1. How to modify below Java OpenGL to add animation that causes the drawn point to grow and shrink, in a cycle. Hint: use the glPointSize() function, with a variable as the parameter. 2. How to modify below Java OpenGL the result from no 1, so that the drawn rectangle (not point) will grow and shrink in a cycle. Possible try to move the rectangle in x-axis. import javax.swing.*; import static com.jogamp.opengl.GL4.*; import com.jogamp.opengl.*; import com.jogamp.opengl.awt.GLCanvas; public class Program2_2 extends JFrame implements GLEventListener { private GLCanvas myCanvas; private int renderingProgram; private int vao[] = new int[1]; public Program2_2() { setTitle("Chapter2 - Program2"); setSize(600, 400); setLocation(200, 200); myCanvas = new GLCanvas(); myCanvas.addGLEventListener(this); this.add(myCanvas); this.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); this.setVisible(true); } public void display(GLAutoDrawable drawable) { GL4 gl = (GL4) GLContext.getCurrentGL(); gl.glUseProgram(renderingProgram); gl.glPointSize(30.0f); gl.glDrawArrays(GL_POINTS, 0, 1); } public void init(GLAutoDrawable drawable) { GL4 gl = (GL4) GLContext.getCurrentGL(); renderingProgram = createShaderProgram(); gl.glBindVertexArray(vao[0]); } private int createShaderProgram() { GL4 gl = (GL4) GLContext.getCurrentGL(); String vshaderSource[] = {"#version 430 \n", "void main(void) \n", "{ gl_Position = vec4(0.0, 0.0, 0.0, 1.0); } \n", }; /*String fshaderSource[] = {"#version 430 \n", "out vec4 color; \n", "void main(void) \n", "{ color = vec4(0.0, 0.0, 1.0, 1.0); } \n", };*/ String fshaderSource[] = {"#version 430 \n", "out vec4 color; \n", "void main(void) \n", "{if (gl_FragCoord.x < 295) color = vec4(1.0, 0.0, 0.0, 1.0); \n", "else color = vec4(0.0, 0.0, 1.0, 1.0);} \n", }; int vShader = gl.glCreateShader(GL_VERTEX_SHADER); gl.glShaderSource(vShader, 3, vshaderSource, null, 0); // 3 is the count of lines of source code gl.glCompileShader(vShader); int fShader = gl.glCreateShader(GL_FRAGMENT_SHADER); gl.glShaderSource(fShader, 5, fshaderSource, null, 0); // 4 is the count of lines of source code gl.glCompileShader(fShader); int vfProgram = gl.glCreateProgram(); gl.glAttachShader(vfProgram, vShader); gl.glAttachShader(vfProgram, fShader); gl.glLinkProgram(vfProgram); gl.glDeleteShader(vShader); gl.glDeleteShader(fShader); return vfProgram; } public void reshape(GLAutoDrawable drawable, int x, int y, int width, int height) { } public void dispose(GLAutoDrawable drawable) { } } vertShader.glsl #version 430 uniform float offset; void main(void) { if (gl_VertexID == 0) gl_Position = vec4( 0.25 + offset, -0.25, 0.0, 1.0); else if (gl_VertexID == 1) gl_Position = vec4(-0.25 + offset, -0.25, 0.0, 1.0); else gl_Position = vec4( 0.25 + offset, 0.25, 0.0, 1.0); } fragShader.glsl #version 430 out vec4 color; void main(void) { if (gl_FragCoord.x < 295) color = vec4(1.0, 0.0, 0.0, 1.0); else if(gl_FragCoord.x > 295 && gl_FragCoord.x < 310) color = vec4(1.0, 0.0, 1.0, 1.0); else color = vec4(0.0, 0.0, 1.0, 1.0); }
1. How to modify below Java OpenGL to add animation that causes the drawn point to grow and shrink, in a cycle. Hint: use the glPointSize() function, with a variable as the parameter.
2. How to modify below Java OpenGL the result from no 1, so that the drawn rectangle (not point) will grow and shrink in a cycle. Possible try to move the rectangle in x-axis.
