ps3_answers
py
keyboard_arrow_up
School
Georgia Institute Of Technology *
*We aren’t endorsed by this school
Course
7638
Subject
Mechanical Engineering
Date
Dec 6, 2023
Type
py
Pages
9
Uploaded by justinfricks
######################################################################
# This file copyright the Georgia Institute of Technology
#
# Permission is given to students to use or modify this file (only)
# to work on their assignments.
#
# You may NOT publish this file or make it available to others not in
# the course.
#
######################################################################
######################################################################
# How to use:
#
# For fill-in questions, change the value of each answer variable.
#
# For programming questions, change the implementation of the
# function provided.
#
# Run the file from the command line to check:
#
# python ps3_answers.py
#
######################################################################
from __future__ import division
from builtins import str
from builtins import range
from builtins import object
from past.utils import old_div
import hashlib
from math import *
import random
seed = 0 # This seed results in successful results for both numpy and python's rng
random.seed(seed)
# Note, the following import is not required, but you may uncomment it
# if you want to use numpy as part of your solution
# make sure to use the provided seed as well, this seed has been tested
# and allows a correct solution to pass the provided test cases:
# import numpy as np
# np.random.seed(seed)
# STUDENT ID
# Please specify your GT login ID in the whoami variable (ex: jsmith123).
whoami = 'jashmore6'
# QUESTION 1
# What is the probability that zero particles are in state A?
q1_n_1 = 0.75
q1_n_4 = .75 **4
q1_n_10 = .75 ** 10
# QUESTION 2
q2_1_step_A = 3
q2_1_step_B = 3
q2_1_step_C = 3
q2_1_step_D = 3
q2_infinite_step_A = 3
q2_infinite_step_B = 3
q2_infinite_step_C = 3
q2_infinite_step_D = 3
# QUESTION 3
# Put 0 for unchecked, 1 for checked
q3_works_fine = 0
q3_ignores_robot_measurements = 1
q3_ignores_robot_motion = 0
q3_it_likely_fails = 1
q3_none_of_above = 0
# QUESTION 4
def q4_move(self, motion):
# You can replace the INSIDE of this function with the move function you modified in the Udacity quiz
steering_angle = motion[0]
distance = motion[1]
beta = distance/self.length * tan(steering_angle)
if beta > 0.001:
R = distance/beta
cx = self.x - sin(self.orientation) * R
cy = self.y + cos(self.orientation) * R
self.x = cx + sin(self.orientation + beta) * R
self.y = cy - cos(self.orientation + beta) * R
else:
self.x = self.x + distance * cos(self.orientation)
self.y = self.y + distance * sin(self.orientation)
self.orientation = (self.orientation + beta ) % (2 * pi)
result = self
return result # make sure your move function returns an instance
# of the robot class with the correct coordinates..
# QUESTION 5
def q5_sense(self, add_noise):
# You can replace the INSIDE of this function with the sense function you modified in the Udacity quiz
# You can ignore add_noise for Q5
Z = []
for cords in landmarks:
ly = cords[0]
lx = cords[1]
Z.append((atan2(ly - self.y, lx-self.x) - self.orientation) % (2 * pi))
return Z #Leave this line here. Return vector Z of 4 bearings.
