# 1. Write a new function to multiply two numbers together and return the result 2. Write a new function to divide tuo numbers and return the result. Run the progran and try entering 0 as the second number, what happens? How could you fix this? # 3. Write a nen function to display a menu which should allow the user to change the numbers or choose which operation they would like to perform, e.g. choose from addition, multiplication, etc E4. Modify your menu function to include an option to exit the program and add a loop to allow the user to restart the progran until they choose the exit option E 5. Write a neu function which takes two arguments, a number and a power and returns the numberApower. You should research and use the math.pow() function to do this. What is the difference between math.pow() and **? E 6. Write a new function which takes two arguments, a number and the nth root (e.9. 2 WOuld be square root, 3 cubic root, etc) and returns the nth root of the number. You should research an appropriate way to do this. # 7. Modify your menu function to include the two new functions from tasks 5 and 6 # 8. Add comments to the code to explain what it is doing ----- Subroutines -----..... def my_add(x y): return x + Y def my subtract(x,y): return x - Y # --------- - Main progran - *********-- # get initial numbers x and y first_num = int(input("x ")) second_num- int(input("y ")) # print result of addition print("x + y =", my_add(first_num, second_num)) # print result of subtraction print("x - y =", my_subtract(first_nun, second_num))
# 1. Write a new function to multiply two numbers together and return the result 2. Write a new function to divide tuo numbers and return the result. Run the progran and try entering 0 as the second number, what happens? How could you fix this? # 3. Write a nen function to display a menu which should allow the user to change the numbers or choose which operation they would like to perform, e.g. choose from addition, multiplication, etc E4. Modify your menu function to include an option to exit the program and add a loop to allow the user to restart the progran until they choose the exit option E 5. Write a neu function which takes two arguments, a number and a power and returns the numberApower. You should research and use the math.pow() function to do this. What is the difference between math.pow() and **? E 6. Write a new function which takes two arguments, a number and the nth root (e.9. 2 WOuld be square root, 3 cubic root, etc) and returns the nth root of the number. You should research an appropriate way to do this. # 7. Modify your menu function to include the two new functions from tasks 5 and 6 # 8. Add comments to the code to explain what it is doing ----- Subroutines -----..... def my_add(x y): return x + Y def my subtract(x,y): return x - Y # --------- - Main progran - *********-- # get initial numbers x and y first_num = int(input("x ")) second_num- int(input("y ")) # print result of addition print("x + y =", my_add(first_num, second_num)) # print result of subtraction print("x - y =", my_subtract(first_nun, second_num))
Computer Networking: A Top-Down Approach (7th Edition)
7th Edition
ISBN:9780133594140
Author:James Kurose, Keith Ross
Publisher:James Kurose, Keith Ross
Chapter1: Computer Networks And The Internet
Section: Chapter Questions
Problem R1RQ: What is the difference between a host and an end system? List several different types of end...
Related questions
Question
first 3 questions of tasks

Transcribed Image Text:# TASKS
# 1. Write a new function to multiply two numbers together and return the result
# 2. Write a new function to divide two numbers and return the result. Run the program and try entering '0' as the second number, what happens? How could you fix this?
# 3. Write a new function to display a menu which should allow the user to change the numbers or choose which operation they would like to perform, e.g. choose from addition, multiplication, etc
# 4. Modify your menu function to include an option to exit the program and add a loop to allow the user to restart the program until they choose the exit option
# 5. Write a new function which takes two arguments, a number and a power and returns the number^power. You should research and use the math.pow() function to do this. What is the difference between math.pow() and **?
# 6. Write a new function which takes two arguments, a number and the nth root (e.g. 2 would be square root, 3 cubic root, etc) and returns the nth root of the number. You should research an appropriate way to do this.
# 7. Modify your menu function to include the two new functions from tasks 5 and 6
# 8. Add comments to the code to explain what it is doing
Subroutines
adef my_add(x,y):
return x + y
def my subtract(x y):
return x -y
Main program
# get initial numbers x and y
first_num = int(input("x= "))
second_num = int(input("y= "))
# print result of addition
print("x + y =", my_add(first_num,second_num))
# print result of subtraction
print("x
y =", my_subtract(first_num,second_num))
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
Step by step
Solved in 2 steps

Recommended textbooks for you
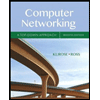
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
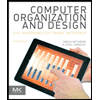
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
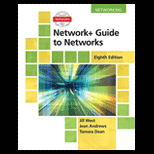
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
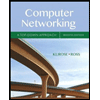
Computer Networking: A Top-Down Approach (7th Edi…
Computer Engineering
ISBN:
9780133594140
Author:
James Kurose, Keith Ross
Publisher:
PEARSON
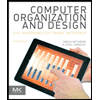
Computer Organization and Design MIPS Edition, Fi…
Computer Engineering
ISBN:
9780124077263
Author:
David A. Patterson, John L. Hennessy
Publisher:
Elsevier Science
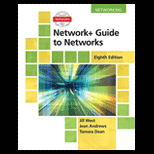
Network+ Guide to Networks (MindTap Course List)
Computer Engineering
ISBN:
9781337569330
Author:
Jill West, Tamara Dean, Jean Andrews
Publisher:
Cengage Learning
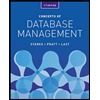
Concepts of Database Management
Computer Engineering
ISBN:
9781337093422
Author:
Joy L. Starks, Philip J. Pratt, Mary Z. Last
Publisher:
Cengage Learning
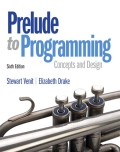
Prelude to Programming
Computer Engineering
ISBN:
9780133750423
Author:
VENIT, Stewart
Publisher:
Pearson Education
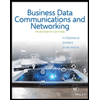
Sc Business Data Communications and Networking, T…
Computer Engineering
ISBN:
9781119368830
Author:
FITZGERALD
Publisher:
WILEY