import javax.swing.*;
import static com.jogamp.opengl.GL4.*;
import com.jogamp.opengl.*;
import com.jogamp.opengl.awt.GLCanvas;
public class Program2_2 extends JFrame implements GLEventListener {
private GLCanvas myCanvas;
private int renderingProgram;
private int vao[] = new int[1];
public Program2_2() {
setTitle("Chapter2 - Program2");
setSize(600, 400);
setLocation(200, 200);
myCanvas = new GLCanvas();
myCanvas.addGLEventListener(this);
this.add(myCanvas);
this.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
this.setVisible(true);
}
public void display(GLAutoDrawable drawable) {
GL4 gl = (GL4) GLContext.getCurrentGL();
gl.glUseProgram(renderingProgram);
gl.glPointSize(30.0f);
gl.glDrawArrays(GL_POINTS, 0, 1);
}
public void init(GLAutoDrawable drawable) {
GL4 gl = (GL4) GLContext.getCurrentGL();
renderingProgram = createShaderProgram();
gl.glBindVertexArray(vao[0]);
}
private int createShaderProgram() {
GL4 gl = (GL4) GLContext.getCurrentGL();
String vshaderSource[] =
{"#version 430 \n",
"void main(void) \n",
"{ gl_Position = vec4(0.0, 0.0, 0.0, 1.0); } \n",
};
/*String fshaderSource[] =
{"#version 430 \n",
"out vec4 color; \n",
"void main(void) \n",
"{ color = vec4(0.0, 0.0, 1.0, 1.0); } \n",
};*/
String fshaderSource[] =
{"#version 430 \n",
"out vec4 color; \n",
"void main(void) \n",
"{if (gl_FragCoord.x < 295) color = vec4(1.0, 0.0, 0.0, 1.0); \n",
"else color = vec4(0.0, 0.0, 1.0, 1.0);} \n",
};
int vShader = gl.glCreateShader(GL_VERTEX_SHADER);
gl.glShaderSource(vShader, 3, vshaderSource, null, 0); // 3 is the count of lines of source code
gl.glCompileShader(vShader);
int fShader = gl.glCreateShader(GL_FRAGMENT_SHADER);
gl.glShaderSource(fShader, 5, fshaderSource, null, 0); // 4 is the count of lines of source code
gl.glCompileShader(fShader);
int vfProgram = gl.glCreateProgram();
gl.glAttachShader(vfProgram, vShader);
gl.glAttachShader(vfProgram, fShader);
gl.glLinkProgram(vfProgram);
gl.glDeleteShader(vShader);
gl.glDeleteShader(fShader);
return vfProgram;
}
public void reshape(GLAutoDrawable drawable, int x, int y, int width, int height) {
}
public void dispose(GLAutoDrawable drawable) {
}
}
vertShader.glsl
#version 430
uniform float offset;
void main(void)
{
if (gl_VertexID == 0) gl_Position = vec4( 0.25 + offset, -0.25, 0.0, 1.0);
else if (gl_VertexID == 1) gl_Position = vec4(-0.25 + offset, -0.25, 0.0, 1.0);
else gl_Position = vec4( 0.25 + offset, 0.25, 0.0, 1.0);
}
fragShader.glsl
#version 430
out vec4 color;
void main(void)
{
if (gl_FragCoord.x < 295) color = vec4(1.0, 0.0, 0.0, 1.0);
else if(gl_FragCoord.x > 295 && gl_FragCoord.x < 310) color = vec4(1.0, 0.0, 1.0, 1.0);
else color = vec4(0.0, 0.0, 1.0, 1.0);
}

Step by step
Solved in 2 steps

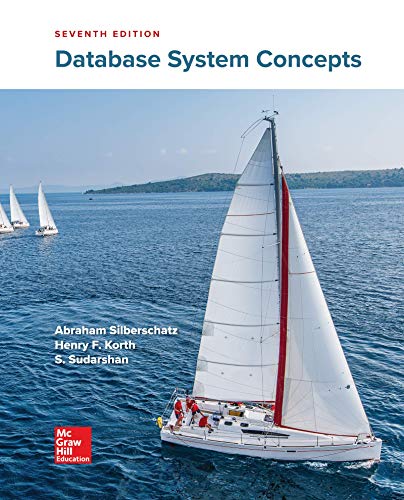
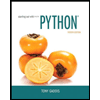
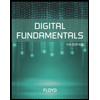
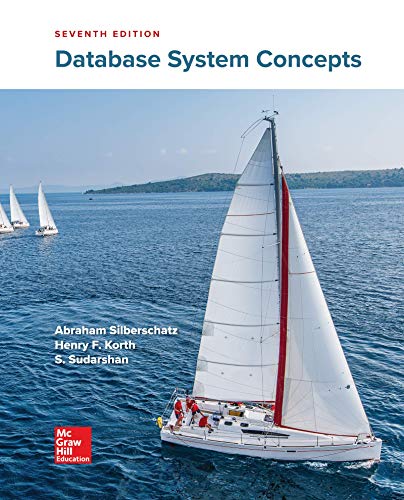
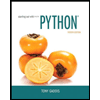
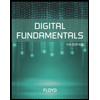
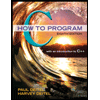
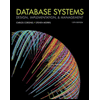
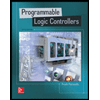