# QUESTION 6
def q6_move(self, motion):
# You can replace the INSIDE of this function with the move and sense functions
you modified in the Udacity quiz
# Note that there is no motion noise parameter necessary
rob = robot()
rob.length = self.length
rob.bearing_noise = self.bearing_noise
rob.steering_noise = self.steering_noise
rob.distance_noise = self.distance_noise
steering_angle = motion[0]
steering_angle = random.gauss(steering_angle, self.steering_noise)
distance = motion[1]
distance = random.gauss(distance, self.distance_noise)
beta = distance/rob.length * tan(steering_angle)
if beta > 0.001:
R = distance/beta
cx = self.x - sin(self.orientation) * R
cy = self.y + cos(self.orientation) * R
#rob.orientation = (self.orientation + beta ) % (2 * pi)
rob.x = cx + sin(self.orientation + beta) * R
rob.y = cy - cos(self.orientation + beta) * R
else:
rob.x = self.x + distance * cos(self.orientation)
rob.y = self.y + distance * sin(self.orientation)
rob.orientation = (self.orientation + beta ) % (2 * pi)
result = rob
return result
def q6_sense(self, add_noise=1):
# You can replace the INSIDE of this function with what you changed in the Udacity quiz
# Note the add_noise parameter is passed to sense()
Z = []
# ENTER CODE HERE
# HINT: You will probably need to use the function atan2()
for cords in landmarks:
ly = cords[0]
lx = cords[1]
bearing = atan2(ly - self.y, lx-self.x) - self.orientation
if add_noise == 1:
bearing = bearing + random.gauss(0, self.bearing_noise)
bearing = bearing % (2 * pi)
Z.append(bearing)
return Z
######################################################################
# Grading methods
#
# Do not modify code below this point.
#
# The auto-grader does not use any of the below code
# ######################################################################
landmarks = [[0.0, 100.0], [0.0, 0.0], [100.0, 0.0], [100.0, 100.0]]
world_size = 100.0
max_steering_angle = old_div(pi, 4) # You do not need to use this value, but keep
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
in mind the limitations of a real car.
bearing_noise = 0.1 # Noise parameter: should be included in sense function.
steering_noise = 0.1 # Noise parameter: should be included in move function.
distance_noise = 5.0 # Noise parameter: should be included in move function.
tolerance_xy = 15.0 # Tolerance for localization in the x and y directions.
tolerance_orientation = 0.25 # Tolerance for orientation.
class robot(object):
move_func_name = None # used to control which move function is used by robot instance
sense_func_name = None # used to control which sense function is used by robot
instance
def __init__(self, length=10.0):
self.x = random.random() * world_size # initial x position
self.y = random.random() * world_size # initial y position
self.orientation = random.random() * 2.0 * pi # initial orientation
self.length = length # length of robot
self.bearing_noise = 0.0 # initialize bearing noise to zero
self.steering_noise = 0.0 # initialize steering noise to zero
self.distance_noise = 0.0 # initialize distance noise to zero
self.move_func = globals().get(robot.move_func_name)
self.sense_func = globals().get(robot.sense_func_name)
def __repr__(self):
return '[x=%.6s y=%.6s orient=%.6s]' % (str(self.x), str(self.y), str(self.orientation))
def set(self, new_x, new_y, new_orientation):
if new_orientation < 0 or new_orientation >= 2 * pi:
raise ValueError('Orientation must be in [0..2pi]')
self.x = float(new_x)
self.y = float(new_y)
self.orientation = float(new_orientation)
def set_noise(self, new_b_noise, new_s_noise, new_d_noise):
self.bearing_noise = float(new_b_noise)
self.steering_noise = float(new_s_noise)
self.distance_noise = float(new_d_noise)
def measurement_prob(self, measurements):
predicted_measurements = self.sense(0)
if not isinstance(predicted_measurements, list) and not isinstance(predicted_measurements, tuple):
