppose now you put in a matrix and you wish to manually do row operations to it. MATLAB doesn't have any functions for doing row operations, instead we have to execute low-level commands to do so. We will use "array slicing." This is easier than it seems. Here is an augmented matrix >> A=[1-1 -37; -3 1 4 -16; 4-3 -5 12] To get this to row echelon form, first we'll clean out the entries below the upper-left 1. To do this we need to add 3 times row 1 to row 2. In MATLAB the code is >> A(2,:)=A(2,:)+3*A(1,:) Confused? I was! The expression A(rownumber, :) refers to the entire row, so basically this line is saying: A(2,:)=A(2,:)+3+A(1,:) Row 2 Row 2 3+Row1 Try it! The result will be given. Next we'll add -4 times row 1 to row 3. >> A(3,:)=A(3,:)+(-4)+A(1,:) Now you'll see that we have Os below the upper-left 1. Next we need to get a 0 in the bottom row where the 1 is. The easiest way to do this is first to interchange rows 2 and 3. >> A([23], :)=A([32], :) This is a confusing command but it does the job. Now we add twice row 2 to row 3. >> A(3,:) A (3,:)+2+A(2,:) The result you'll see is the matrix in row echelon form. Remember this means any rows of Os are at the bottom (none in this case) and the leading entries go down and right. To get to reduced row echelon form, we first have to get 1s for all our leading entries. The third row 2. Consider the system of equations 12x2 3x3 + 2x4 − - - x5=-7 -2x19x26x38x4+16x5 = 14 - -17x2+3x3 2x4 +115=-3 4x18x212x3 + 4x5=-8 (a) Enter it into MATLAB as an (augmented) matrix named B. (b) Use elementary row operations (as in part 5 of the guide) to reduce it to row echelon form. (c) Continue using elementary row operations to get to reduced row echelon form. Yes, you have to. (d) Re-enter the original matrix into MATLAB and use the rref command to instantly get the reduced row echelon form. Make sure it is the same as your previous answer. (From this point onward, we will make extensive use of the rref command in MATLAB. You do not need to do row reduction in MATLAB one elementary row operation at a time anymore.) (e) Give the solution of the system in parametric vector form. 3. Start this problem in format short. Consider the linear system whose augmented matrix is 2 2 -7 A = 3 1 -1 0 -6 6 -5 (a) Use the rref command to give the reduced row echelon form of A. Make sure your result in presented in decimals, not fractions. (b) Write the solution of the linear system which corresponds to A in decimals, not fractions. (Your "solution" is actually a decimal approximation to the solution.) (c) Give the reduced row echelon form of A in fractions rather than decimals. (d) Write the solution of the linear system which corresponds to A in fractions, not decimals.
5. Matrix Operations Suppose now you put in a matrix and you wish to manually do row operations to it. MATLAB doesn't have any functions for doing row operations, instead we have to execute low-level commands to do so. We will use "array slicing." This is easier than it seems. Here is an augmented matrix >> A=[1-1 -37; -3 1 4 -16; 4-3 -5 12] To get this to row echelon form, first we'll clean out the entries below the upper-left 1. To do this we need to add 3 times row 1 to row 2. In MATLAB the code is >> A(2,:)=A(2,:)+3*A(1,:) Confused? I was! The expression A(rownumber, :) refers to the entire row, so basically this line is saying: A(2,:)=A(2,:)+3+A(1,:) Row 2 Row 2 3+Row1 Try it! The result will be given. Next we'll add -4 times row 1 to row 3. >> A(3,:)=A(3,:)+(-4)+A(1,:) Now you'll see that we have Os below the upper-left 1. Next we need to get a 0 in the bottom row where the 1 is. The easiest way to do this is first to interchange rows 2 and 3. >> A([23], :)=A([32], :) This is a confusing command but it does the job. Now we add twice row 2 to row 3. >> A(3,:) A (3,:)+2+A(2,:) The result you'll see is the matrix in row echelon form. Remember this means any rows of Os are at the bottom (none in this case) and the leading entries go