15.14 LAB: Binary search Binary search can be implemented as a recursive algorithm. Each call makes a recursive call on one-half of the list the call received as an argument. Complete the recursive function BinarySearch() with the following specifications: Parameters: a target integer a vector of integers lower and upper bounds within which the recursive call will search Return value: the index within the vector where the target is located -1 if target is not found The template provides the main program and a helper function that reads a vector from input. The algorithm begins by choosing an index midway between the lower and upper bounds. If target == integers.at(index) return index If lower == upper, return -1 to indicate not found Otherwise call the function recursively on half the vector parameter: If integers.at(index) < target, search the vector from index + 1 to upper If integers.at(index) > target, search the vector from lower to index - 1 The vector must be ordered, but duplicates are allowed. Once the search algorithm works correctly, add the following to BinarySearch(): Count the number of calls to BinarySearch(). Count the number of times when the target is compared to an element of the vector. Note: lower == upper should not be counted. Hint: Use a global variable to count calls and comparisons. The input of the program consists of: the number of integers in the vector the integers in the vector the target to be located Ex: If the input is: 9 1 2 3 4 5 6 7 8 9 2 the output is: index: 1, recursions: 2, comparisons: 3 ATTENTION WHERE IT SAYS CODE HERE, IS WHERE I MUST ADD CODE. EVERYTHING ELSE CANNOT BE ALTERED #include #include #include using namespace std; // Read integers from input and store them in a vector. // Return the vector. vector ReadIntegers() { int size; cin >> size; vector integers(size); for (int i = 0; i < size; ++i) { // Read the numbers cin >> integers.at(i); } sort(integers.begin(), integers.end()); return integers; } int BinarySearch(int target, vector integers, int lower, int upper) { /* code here. */ } int main() { int target; int index; vector integers = ReadIntegers(); cin >> target; index = BinarySearch(target, integers, 0, integers.size() - 1); printf("index: %d, recursions: %d, comparisons: %d\n", index, recursions, comparisons); return 0; }
Types of Linked List
A sequence of data elements connected through links is called a linked list (LL). The elements of a linked list are nodes containing data and a reference to the next node in the list. In a linked list, the elements are stored in a non-contiguous manner and the linear order in maintained by means of a pointer associated with each node in the list which is used to point to the subsequent node in the list.
Linked List
When a set of items is organized sequentially, it is termed as list. Linked list is a list whose order is given by links from one item to the next. It contains a link to the structure containing the next item so we can say that it is a completely different way to represent a list. In linked list, each structure of the list is known as node and it consists of two fields (one for containing the item and other one is for containing the next item address).
15.14 LAB: Binary search
Binary search can be implemented as a recursive
Complete the recursive function BinarySearch() with the following specifications:
- Parameters:
- a target integer
- a
vector of integers - lower and upper bounds within which the recursive call will search
- Return value:
- the index within the vector where the target is located
- -1 if target is not found
The template provides the main
The algorithm begins by choosing an index midway between the lower and upper bounds.
- If target == integers.at(index) return index
- If lower == upper, return -1 to indicate not found
- Otherwise call the function recursively on half the vector parameter:
- If integers.at(index) < target, search the vector from index + 1 to upper
- If integers.at(index) > target, search the vector from lower to index - 1
The vector must be ordered, but duplicates are allowed.
Once the search algorithm works correctly, add the following to BinarySearch():
- Count the number of calls to BinarySearch().
- Count the number of times when the target is compared to an element of the vector. Note: lower == upper should not be counted.
Hint: Use a global variable to count calls and comparisons.
The input of the program consists of:
- the number of integers in the vector
- the integers in the vector
- the target to be located
Ex: If the input is:
9 1 2 3 4 5 6 7 8 9 2the output is:
index: 1, recursions: 2, comparisons: 3ATTENTION
WHERE IT SAYS CODE HERE, IS WHERE I MUST ADD CODE. EVERYTHING ELSE CANNOT BE ALTERED
#include <iostream>
#include <vector>
#include <algorithm>
using namespace std;
// Read integers from input and store them in a vector.
// Return the vector.
vector<int> ReadIntegers() {
int size;
cin >> size;
vector<int> integers(size);
for (int i = 0; i < size; ++i) { // Read the numbers
cin >> integers.at(i);
}
sort(integers.begin(), integers.end());
return integers;
}
int BinarySearch(int target, vector<int> integers, int lower, int upper) {
/* code here. */
}
int main() {
int target;
int index;
vector<int> integers = ReadIntegers();
cin >> target;
index = BinarySearch(target, integers, 0, integers.size() - 1);
printf("index: %d, recursions: %d, comparisons: %d\n",
index, recursions, comparisons);
return 0;
}

Trending now
This is a popular solution!
Step by step
Solved in 2 steps with 2 images

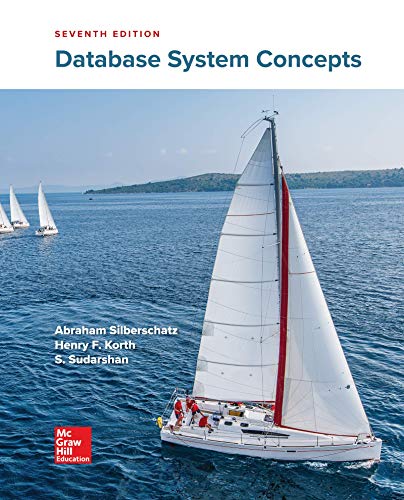
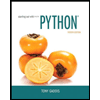
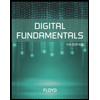
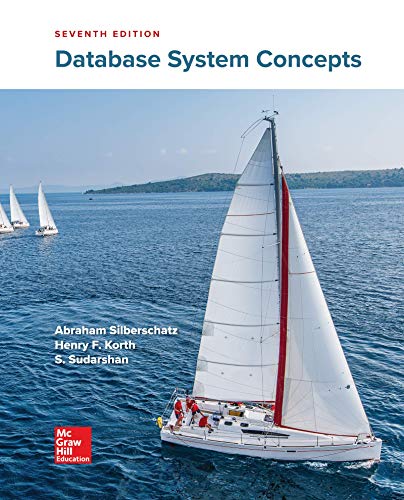
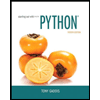
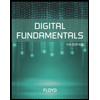
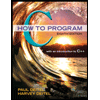
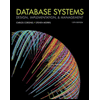
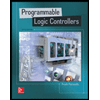