raise RuntimeError( 'sense() expected to return a list, instead got %s'
% type(predicted_measurements) )
if len(predicted_measurements) != len(measurements):
raise RuntimeError( '%d measurements but %d predicted measurements from
sense'
% (len(measurements), len(predicted_measurements)) )
error = 1.0
for i in range(len(measurements)):
error_bearing = abs(measurements[i] - predicted_measurements[i])
error_bearing = (error_bearing + pi) % (2.0 * pi) - pi
error *= (old_div(exp(old_div(- (error_bearing ** 2), (self.bearing_noise ** 2)) / 2.0),
sqrt(2.0 * pi * (self.bearing_noise ** 2))))
return error
def move(self, motion):
if self.move_func is not None:
return self.move_func(self, motion)
else:
return self
def sense(self, add_noise=1):
if self.sense_func is not None:
return self.sense_func(self, add_noise)
else:
return []
def get_position(p):
x = 0.0
y = 0.0
orientation = 0.0
for i in range(len(p)):
x += p[i].x
y += p[i].y
orientation += (((p[i].orientation - p[0].orientation + pi) % (2.0 * pi)) + p[0].orientation - pi)
return [old_div(x, len(p)), old_div(y, len(p)), old_div(orientation, len(p))]
def float_to_str(x): return "%.04f" % x
def do_nothing(x): return x
FILL_IN_TEST_CASES = ({'variable_name': 'q1_n_1',
'str_func': float_to_str,
'answer_hash': '42f2d990b0d1b069fcc6952dcf8e03f5',
'points_avail': 1./3.},
{'variable_name': 'q1_n_4',
'str_func': float_to_str,
'answer_hash': '13786b0afa92c4cfa9bb390dcda4eeaf',
'points_avail': 1./3.},
{'variable_name': 'q1_n_10',
'str_func': float_to_str,
'answer_hash': '162d0da51e5b91c22b8ef816a33b93ae',
'points_avail': 1./3.},
{'variable_name': 'q2_1_step_A',
'str_func': float_to_str,
'answer_hash': '4c519e55122fb9103f3429d4d35246c2',
'points_avail': 1./8.},
{'variable_name': 'q2_1_step_B',
'str_func': float_to_str,
'answer_hash': '865f680967fee7bab877431d55a667e9',
'points_avail': 1./8.},
{'variable_name': 'q2_1_step_C',
'str_func': float_to_str,
'answer_hash': '48482b0046ab77768ab1471544d39c03',
'points_avail': 1./8.},
{'variable_name': 'q2_1_step_D',
'str_func': float_to_str,
'answer_hash': 'b67dd96cc694c9beac33d9fbd459174c',
'points_avail': 1./8.},
{'variable_name': 'q2_infinite_step_A',
'str_func': float_to_str,
'answer_hash': 'eff0c2c81ea4c663110ccca7da9783c9',
'points_avail': 1./8.},
{'variable_name': 'q2_infinite_step_B',
'str_func': float_to_str,
'answer_hash': '3e74f80dcde13f3e2d4016ccfdb3e6fd',
'points_avail': 1./8.},
{'variable_name': 'q2_infinite_step_C',
'str_func': float_to_str,
'answer_hash': '32e9394cabcb64eb6fe926fd18f7eea1',
'points_avail': 1./8.},
{'variable_name': 'q2_infinite_step_D',
'str_func': float_to_str,
'answer_hash': 'b6a07a19e607d0686dfc627c139c373f',
'points_avail': 1./8.},
{'variable_name': 'q3_works_fine',
'str_func': float_to_str,
'answer_hash': 'ab5378bc6072dac87d8eae185c1d5a50',
'points_avail': 1./5.},
{'variable_name': 'q3_ignores_robot_measurements',
'str_func': float_to_str,
'answer_hash': '85f15f9ddfad3555ce7f803375591fcb',
'points_avail': 1./5.},
{'variable_name': 'q3_ignores_robot_motion',
'str_func': float_to_str,
'answer_hash': 'a16592a0343fcca9b90b5220ac53491b',
'points_avail': 1./5.},
{'variable_name': 'q3_it_likely_fails',
'str_func': float_to_str,
'answer_hash': '945f27265e22c511bb56f1631610442a',
'points_avail': 1./5.},
{'variable_name': 'q3_none_of_above',
'str_func': float_to_str,
'answer_hash': 'c885bdcdfbd4a9f353ee68f7be7dbf9e',
'points_avail': 1./5.}, )
# PROGRAMMING
def grader_q4(length=20,
x = 0.0,
y = 0.0,
orientation = 0.0,
bearing_noise = 0.0,
steering_noise = 0.0,
distance_noise = 0.0,
motions = ()):
robot.move_func_name = 'q4_move'
outputs = []
grader_robot = robot(length)
grader_robot.set(x, y, orientation)
grader_robot.set_noise(bearing_noise, steering_noise, distance_noise)
for motion in motions:
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
grader_robot = grader_robot.move(motion)
outputs.append((grader_robot.x, grader_robot.y, grader_robot.orientation))
