1 import random 2 3 def heads_or_tails(): # Type your code here. 4 7 if name = random.seed(1) number of flips int(input()) '_main: 8 10 11 # Type your code here.
1 import random 2 3 def heads_or_tails(): # Type your code here. 4 7 if name = random.seed(1) number of flips int(input()) '_main: 8 10 11 # Type your code here.
Database System Concepts
7th Edition
ISBN:9780078022159
Author:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Chapter1: Introduction
Section: Chapter Questions
Problem 1PE
Related questions
Question
I need help solving this in python with given template
![```python
import random
def heads_or_tails():
# Type your code here.
if __name__ == '__main__':
random.seed(1)
number_of_flips = int(input())
# Type your code here.
```
### Explanation
This code snippet is set up for a Python script that intends to simulate a coin flip. Here's a breakdown of the components:
1. **Import Statement**: `import random`
- This line imports the `random` module, which supports generating random numbers and is essential for simulating randomness, such as flipping a coin.
2. **Function Definition**: `def heads_or_tails():`
- A function named `heads_or_tails` is defined. There is a placeholder comment inside where the actual coin flipping logic needs to be implemented.
3. **Main Execution Block**:
- The line `if __name__ == '__main__':` checks if the script is being run directly (not imported as a module).
- `random.seed(1)` initializes the random number generator with a seed of 1, which ensures that the results are reproducible for testing purposes.
- `number_of_flips = int(input())` prompts the user for input, expecting an integer that represents the number of times the coin should be flipped.
4. **Code Placeholder**:
- The comments `# Type your code here.` indicate places where additional code should be written to complete the program functionality. Specifically, the function should simulate flipping a coin and determine whether it lands on heads or tails for the number of times specified by the user's input.
### Usage
To complete the program, one would typically add random selection logic inside the `heads_or_tails` function, using `random.choice(['heads', 'tails'])` to randomly decide the result of each flip. The accumulated results could then be displayed or used for further analysis.](/v2/_next/image?url=https%3A%2F%2Fcontent.bartleby.com%2Fqna-images%2Fquestion%2F722c0934-aff7-4600-989e-1d198e6697ab%2F70fe6fc8-49c8-4bc3-9a3e-bb83ea931345%2Ff5ufsn_processed.png&w=3840&q=75)
Transcribed Image Text:```python
import random
def heads_or_tails():
# Type your code here.
if __name__ == '__main__':
random.seed(1)
number_of_flips = int(input())
# Type your code here.
```
### Explanation
This code snippet is set up for a Python script that intends to simulate a coin flip. Here's a breakdown of the components:
1. **Import Statement**: `import random`
- This line imports the `random` module, which supports generating random numbers and is essential for simulating randomness, such as flipping a coin.
2. **Function Definition**: `def heads_or_tails():`
- A function named `heads_or_tails` is defined. There is a placeholder comment inside where the actual coin flipping logic needs to be implemented.
3. **Main Execution Block**:
- The line `if __name__ == '__main__':` checks if the script is being run directly (not imported as a module).
- `random.seed(1)` initializes the random number generator with a seed of 1, which ensures that the results are reproducible for testing purposes.
- `number_of_flips = int(input())` prompts the user for input, expecting an integer that represents the number of times the coin should be flipped.
4. **Code Placeholder**:
- The comments `# Type your code here.` indicate places where additional code should be written to complete the program functionality. Specifically, the function should simulate flipping a coin and determine whether it lands on heads or tails for the number of times specified by the user's input.
### Usage
To complete the program, one would typically add random selection logic inside the `heads_or_tails` function, using `random.choice(['heads', 'tails'])` to randomly decide the result of each flip. The accumulated results could then be displayed or used for further analysis.

