11.15 LAB: Instrument information (derived classes) Given main() and the Instrument class, define a derived class, stringInstrument, with methods to set and get private fields of the following types: • int to store the number of strings • int to store the number of frets • boolean to store whether the instrument is bowed Ex. If the input is: Drums Zildjian 2015 2500 Guitar Gibson 2002 1200 6 19 false the output is: Instrument Information: Name: Drums Manufacturer: Zildjian Year built: 2015 Cost: 2500 Instrument Information: Name: Guitar Manufacturer: Gibson. Year built: 2002 Cost: 1200 Number of strings: 6 Number of frets: 19 Is bowed: false
OOPs
In today's technology-driven world, computer programming skills are in high demand. The object-oriented programming (OOP) approach is very much useful while designing and maintaining software programs. Object-oriented programming (OOP) is a basic programming paradigm that almost every developer has used at some stage in their career.
Constructor
The easiest way to think of a constructor in object-oriented programming (OOP) languages is:
I need help with creating a Java program described in the image below:
StringInstrument.java:
// TODO: Define a class: StringInstrument that is derived from the Instrument class
public class StringInstrument extends Instrument {
// TODO: Declare private fields
// TODO: Define mutator methods -
// setNumOfStrings(), setNumOfFrets(), setIsBowed()
// TODO: Define accessor methods -
// getNumOfStrings(), getNumOfFrets(), getIsBowed()
}
Instrument.java:
public class Instrument {
protected String instrumentName;
protected String instrumentManufacturer;
protected int yearBuilt, cost;
public void setName(String userName) {
instrumentName = userName;
}
public String getName() {
return instrumentName;
}
public void setManufacturer(String userManufacturer) {
instrumentManufacturer = userManufacturer;
}
public String getManufacturer(){
return instrumentManufacturer;
}
public void setYearBuilt(int userYearBuilt) {
yearBuilt = userYearBuilt;
}
public int getYearBuilt() {
return yearBuilt;
}
public void setCost(int userCost) {
cost = userCost;
}
public int getCost() {
return cost;
}
public void printInfo() {
System.out.println("Instrument Information: ");
System.out.println(" Name: " + instrumentName);
System.out.println(" Manufacturer: " + instrumentManufacturer);
System.out.println(" Year built: " + yearBuilt);
System.out.println(" Cost: " + cost);
}
}
Instrument.Information.java:
import java.util.Scanner;
public class InstrumentInformation {
public static void main(String[] args) {
Scanner scnr = new Scanner(System.in);
Instrument myInstrument = new Instrument();
StringInstrument myStringInstrument = new StringInstrument();
String instrumentName, manufacturerName, stringInstrumentName, stringManufacturer;
int yearBuilt, cost, stringYearBuilt, stringCost, numStrings, numFrets;
boolean bowed;
instrumentName = scnr.nextLine();
manufacturerName = scnr.nextLine();
yearBuilt = scnr.nextInt();
scnr.nextLine();
cost = scnr.nextInt();
scnr.nextLine();
stringInstrumentName = scnr.nextLine();
stringManufacturer = scnr.nextLine();
stringYearBuilt = scnr.nextInt();
stringCost = scnr.nextInt();
numStrings = scnr.nextInt();
numFrets = scnr.nextInt();
bowed = scnr.nextBoolean();
myInstrument.setName(instrumentName);
myInstrument.setManufacturer(manufacturerName);
myInstrument.setYearBuilt(yearBuilt);
myInstrument.setCost(cost);
myInstrument.printInfo();
myStringInstrument.setName(stringInstrumentName);
myStringInstrument.setManufacturer(stringManufacturer);
myStringInstrument.setYearBuilt(stringYearBuilt);
myStringInstrument.setCost(stringCost);
myStringInstrument.setNumOfStrings(numStrings);
myStringInstrument.setNumOfFrets(numFrets);
myStringInstrument.setIsBowed(bowed);
myStringInstrument.printInfo();
System.out.println(" Number of strings: " + myStringInstrument.getNumOfStrings());
System.out.println(" Number of frets: " + myStringInstrument.getNumOfFrets());
System.out.println(" Is bowed: " + myStringInstrument.getIsBowed());
}
}


Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 6 images

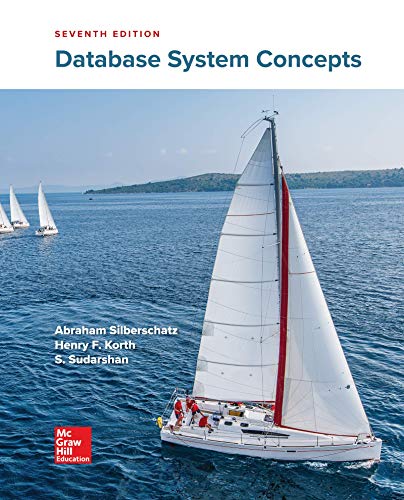
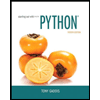
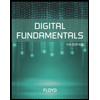
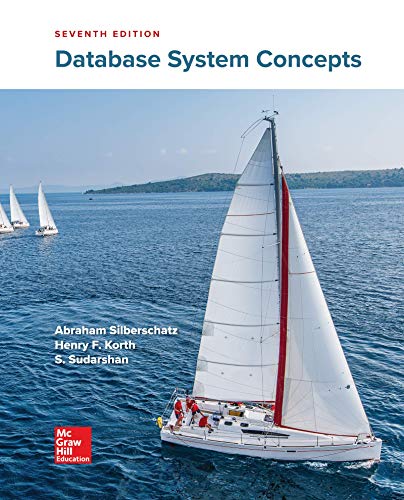
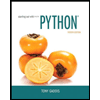
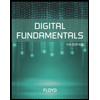
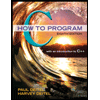
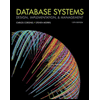
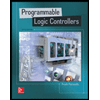