down and right. To get to reduced row echelon form, we first have to get 1s for all our leading entries. The third row 2. Consider the system of equations 12x2 3x3 + 2x4 − - - x5=-7 -2x19x26x38x4+16x5 = 14 - -17x2+3x3 2x4 +115=-3 4x18x212x3 + 4x5=-8 (a) Enter it into MATLAB as an (augmented) matrix named B. (b) Use elementary row operations (as in part 5 of the guide) to reduce it to row echelon form. (c) Continue using elementary row operations to get to reduced row echelon form. Yes, you have to. (d) Re-enter the original matrix into MATLAB and use the rref command to instantly get the reduced row echelon form. Make sure it is the same as your previous answer. (From this point onward, we will make extensive use of the rref command in MATLAB. You do not need to do row reduction in MATLAB one elementary row operation at a time anymore.) (e) Give the solution of the system in parametric vector form. 3. Start this problem in format short. Consider the linear system whose augmented matrix is 2 2 -7 A = 3 1 -1 0 -6 6 -5 (a) Use the rref command to give the reduced row echelon form of A. Make sure your result in presented in decimals, not fractions. (b) Write the solution of the linear system which corresponds to A in decimals, not fractions. (Your "solution" is actually a decimal approximation to the solution.) (c) Give the reduced row echelon form of A in fractions rather than decimals. (d) Write the solution of the linear system which corresponds to A in fractions, not decimals.
College Algebra (MindTap Course List)
12th Edition
ISBN:9781305652231
Author:R. David Gustafson, Jeff Hughes
Publisher:R. David Gustafson, Jeff Hughes
Chapter6: Linear Systems
Section6.2: Guassian Elimination And Matrix Methods
Problem 83E: Explain the difference between the row-echelon form and the reduced row-echelon form of a matrix.
Related questions
Question
MATLAB. Awnser written questions (*) in the comments. Null, Rank, and most functions outside of rref() and disp() are not allowed! Solutions must be given manually! Elementary form means to reduce to RREF manually, without rref(). Please see other attached image for explanation
![5. Matrix Operations
Suppose now you put in a matrix and you wish to manually do row operations to it. MATLAB doesn't
have any functions for doing row operations, instead we have to execute low-level commands to do so.
We will use "array slicing." This is easier than it seems.
Here is an augmented matrix
>> A=[1-1 -37; -3 1 4 -16; 4-3 -5 12]
To get this to row echelon form, first we'll clean out the entries below the upper-left 1. To do this we
need to add 3 times row 1 to row 2. In MATLAB the code is
>> A(2,:)=A(2,:)+3*A(1,:)
Confused? I was! The expression A(rownumber, :) refers to the entire row, so basically this line is
saying:
A(2,:)=A(2,:)+3+A(1,:)
Row 2
Row 2
3+Row1
Try it! The result will be given.
Next we'll add -4 times row 1 to row 3.
>> A(3,:)=A(3,:)+(-4)+A(1,:)
Now you'll see that we have Os below the upper-left 1. Next we need to get a 0 in the bottom row
where the 1 is. The easiest way to do this is first to interchange rows 2 and 3.
>> A([23], :)=A([32], :)
This is a confusing command but it does the job.
Now we add twice row 2 to row 3.
>> A(3,:) A (3,:)+2+A(2,:)
The result you'll see is the matrix in row echelon form. Remember this means any rows of Os are at
the bottom (none in this case) and the leading entries go down and right.
To get to reduced row echelon form, we first have to get 1s for all our leading entries. The third row](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F8394ca4d-f3a7-477b-8001-e6919ea106d5%2Fff618eca-81b2-4a54-a0ec-d7423fd354c5%2Fkbtonb5_processed.png&w=3840&q=75)
Transcribed Image Text:5. Matrix Operations
Suppose now you put in a matrix and you wish to manually do row operations to it. MATLAB doesn't
have any functions for doing row operations, instead we have to execute low-level commands to do so.
We will use "array slicing." This is easier than it seems.
