1. xp_levels should be dictionary. If it is not, raise an TypeError exception with a message "Survey result is represented in a wrong way!" 2. Check all landscapes in xp_levels are strings. a. If there are multiple invalid landscape representations, you only need to throw an TypeError exception for the first invalid landscape encountered, along with a message "Landscape is represented in a wrong way!" 3. Check all experience levels (dictionary values) are integers, each a positive value that is a multiple of 10 (e.g. 10, 20, 30, 40). a. If there are multiple invalid experience levels (of a type other than int), you only need to throw a TypeError exception for the first invalid experience level encountered, along with a message "Experience level for is represented in a wrong way!" b. If there are multiple experience level values that are not a multiple of 10, you only need to throw an ValueError exception for the first such experience level encountered, along with a message "Experience level for is incorrectly entered!" 4. Check all landscapes are in landscapes (a list provided in the starter file). a. If there are multiple landscapes in the dictionary but not in the selected list of landscapes, you only need to throw an KeyError exception for the first non-matching landscape encountered, along with a message "Landscape does not exist in Pythonia!" 5. Check you have visited at least 6 out of num_landscapes of the selected landscapes - security guards might reject you if you miss too much experience in Pythonia. a. If more than six landscapes are missing, raise an KeyError exception with a message "You miss too many Pythonia landscapes!" Upon passing all checks, the security guards will evaluate your ability to survive in Javania. Your function will need to check the following conditions and return True if they pass, and False otherwise. You have visited at least 9 landscapes in total. - The average experience level across all landscapes you have visited is greater than or equal to 20. The following data structures are provided: - landscapes: a list of strings representing a subset of landscapes you have visited. num_landscapes: integer representing the total number of landscapes in landscapes. File "/autograder/source/lab09.py", line 24, in lab09.admission_checker Failed example: admission_checker(xp_levels) Expected: Traceback (most recent call last): KeyError: 'Landscape Sorting does not exist in Pythonia!' Got: 'Landscape Sorting does not exist in Pythonia!'
landscapes = ['Return vs Print', 'Dictionaries', 'Files', 'List Comprehension',
'Lambda Functions', 'Iterators', 'Complexity',
'Scope of variables', 'Classes and Objects', 'Inheritance',
'Special Methods','Mutable data']
num_landscapes = 12
# Question 1
def admission_checker(xp_levels):
"""
Checks necessary conditions for admission into Javania. Raise an exception
when condition check fails. If all necessary conditions pass, evaluate
capability to survive in Javania -- return True if it passes
and False otherwise.
>>> xp_levels = {'Return vs Print': 20, 'Dictionaries': 20, 'Files': 20,\
'List Comprehension': 20, 'Lambda Functions': 20, 'Iterators': 20,\
'Complexity': 20, 'Scope of variables': 20, 'Classes and Objects': 20,\
'Inheritance': 20, 'Special Methods': 20, 'Sorting': 20}
>>> admission_checker(xp_levels)
Traceback (most recent call last):
...
KeyError: 'Landscape Sorting does not exist in Pythonia!'
>>> xp_levels = {'Return vs Print': 20, 'Dictionaries': 20, 'Files': 20,\
'List Comprehension': 20, 'Lambda Functions': 20, 'Iterators': 20,\
'Complexity': 20, 'Scope of variables': 20, 'Classes and Objects': 20,\
'Inheritance': 20, 'Special Methods': 20, ('Mutable data',): 20}
>>> admission_checker(xp_levels)
Traceback (most recent call last):
...
TypeError: Landscape is represented in a wrong way!