return outputs
def grader_q5(length=20,
x = 0.0,
y = 0.0,
orientation = 0.0,
bearing_noise = 0.0,
steering_noise = 0.0,
distance_noise = 0.0):
robot.sense_func_name = 'q5_sense'
grader_robot = robot(length)
grader_robot.set(x, y, orientation)
grader_robot.set_noise(bearing_noise, steering_noise, distance_noise)
return grader_robot.sense()
def grader_q6(length=20,
bearing_noise = 0.0,
steering_noise = 0.0,
distance_noise = 0.0,
motions=(),
measurements=(),
N=100):
robot.move_func_name = 'q6_move'
robot.sense_func_name = 'q6_sense'
result = ''
points_earned = 0
p = []
for i in range(N):
r = robot(length=length)
r.set_noise(bearing_noise, steering_noise, distance_noise)
p.append(r)
for t in range(len(motions)):
p2 = []
for i in range(N):
p2.append(p[i].move(motions[t]))
p = p2
w = []
for i in range(N):
w.append(p[i].measurement_prob(measurements[t]))
p3 = []
index = int(random.random() * N) % N
beta = 0.0
mw = max(w)
for i in range(N):
beta += random.random() * 2.0 * mw
while beta > w[index]:
beta -= w[index]
index = (index + 1) % N
p3.append(p[index])
p = p3
return get_position(p)
def q4_output_match(values, expected):
temp = True
if len(values) != len(expected):
return False
else:
for t in range(len(values)):
if not (abs(values[t][0] - expected[t][0]) < 0.01 and abs(values[t][1] - expected[t][1]) < 0.01 and abs(values[t][2] - expected[t][2]) < 0.01):
temp = False
break
return temp
def q5_output_match(values, expected):
temp = True
if len(values) != len(expected):
return False
else:
for t in range(len(values)):
if not (abs(values[t] - expected[t]) < 0.01):
temp = False
break
return temp
def q6_check_output(pos0, pos1):
error_x = abs(pos0[0] - pos1[0])
error_y = abs(pos0[1] - pos1[1])
error_orientation = abs(pos0[2] - pos1[2])
error_orientation = (error_orientation + pi) % (2.0 * pi) - pi
correct = error_x < tolerance_xy and error_y < tolerance_xy \
and error_orientation < tolerance_orientation
return correct
CODE_TEST_CASES = ({'function_name': 'grader_q4',
'function_input': dict(length=20.,
x=0.0,
y=0.0,
orientation=0.0,
bearing_noise=0.0,
steering_noise=0.0,
distance_noise=0.0,
motions=[[0.0, 10.0], [pi / 6.0, 10], [0.0, 20.0]]),
'expected_output': [(10.0, 0.0, 0.0), (19.861, 1.4333, 0.2886),
(39.034, 7.127, 0.2886)],
'outputs_match_func': lambda actual, expected: q4_output_match(actual, expected),
'output_to_str_func': do_nothing},
{'function_name': 'grader_q5',
'function_input': dict(length=20.,
x=30.0,
y=20.0,
orientation=0.0,
bearing_noise=0.0,
steering_noise=0.0,
distance_noise=0.0),
'expected_output': [6.004885648174475, 3.7295952571373605, 1.9295669970654687, 0.8519663271732721],
'outputs_match_func': lambda actual, expected: q5_output_match(actual, expected),
'output_to_str_func': do_nothing},
{'function_name': 'grader_q6',
'function_input': dict(length=20.,
bearing_noise=0.1,
steering_noise=0.1,
distance_noise=5.0,
motions=[[old_div(2. * pi, 10), 20.] for
row in range(8)],
measurements=[
[4.746936, 3.859782, 3.045217, 2.045506],
[3.510067, 2.916300, 2.146394, 1.598332],
[2.972469, 2.407489, 1.588474, 1.611094],
[1.906178, 1.193329, 0.619356, 0.807930],
[1.352825, 0.662233, 0.144927, 0.799090],
[0.856150, 0.214590, 5.651497, 1.062401],
[0.194460, 5.660382, 4.761072, 2.471682],
[5.717342, 4.736780, 3.909599, 2.342536]
]),
'expected_output': [93.476, 75.186, 5.2664],
'outputs_match_func': q6_check_output,
'output_to_str_func': do_nothing,
'tries': 20,
'matches_required': 12 },
)
if __name__ == '__main__':
import checkutil
checkutil.check( FILL_IN_TEST_CASES, CODE_TEST_CASES, locals() )
Your preview ends here
Eager to read complete document? Join bartleby learn and gain access to the full version
- Access to all documents
- Unlimited textbook solutions
- 24/7 expert homework help
Related Documents
Related Questions
I need answers to questions 1, 2, and 3 pertaining to the print provided.
