10.10 LAB: Quadratic formula Implement the quadratic_formula() function. The function takes 3 arguments, a, b, and c, and computes the two results of the quadratic formula: x1 = 2-3-77 -b+√b² - 4ac -b-√b² - 4ac 2a The quadratic_formula() function returns the tuple (x1, x2). Ex: When a = 1, b = -5, and c = 6, quadratic_formula() returns (3, 2). Code provided in main.py reads a single input line containing values for a, b, and c, separated by spaces. Each input is converted to a float and passed to the quadratic_formula() function. Ex: If the input is: x2 = 2a
10.10 LAB: Quadratic formula Implement the quadratic_formula() function. The function takes 3 arguments, a, b, and c, and computes the two results of the quadratic formula: x1 = 2-3-77 -b+√b² - 4ac -b-√b² - 4ac 2a The quadratic_formula() function returns the tuple (x1, x2). Ex: When a = 1, b = -5, and c = 6, quadratic_formula() returns (3, 2). Code provided in main.py reads a single input line containing values for a, b, and c, separated by spaces. Each input is converted to a float and passed to the quadratic_formula() function. Ex: If the input is: x2 = 2a
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
![1 # TODO: Import math module
2
3 def quadratic_formula(a, b, c):
4
# TODO: Compute the quadratic formula results in variables x1 and x2
return (x1, x2)
5
6
7
8 def print_number(number, prefix_str):
9
10
11
12
13
14
15 if
16
17
18
if float(int (number))
number:
print(f' {prefix_str}{number:.0f}')
print(f' {prefix_str}{number:.2f}')
else:
__name_____
input_line
split_line
11
'__main__":
input()
=
=
==
input_line.split(" ")
a = float(split_line [0])
b = float(split_line[1])
C = float(split_line[2])
19
20
21
solution = quadratic_formula(a, b, c)
22 print(f'Solutions to {a:.0f}x^2 + {b:.0f}x + {c:.0f} = 0')
23
24
print_number(solution[0], 'x1
print_number(solution[1],
'x2
=
=
')
'D](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F9978ffa8-8e6a-4550-8363-e044b6a6e895%2Fdd360eff-fe97-4b45-a548-cec454b2b516%2Faj0yt9_processed.png&w=3840&q=75)
Transcribed Image Text:1 # TODO: Import math module
2
3 def quadratic_formula(a, b, c):
4
# TODO: Compute the quadratic formula results in variables x1 and x2
return (x1, x2)
5
6
7
8 def print_number(number, prefix_str):
9
10
11
12
13
14
15 if
16
17
18
if float(int (number))
number:
print(f' {prefix_str}{number:.0f}')
print(f' {prefix_str}{number:.2f}')
else:
__name_____
input_line
split_line
11
'__main__":
input()
=
=
==
input_line.split(" ")
a = float(split_line [0])
b = float(split_line[1])
C = float(split_line[2])
19
20
21
solution = quadratic_formula(a, b, c)
22 print(f'Solutions to {a:.0f}x^2 + {b:.0f}x + {c:.0f} = 0')
23
24
print_number(solution[0], 'x1
print_number(solution[1],
'x2
=
=
')
'D

Transcribed Image Text:10.10 LAB: Quadratic formula
Implement the quadratic_formula() function. The function takes 3 arguments, a, b, and c, and computes the two results of the quadratic
formula:
2 -3-77
the output is:
Solutions to 2x^2 + −3x + −77 = 0
x1 = 7
x2
=
The quadratic_formula() function returns the tuple (x1, x2). Ex: When a 1, b = -5, and c = 6, quadratic_formula() returns (3, 2).
Code provided in main.py reads a single input line containing values for a, b, and c, separated by spaces. Each input is converted to a float
and passed to the quadratic_formula() function.
Ex: If the input is:
-5.50
x1 =
487180.3542414.qx3zqy7
x2 =
−6+ √b²
2a
4ac
-b- √b² - 4ac
2a
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 4 steps with 2 images

Similar questions
Recommended textbooks for you
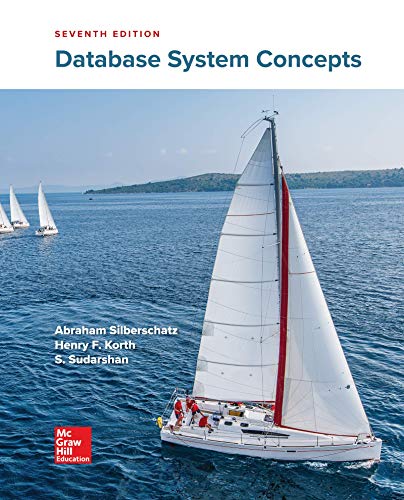
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
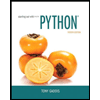
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
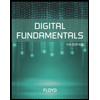
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
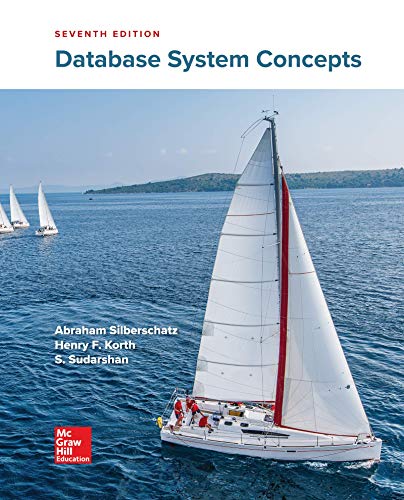
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
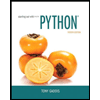
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
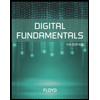
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
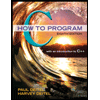
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
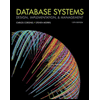
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
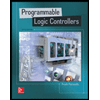
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education