Here is an augmented matrix
>> A=[1-1 -37; -3 1 4 -16; 4-3 -5 12]
To get this to row echelon form, first we'll clean out the entries below the upper-left 1. To do this we
need to add 3 times row 1 to row 2. In MATLAB the code is
>> A(2,:)=A(2,:)+3*A(1,:)
Confused? I was! The expression A(rownumber, :) refers to the entire row, so basically this line is
saying:
A(2,:)=A(2,:)+3+A(1,:)
Row 2
Row 2
3+Row1
Try it! The result will be given.
Next we'll add -4 times row 1 to row 3.
>> A(3,:)=A(3,:)+(-4)+A(1,:)
Now you'll see that we have Os below the upper-left 1. Next we need to get a 0 in the bottom row
where the 1 is. The easiest way to do this is first to interchange rows 2 and 3.
>> A([23], :)=A([32], :)
This is a confusing command but it does the job.
Now we add twice row 2 to row 3.
>> A(3,:) A (3,:)+2+A(2,:)
The result you'll see is the matrix in row echelon form. Remember this means any rows of Os are at
the bottom (none in this case) and the leading entries go down and right.
To get to reduced row echelon form, we first have to get 1s for all our leading entries. The third row

Transcribed Image Text:2. Consider the system of equations
12x2 3x3 + 2x4 −
-
-
x5=-7
-2x19x26x38x4+16x5 = 14
-
-17x2+3x3 2x4 +115=-3
4x18x212x3
+ 4x5=-8
(a) Enter it into MATLAB as an (augmented) matrix named B.
(b) Use elementary row operations (as in part 5 of the guide) to reduce it to row echelon form.
(c) Continue using elementary row operations to get to reduced row echelon form. Yes, you have to.
(d) Re-enter the original matrix into MATLAB and use the rref command to instantly get the
reduced row echelon form. Make sure it is the same as your previous answer. (From this point
onward, we will make extensive use of the rref command in MATLAB. You do not need to do
row reduction in MATLAB one elementary row operation at a time anymore.)
(e) Give the solution of the system in parametric vector form.
3. Start this problem in format short. Consider the linear system whose augmented matrix is
2 2 -7
A = 3 1 -1 0
-6 6
-5
(a) Use the rref command to give the reduced row echelon form of A. Make sure your result in
presented in decimals, not fractions.
(b) Write the solution of the linear system which corresponds to A in decimals, not fractions. (Your
"solution" is actually a decimal approximation to the solution.)
(c) Give the reduced row echelon form of A in fractions rather than decimals.
(d) Write the solution of the linear system which corresponds to A in fractions, not decimals.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps with 1 images

Recommended textbooks for you
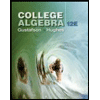
College Algebra (MindTap Course List)
Algebra
ISBN:
9781305652231
Author:
R. David Gustafson, Jeff Hughes
Publisher:
Cengage Learning
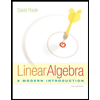
Linear Algebra: A Modern Introduction
Algebra
ISBN:
9781285463247
Author:
David Poole
Publisher:
Cengage Learning
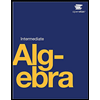
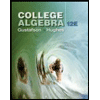
College Algebra (MindTap Course List)
Algebra
ISBN:
9781305652231
Author:
R. David Gustafson, Jeff Hughes
Publisher:
Cengage Learning
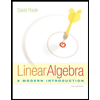
Linear Algebra: A Modern Introduction
Algebra
ISBN:
9781285463247
Author:
David Poole
Publisher:
Cengage Learning
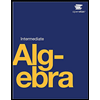
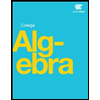
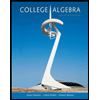
College Algebra
Algebra
ISBN:
9781305115545
Author:
James Stewart, Lothar Redlin, Saleem Watson
Publisher:
Cengage Learning
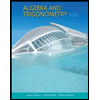
Algebra and Trigonometry (MindTap Course List)
Algebra
ISBN:
9781305071742
Author:
James Stewart, Lothar Redlin, Saleem Watson
Publisher:
Cengage Learning