Note: A tutor keeps putting 1 question into 3 parts and wasted so many of my questions. Never had a issue before until now, please allow a different tutor to answer because I was told I am allowed 3 of these questions.
arrow_forward
I need answers to questions 4, 5, and 6 pertaining to the print provided.
Note: A tutor keeps putting 1 question into 3 parts and wasted so many of my questions. Never had a issue before until now, please allow a different tutor to answer because I was told I am allowed 3 of these questions.
arrow_forward
A submarine robot explorer is used to explore the marine life in deep water. The weakest point in the submarine robot explorer structure can hold a pressure force of 300 MPa. What is the deepest point can it reach? *
arrow_forward
question in the picture
arrow_forward
rod-fleet02-xythos.content.blackboardcdn.com/5e0164771d151/9050980?X-Blackboard-Expiration=1645941600-
| D Page view A Read aloud |O Add text Draw
Name:
Banner ID:
1)
In Gotham City, the departments of Administration (A), Health (H), and Transportation (T) are
interdependent. For every dollar's worth of services, they produce, each department uses a certain
amount of the services produced by the other departments and itself, as shown in Table 1. Suppose
that, during the
in Health services, and $0.8 million in Transportation services. What does the annual dollar value of
the services produced by each department need to be in order to meet the demands?
year,
other city departments require S1 million in Administrative services, $1.2 million
Table 1
Department
A
H.
T
$0.20
0.10
0.20
0.10
0.10
0.20
Buy
H.
0.40
0.30
1.
0.20
arrow_forward
Don't copy paste someone else answer if I get to know I'll report and downvote too do on your own and only handwritten with proper steps not that handwritten only
arrow_forward
please show all the work with diagrams
arrow_forward
I cannot read the answer. Is there a way to upload it in another format?
arrow_forward
\ח
"Chicago - Lakeshore Dr from John Hancock Center" by Ryan from Toronto, CA CC BY 2.0
This image is taken from the John Hancock Center and is an aerial view near Oak Street Beach. The
general speed limit on Jean Baptiste Point DuSable Lake Shore Drive, shown in this image, is 40
mph. As you travel south on the drive, you must enter the curve south of Oak Street Beach (at Point
..AL
-far
BIKI
O Search
Hintain
11
3
SIM
arrow_forward
need some help on this please
arrow_forward
AutoSave
STATICS - Protected View• Saved to this PC -
O Search (Alt+Q)
Off
ERIKA JOY DAILEG
EJ
File
Home
Insert
Draw
Design
Layout
References
Mailings
Review
View
Help
Acrobat
O Comments
E Share
PROTECTED VIEW Be careful-files from the Internet can contain viruses. Unless you need to edit, it's safer to stay in Protected View.