>>> xp_levels = {'Return vs Print': 20, 'Dictionaries': 20, 'Files': 20,\
'List Comprehension': 10, 'Lambda Functions': 10, 'Iterators': 10,\
'Complexity': 10, 'Scope of variables': 10, 'Classes and Objects': 10,\
'Inheritance': 10, 'Special Methods': 10, 'Mutable data': 10}
>>> admission_checker(xp_levels)
False
>>> xp_levels = {'Return vs Print': 20, 'Dictionaries': 20, 'Files': 20,\
'List Comprehension': 20, 'Lambda Functions': 20, 'Iterators': 20,\
'Complexity': 20, 'Scope of variables': 20, 'Classes and Objects': 20,\
'Inheritance': 20, 'Special Methods': 20, 'Mutable data': 20}
>>> admission_checker(xp_levels)
True
"""
# YOUR CODE GOES HERE #
try:
if not isinstance(xp_levels,dict):
raise TypeError('Survey result is represented in a wrong way!')
invalid_landscapes = []
for key, value in xp_levels.items():
if not isinstance(key, str):
invalid_landscapes.append(key)
if invalid_landscapes:
raise TypeError("Landscape for is represented in a wrong way!")
invalid_type = []
invalid_experience = []
for key, value in xp_levels.items():
if not isinstance(value, int):
invalid_type.append(key)
if value <= 0 or value % 10 != 0:
invalid_experience.append(key)
if invalid_type:
raise TypeError("Experience level for " + str(invalid_type[0]) + " is represented in a wrong way!")
if invalid_experience:
raise ValueError("Experience level for " + str(invalid_experience[0]) + " is incorrectly entered!")
invalid_land = []
for key,value in xp_levels.items():
if key not in landscapes:
invalid_land.append(key)
if invalid_land:
raise KeyError('Landscape ' + str(invalid_land[0]) + ' does not exist in Pythonia!')
visited_landscapes = [landscape for landscape in xp_levels if landscape in landscapes]
if len(visited_landscapes) < 6:
raise KeyError('You miss too many Pythonia landscapes!')
if len(visited_landscapes) >= 9:
total_xp = sum(xp_levels[landscape] for landscape in visited_landscapes)
average_xp = total_xp / len(visited_landscapes)
if average_xp >= 20:
return True
return False
except (TypeError, ValueError, KeyError) as error:
print(error)
xp_levels = {'Return vs Print': 20, 'Dictionaries': 20, 'Files': 20,\
'List Comprehension': 20, 'Lambda Functions': 20, 'Iterators': 20,\
'Complexity': 20, 'Scope of variables': 20, 'Classes and Objects': 20,\
'Inheritance': 20, 'Special Methods': 20, 'Sorting': 20}
admission_checker(xp_levels)
DONT USE ASSERTION USE TRY/EXCEPT



Step by step
Solved in 2 steps

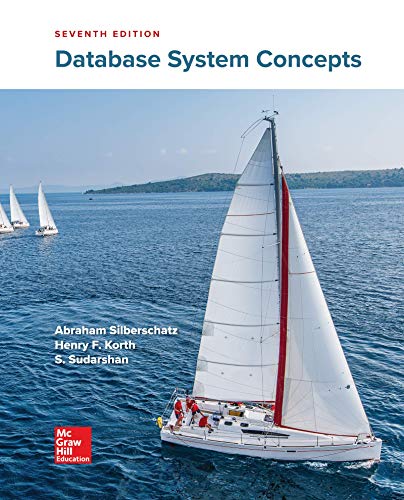
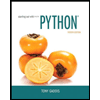
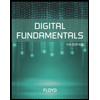
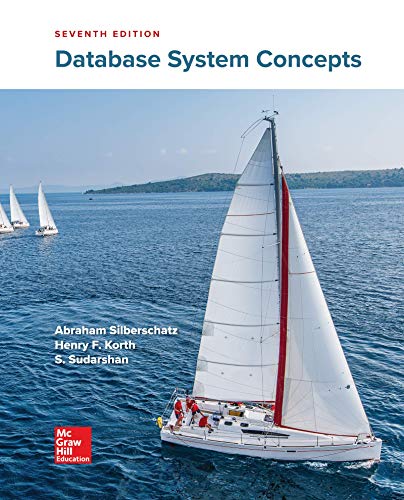
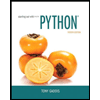
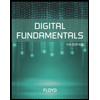
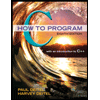
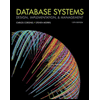
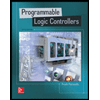