Transcribed Image Text:**Title: Simulating Coin Flips for Decision Making**
**Description:**
This educational section guides you through writing a program that simulates flipping a coin to make decisions. The number of decisions needed serves as the input, while the output will display either "heads" or "tails." Ensure the input is a number greater than 0.
**Example:**
- **Input:**
- 3
- **Output:**
```
heads
heads
tails
```
**Detailed Instructions:**
- **Reproducibility for Auto-Grading:**
Seed the program using a value of 1 to achieve consistent outputs. In practical scenarios, seeding with the current time generates diverse outputs, although this method is not suitable for auto-grading purposes.
- **Common Mistake to Avoid:**
- Do not seed before each call to `random.randint()`. Seed only once at the beginning of your program. Then, you can call `random.randint()` multiple times without reseeding.
- **Function Definition:**
- Create a function `heads_or_tails()` that randomly selects 0 or 1. This function should return "heads" when the value is 0 and "tails" when the value is 1. Remember, 0 corresponds to "heads" and 1 to "tails."
By following these guidelines, you can efficiently simulate coin tosses for decision-making in your program.
Expert Solution

This question has been solved!
Explore an expertly crafted, step-by-step solution for a thorough understanding of key concepts.
This is a popular solution!
Trending now
This is a popular solution!
Step by step
Solved in 3 steps with 1 images

Knowledge Booster
Learn more about
Need a deep-dive on the concept behind this application? Look no further. Learn more about this topic, computer-science and related others by exploring similar questions and additional content below.Recommended textbooks for you
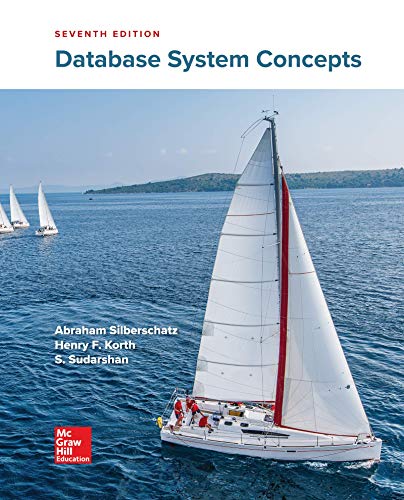
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
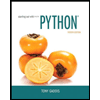
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
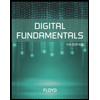
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
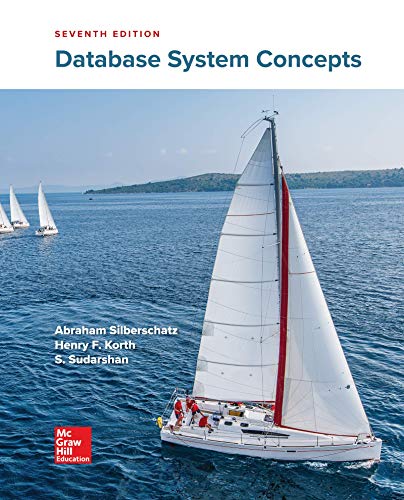
Database System Concepts
Computer Science
ISBN:
9780078022159
Author:
Abraham Silberschatz Professor, Henry F. Korth, S. Sudarshan
Publisher:
McGraw-Hill Education
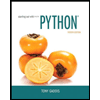
Starting Out with Python (4th Edition)
Computer Science
ISBN:
9780134444321
Author:
Tony Gaddis
Publisher:
PEARSON
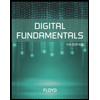
Digital Fundamentals (11th Edition)
Computer Science
ISBN:
9780132737968
Author:
Thomas L. Floyd
Publisher:
PEARSON
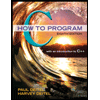
C How to Program (8th Edition)
Computer Science
ISBN:
9780133976892
Author:
Paul J. Deitel, Harvey Deitel
Publisher:
PEARSON
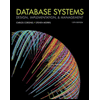
Database Systems: Design, Implementation, & Manag…
Computer Science
ISBN:
9781337627900
Author:
Carlos Coronel, Steven Morris
Publisher:
Cengage Learning
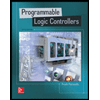
Programmable Logic Controllers
Computer Science
ISBN:
9780073373843
Author:
Frank D. Petruzella
Publisher:
McGraw-Hill Education