Enable Editing
Situation 9 - A 6-m long ladder weighing 600 N is shown in the Figure. It is required to determine
the horizontal for P that must be exerted at point C to prevent the ladder from sliding. The
coefficient of friction between the ladder and the surface at A and B is 0.20.
25. Determine the reaction at A.
26. Determine the reaction at B.
27. Determine the required force P.
4.5 m
1.5 m
H=0.2
30°
Page 5 of 5
671 words
D. Focus
100%
C
ЕPIC
GAMES
ENG
7:24 pm
w
US
16/02/2022
IZ
arrow_forward
A Team of Engineers asked for an internal combustion engine to use it in a designed car. Your role is to describe the operation sequence of different types of available engines, explain their mechanical efficiency, and deliver a detailed technical report to show your approach in solving and discussing the following tasks and issues.
You must follow the following steps to help the team:
STEP 1
Describe the operational sequence of four-stroke spark ignition and four-stroke compression ignition engines with the aid of sketches by constructing simple sketch representing the operation and plotting the P-V diagrams for each process during the cycle to show the following:
The input and output heat and net output work
The expansion and compression strokes
The air-fuel mixture intake and exhaust gasses
The spark plug when it is in the active mode
The complete cycle of ideal Otto and Diesel cycles that shows the input and output heat and net output work.
STEP 2
Explain the mechanical…
arrow_forward
label the oarts of otto enhine
arrow_forward
Use the following information to answer the next three questions
Mobile power plants, such as the one illustrated below, are used in remote locations.
Flowing water turns a turbine, which then moves a coil of wire through a magnetic
field.
Mobile Power Plant
Moving water
Stream
flowing downhill
Location I
Water turning turbine
Location II
-Spinning turbine
-Permanent magnet
Rotating wire coil
Split-ring
commutator
Metal brush
10. The components that connect to the spinning turbine in the illustration on the
previous page form a
O ammeter
O generator
O motor
1.
O transformer
Your reasoning:
MacBook Pro
arrow_forward
The answer from Bartleby is missing/blank. Can someone help answer it please and credit this question if possible since it would have been included/explained?
Thank you
Chapter 50 multiple Choice #5 what is the cause of an extremely hard brake pedal
arrow_forward
Can anyone help me with these questions please
arrow_forward
Chrome
File
Edit
View
History
Bookmarks
People
Tab
Window
Help
McGraw-Hill Campus - ALEKS Science - CHM1045 GEN CHEM 1 BLENDED 669113
A bconline.broward.edu/d21/le/content/466883/fullscreen/12868783/View
McGraw-Hill Campus - ALEKS Science
O GASES
Interconverting pressure and force
A chemistry graduate student is designing a pressure vessel for an experiment. The vessel will contain gases at pressures up to 470.0 MPa. The student's
design calls for an observation port on the side of the vessel (see diagram below). The bolts that hold the cover of this port onto the vessel can safely withstand
a force of 2.80 MN.
pressure vessel
bolts
side
View
port
Calculate the maximum safe diameter w of the port. Round your answer to the nearest 0.1 cm.
O cm
Explanation
Check
O2021 McGraw-Hill Education. All Rights Reserved. Terms of Use
FEB
arrow_forward
arrow_forward
Oh no! Our expert couldn't answer your question.
Don't worry! We won't leave you hanging. Plus, we're giving you back one question for the inconvenience.
Here's what the expert had to say:
Hi and thanks for your question! Unfortunately we cannot answer this particular question due to its complexity. We've credited a question back to your account. Apologies for the inconvenience.
Ask Your Question Again
5 of 10 questions left
until 8/10/20
Question
Asked Jul 13, 2020
1 views
An air conditioning unit uses Freon (R-22) to adapt an office room at temperature 25 oC in the summer, if the temperature of the evaporator is 16 oC and of the condenser is 48 oC. The reciprocating compressor is single acting, number of cylinders are 2, the volumetric efficiency is 0.9, number of revolutions are 900 r.p.m. and L\D= 1.25. If the compressor consumes a power of 3 kW and its mechanical efficiency is 0.9. Find the following:
(A) Flow rate of the refrigerant per…
arrow_forward
SEE MORE QUESTIONS
Recommended textbooks for you
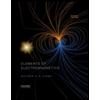
Elements Of Electromagnetics
Mechanical Engineering
ISBN:9780190698614
Author:Sadiku, Matthew N. O.
Publisher:Oxford University Press
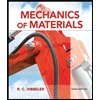
Mechanics of Materials (10th Edition)
Mechanical Engineering
ISBN:9780134319650
Author:Russell C. Hibbeler
Publisher:PEARSON
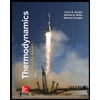
Thermodynamics: An Engineering Approach
Mechanical Engineering
ISBN:9781259822674
Author:Yunus A. Cengel Dr., Michael A. Boles
Publisher:McGraw-Hill Education
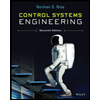
Control Systems Engineering
Mechanical Engineering
ISBN:9781118170519
Author:Norman S. Nise
Publisher:WILEY
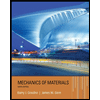
Mechanics of Materials (MindTap Course List)
Mechanical Engineering
ISBN:9781337093347
Author:Barry J. Goodno, James M. Gere
Publisher:Cengage Learning
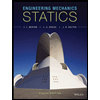
Engineering Mechanics: Statics
Mechanical Engineering
ISBN:9781118807330
Author:James L. Meriam, L. G. Kraige, J. N. Bolton
Publisher:WILEY
Related Questions
- I need answers to questions 1, 2, and 3 pertaining to the print provided. Note: A tutor keeps putting 1 question into 3 parts and wasted so many of my questions. Never had a issue before until now, please allow a different tutor to answer because I was told I am allowed 3 of these questions.arrow_forwardI need answers to questions 4, 5, and 6 pertaining to the print provided. Note: A tutor keeps putting 1 question into 3 parts and wasted so many of my questions. Never had a issue before until now, please allow a different tutor to answer because I was told I am allowed 3 of these questions.arrow_forwardA submarine robot explorer is used to explore the marine life in deep water. The weakest point in the submarine robot explorer structure can hold a pressure force of 300 MPa. What is the deepest point can it reach? *arrow_forward
- question in the picturearrow_forwardrod-fleet02-xythos.content.blackboardcdn.com/5e0164771d151/9050980?X-Blackboard-Expiration=1645941600- | D Page view A Read aloud |O Add text Draw Name: Banner ID: 1) In Gotham City, the departments of Administration (A), Health (H), and Transportation (T) are interdependent. For every dollar's worth of services, they produce, each department uses a certain amount of the services produced by the other departments and itself, as shown in Table 1. Suppose that, during the in Health services, and $0.8 million in Transportation services. What does the annual dollar value of the services produced by each department need to be in order to meet the demands? year, other city departments require S1 million in Administrative services, $1.2 million Table 1 Department A H. T $0.20 0.10 0.20 0.10 0.10 0.20 Buy H. 0.40 0.30 1. 0.20arrow_forwardDon't copy paste someone else answer if I get to know I'll report and downvote too do on your own and only handwritten with proper steps not that handwritten onlyarrow_forward
- please show all the work with diagramsarrow_forwardI cannot read the answer. Is there a way to upload it in another format?arrow_forward\ח "Chicago - Lakeshore Dr from John Hancock Center" by Ryan from Toronto, CA CC BY 2.0 This image is taken from the John Hancock Center and is an aerial view near Oak Street Beach. The general speed limit on Jean Baptiste Point DuSable Lake Shore Drive, shown in this image, is 40 mph. As you travel south on the drive, you must enter the curve south of Oak Street Beach (at Point ..AL -far BIKI O Search Hintain 11 3 SIMarrow_forward
- need some help on this pleasearrow_forwardAutoSave STATICS - Protected View• Saved to this PC - O Search (Alt+Q) Off ERIKA JOY DAILEG EJ File Home Insert Draw Design Layout References Mailings Review View Help Acrobat O Comments E Share PROTECTED VIEW Be careful-files from the Internet can contain viruses. Unless you need to edit, it's safer to stay in Protected View. Enable Editing Situation 9 - A 6-m long ladder weighing 600 N is shown in the Figure. It is required to determine the horizontal for P that must be exerted at point C to prevent the ladder from sliding. The coefficient of friction between the ladder and the surface at A and B is 0.20. 25. Determine the reaction at A. 26. Determine the reaction at B. 27. Determine the required force P. 4.5 m 1.5 m H=0.2 30° Page 5 of 5 671 words D. Focus 100% C ЕPIC GAMES ENG 7:24 pm w US 16/02/2022 IZarrow_forwardA Team of Engineers asked for an internal combustion engine to use it in a designed car. Your role is to describe the operation sequence of different types of available engines, explain their mechanical efficiency, and deliver a detailed technical report to show your approach in solving and discussing the following tasks and issues. You must follow the following steps to help the team: STEP 1 Describe the operational sequence of four-stroke spark ignition and four-stroke compression ignition engines with the aid of sketches by constructing simple sketch representing the operation and plotting the P-V diagrams for each process during the cycle to show the following: The input and output heat and net output work The expansion and compression strokes The air-fuel mixture intake and exhaust gasses The spark plug when it is in the active mode The complete cycle of ideal Otto and Diesel cycles that shows the input and output heat and net output work. STEP 2 Explain the mechanical…arrow_forward
arrow_back_ios
SEE MORE QUESTIONS
arrow_forward_ios
Recommended textbooks for you
- Elements Of ElectromagneticsMechanical EngineeringISBN:9780190698614Author:Sadiku, Matthew N. O.Publisher:Oxford University PressMechanics of Materials (10th Edition)Mechanical EngineeringISBN:9780134319650Author:Russell C. HibbelerPublisher:PEARSONThermodynamics: An Engineering ApproachMechanical EngineeringISBN:9781259822674Author:Yunus A. Cengel Dr., Michael A. BolesPublisher:McGraw-Hill Education
- Control Systems EngineeringMechanical EngineeringISBN:9781118170519Author:Norman S. NisePublisher:WILEYMechanics of Materials (MindTap Course List)Mechanical EngineeringISBN:9781337093347Author:Barry J. Goodno, James M. GerePublisher:Cengage LearningEngineering Mechanics: StaticsMechanical EngineeringISBN:9781118807330Author:James L. Meriam, L. G. Kraige, J. N. BoltonPublisher:WILEY
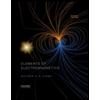
Elements Of Electromagnetics
Mechanical Engineering
ISBN:9780190698614
Author:Sadiku, Matthew N. O.
Publisher:Oxford University Press
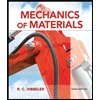
Mechanics of Materials (10th Edition)
Mechanical Engineering
ISBN:9780134319650
Author:Russell C. Hibbeler
Publisher:PEARSON
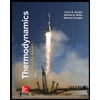
Thermodynamics: An Engineering Approach
Mechanical Engineering
ISBN:9781259822674
Author:Yunus A. Cengel Dr., Michael A. Boles
Publisher:McGraw-Hill Education
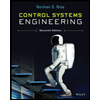
Control Systems Engineering
Mechanical Engineering
ISBN:9781118170519
Author:Norman S. Nise
Publisher:WILEY
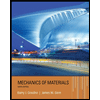
Mechanics of Materials (MindTap Course List)
Mechanical Engineering
ISBN:9781337093347
Author:Barry J. Goodno, James M. Gere
Publisher:Cengage Learning
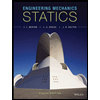
Engineering Mechanics: Statics
Mechanical Engineering
ISBN:9781118807330
Author:James L. Meriam, L. G. Kraige, J. N. Bolton
Publisher:WILEY